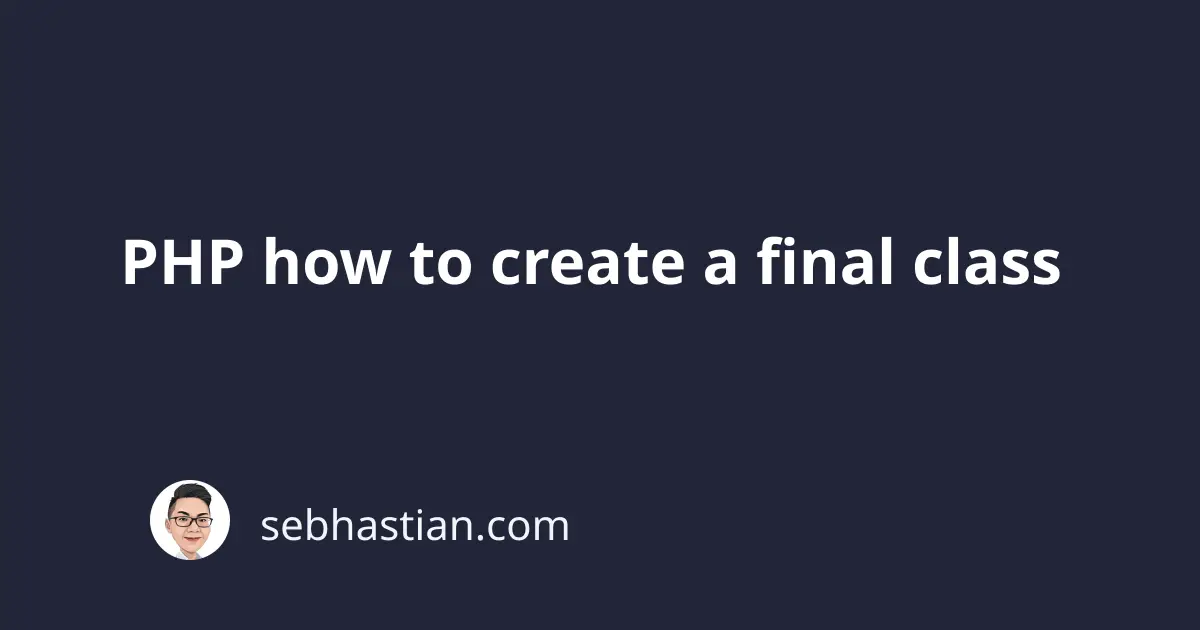
PHP provides you with the final
keyword that you can use as a modifier for classes and functions.
When you add the final
keyword to a class, that class can’t be extended.
Here’s an example of creating a final class:
<?php
final class Car {
function start(){
print "Start the engine";
}
}
PHP will throw an error when you try to extend a final class as shown below:
class Tesla extends Car {}
// Fatal error:
// Class Tesla cannot extend final class Car
The final
keyword is useful to restrict a class
, removing the possibility of extending that class.
You can also put the final
keyword in front of class functions to disable child classes from overriding that function:
<?php
class Car {
final function start(){
print "Start the engine";
}
}
class Tesla extends Car {
// 👇 Cannot override final method Car::start()
function start(){
print "Tesla starting...";
}
}
Now you’ve learned how to create a final class in PHP.