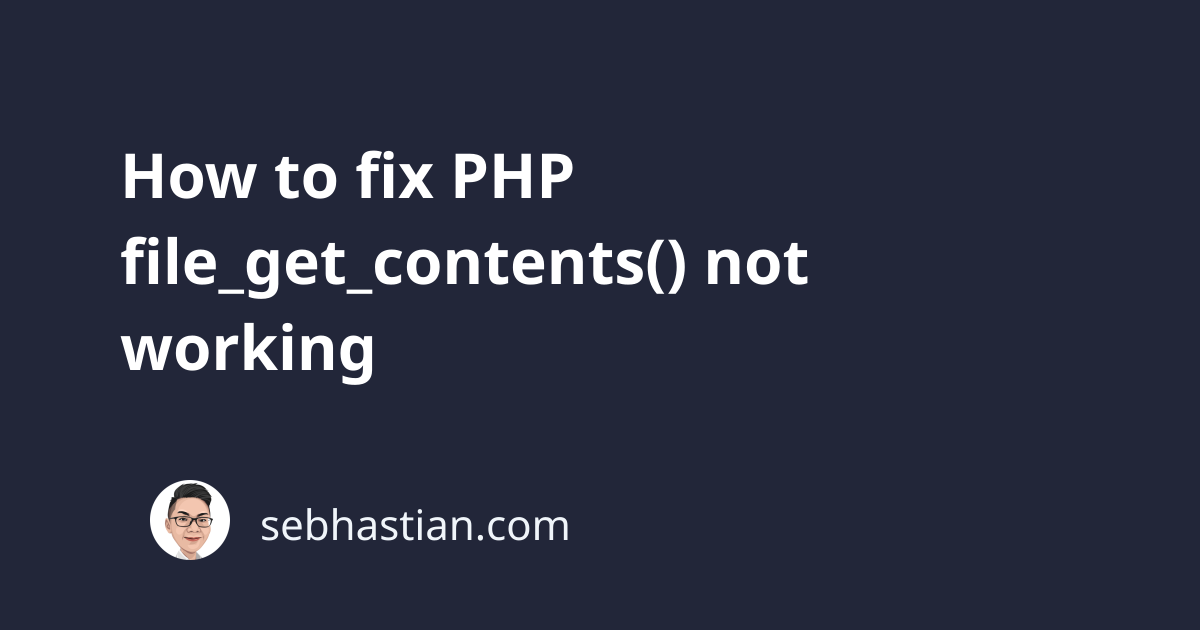
The PHP file_get_contents()
function is used to read a file content and return it as a string.
Most of the time, this function is also used to get the content of a file.
But when you need to read a remote file, the function may not work and give a warning response instead.
For example, the following code:
<?php
$res = file_get_contents("https://google.com");
Produced the following warning:
Warning: file_get_contents(https://google.com):
Failed to open stream: no suitable wrapper could be found
in ... on line ...
To access a remote file using the file_get_contents()
function, you need to activate the allow_url_fopen
configuration.
You need to open the php.ini
file loaded by your PHP server and set the allow_url_fopen
configuration to On
.
If you can’t find where your php.ini
file is located, then run the phpinfo()
function by creating a new file:
<?php
phpinfo();
?>
You need to find the php.ini
location as shown below:
Open the php.ini
file, then activate the allow_url_fopen
config:
allow_url_fopen = On
Restart your Apache or Nginx server, and you should be able to read the content of a remote file.
Using cURL instead of file_get_contents
Sometimes, you may see that you can use file_get_contents()
from a local machine but doesn’t work from a server.
This is because your server may have security protocols that prevent file_get_contents()
from working.
For an alternative solution, you can use the cURL library to read the content of a remote file.
Here is an example of using cURL:
// 1. initialize cURL
$ch = curl_init();
// 2. set the URL to access
$url = "https://google.com";
curl_setopt($ch, CURLOPT_URL, $url);
// 3. set cURL to return as a string
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// 4. execute cURL and store the result
$output = curl_exec($ch);
// 5. close cURL after use
curl_close($ch);
// 6. print the output
print $output;
You can read more about cURL options in curl_setopt documentation.
Unlike the file_get_contents()
function, cURL is designed to allow data transfer from various network protocols.
It’s usually enabled by default, so you don’t need to tweak your PHP configuration.
Whenever possible, use cURL to access remote files and file_get_contents()
function for local files.
Now you’ve learned how to solve file_get_contents()
function not working. You’ve also learned how to replace the function with cURL.