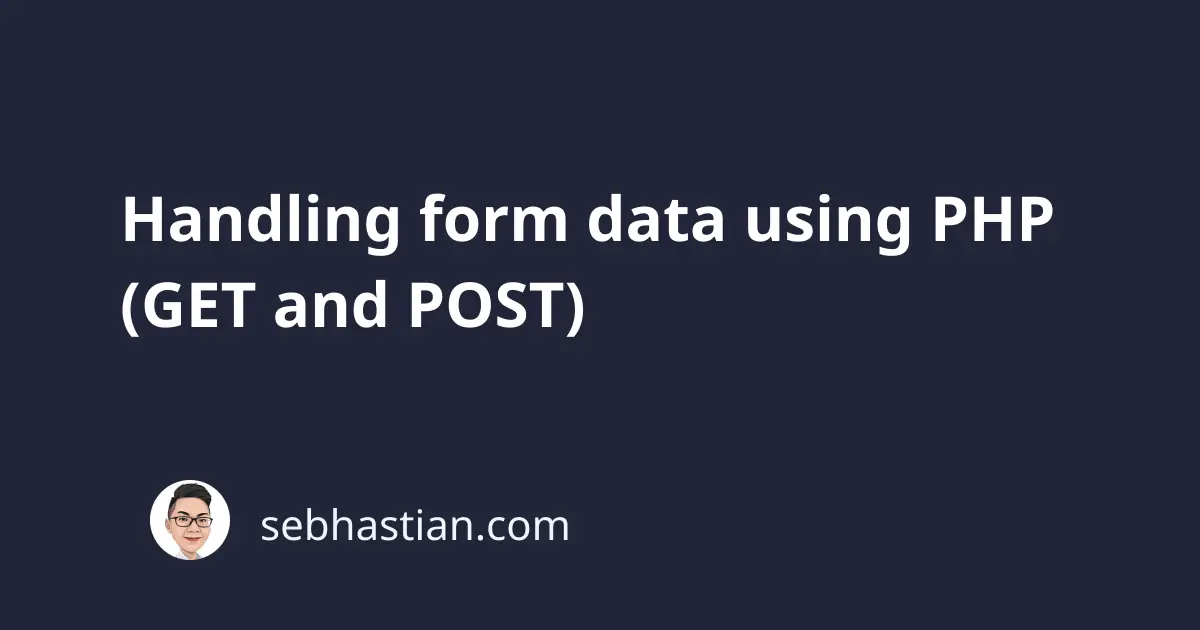
PHP is able to handle form data submitted using HTML forms.
The PHP superglobals $_GET
and $_POST
are used to handle form data using GET and POST HTTP request methods.
Let’s see an example of how to accept user data using a form in PHP.
Handling forms using the POST method
First, let’s start by creating a simple HTML form. This form will have a single text input field and a submit button:
<form action="submit.php" method="post">
<label for="name">Name:</label>
<input type="text" name="name" />
<input type="submit" value="Submit" />
</form>
The action
attribute specifies the URL where the form data will be sent when the form is submitted.
The method
attribute specifies the HTTP request method used to submit the form data. In this case, we’re using the POST method, which is more secure than the GET method.
Next, we’ll create the submit.php
script to handle the form submission. The form data will be sent through the $_POST
superglobal array:
<html>
<body>
Hello, <?php echo $_POST["name"]; ?>
</body>
</html>
The name
attribute of the <input>
element will be used as the key value of the $_POST
array.
The output of the code above will be:
Hello, Nathan
Suppose you have a form with two input fields of name
and email
as shown below:
<form action="submit.php" method="post">
<label for="name">Name:</label>
<input type="text" name="name" /><br/>
<label for="email">Email:</label>
<input type="email" name="email" /><br/>
<input type="submit" value="Submit" />
</form>
Then you can access those data using $_POST
array like this:
$name = $_POST['name'];
$email = $_POST['email'];
Handling forms using the GET method
To handle form data using the GET method, you can use the $_GET
superglobal array in the same way that you would use the $_POST
array for handling form data with the POST method.
In the HTML form, you need to change the method
attribute to get
as follows:
<form action="submit.php" method="get">
<label for="name">Name:</label>
<input type="text" name="name" id="name">
<input type="submit" value="Submit">
</form>
And here’s the code to handle the form submission:
<html>
<body>
Hello, <?php echo $_GET["name"]; ?>
</body>
</html>
One thing to note is that the GET method is less secure than the POST method because the form data is included in the URL and is visible to the user.
The POST method sends the form data in the request body, which is not visible to the user.
This is why it’s generally recommended to use the POST method for handling sensitive data, such as passwords or credit card numbers.
The GET method can be used for non-sensitive input such as a search query. As a matter of fact, when you perform a Google search, you can see what you put into the search bar in the URL.
When you have the data under $_POST
or $_GET
array, you are free to process those data according to your requirements.
Now that you’ve learned the basics of form handling with PHP, you may also want to know how you can validate your form data.
Be sure to check out my Form validation in PHP article.