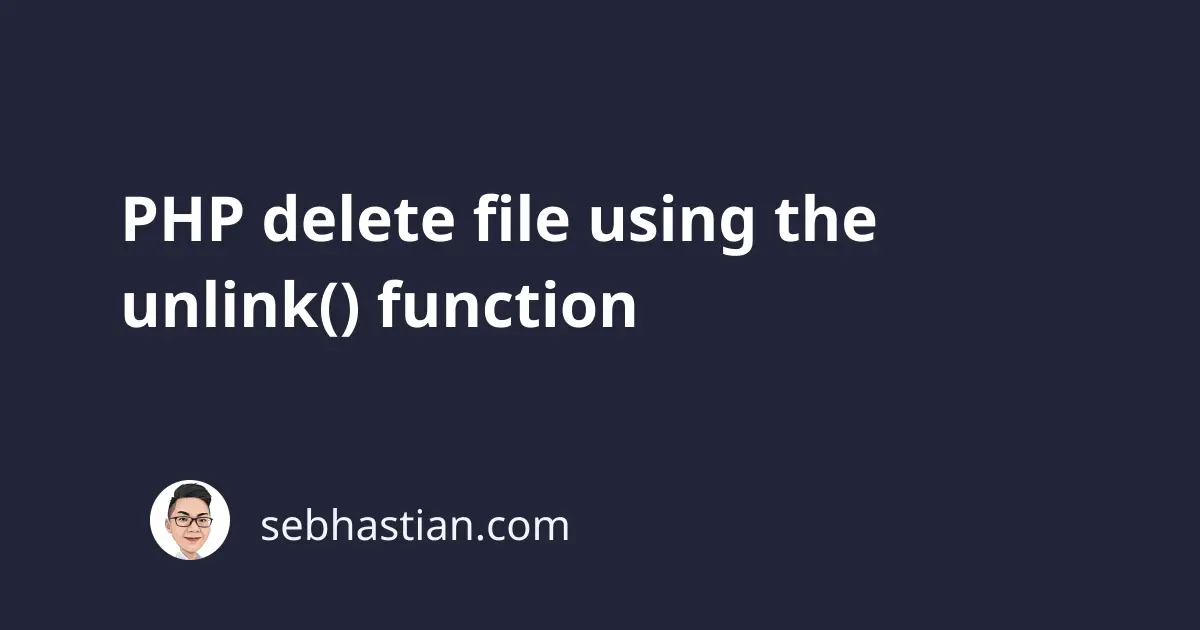
To delete a file you have in your server using PHP, you need to use the unlink()
function.
The unlink()
function accepts a string
argument that represents the path to the file.
For example, suppose you have the following files in your current directory:
.
├── index.php
├── one.txt
├── three.txt
└── two.txt
To delete the file one.txt
from the directory, add the following code to the index.php
file:
<?php
unlink('one.txt');
?>
When you run the index.php
file, then the file one.txt
will be deleted from the directory.
When your file is in another directory, you need to present the file path from the current directory.
Suppose you have the following tree structure for your files:
.
├── assets
│ └── image.jpg
├── index.php
├── three.txt
└── two.txt
To delete the file image.jpg
, pass the file path to the unlink()
function:
<?php
unlink('assets/image.jpg');
?>
When a file is not found for deletion, then PHP will output a warning as shown below:
PHP Warning: unlink(assets/image.jpg):
No such file or directory in /DEV/assets/index.php on line 2
Unlink will not cause an error when it can’t delete the file.
You can also check if the unlink is successful using the bang !
operator:
<?php
if (!unlink('assets/image.jpg')) {
echo "The file can't be deleted";
} else {
echo "The file has been deleted successfully";
}
?>
Next, let’s see how you can delete multiple files using the unlink()
function.
Delete multiple files using array_map
Sometimes, you may want to delete many files that match a certain pattern.
Rather than repeatedly calling the unlink()
function, you can use this tip from anagai that allows you to perform a recursive delete using the unlink()
function:
<?php
array_map('unlink', glob("*.txt"));
?>
The above code will delete all .txt
file in the current working directory where you call the PHP code.
You can also add the path to a certain folder you want to perform the recursive delete on:
<?php
// 👇 delete all jpg files on the assets folder
array_map('unlink', glob("assets/*.jpg"));
?>
And that’s how you remove multiple files with the unlink()
function. Good work!