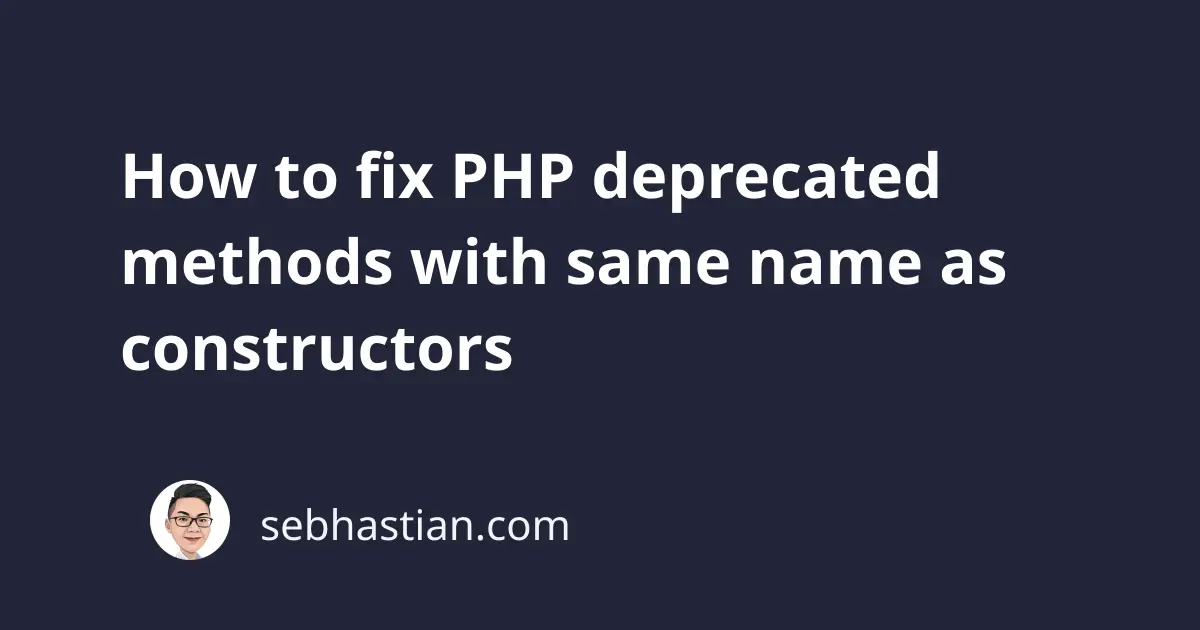
When developing your application in PHP v7, you may get a deprecated message as follows:
PHP Deprecated: Methods with the same name as their class will not be constructors in a future version of PHP; Dog has a deprecated constructor in lib.php on line 2
The deprecated message above happens because you have a class
with an old style constructor.
To resolve the error, replace the constructor function name with __construct
.
Here’s an example:
<?php
class Dog {
public string $name;
public function Dog(string $name) {
$this->name = $name;
}
}
Replace the Dog()
function above as follows:
<?php
class Dog {
public string $name;
public function __construct(string $name) {
$this->name = $name;
}
}
And the deprecated message should disappear when you rerun the PHP script.
Why PHP deprecated methods with same name as constructors?
In PHP v4, constructors were introduced so that you can create a class with a method that automatically gets called whenever an object of that class is instantiated.
In PHP v5, the __construct()
function was released. This causes PHP to have two ways to define a constructor in a class.
In PHP v7, it was decided that the __construct()
function would be the only way to create a constructor in future versions of PHP. This is why you get the message as shown above.
If you still use a method with the same name as the class as the constructor, you will get a fatal error in PHP v8 when creating the class instance:
PHP Fatal error: Uncaught Error: Typed property Dog::$name must not be accessed before initialization in lib.php:14
In PHP v8, the Dog()
function in the Dog
class as the example above won’t have any special meaning. PHP doesn’t consider it as a constructor.
Always use the __construct()
function to create constructors from PHP v7 onward.
And that’s how you fix PHP Deprecated: Methods with the same name as their class will not be constructors in a future version of PHP message.