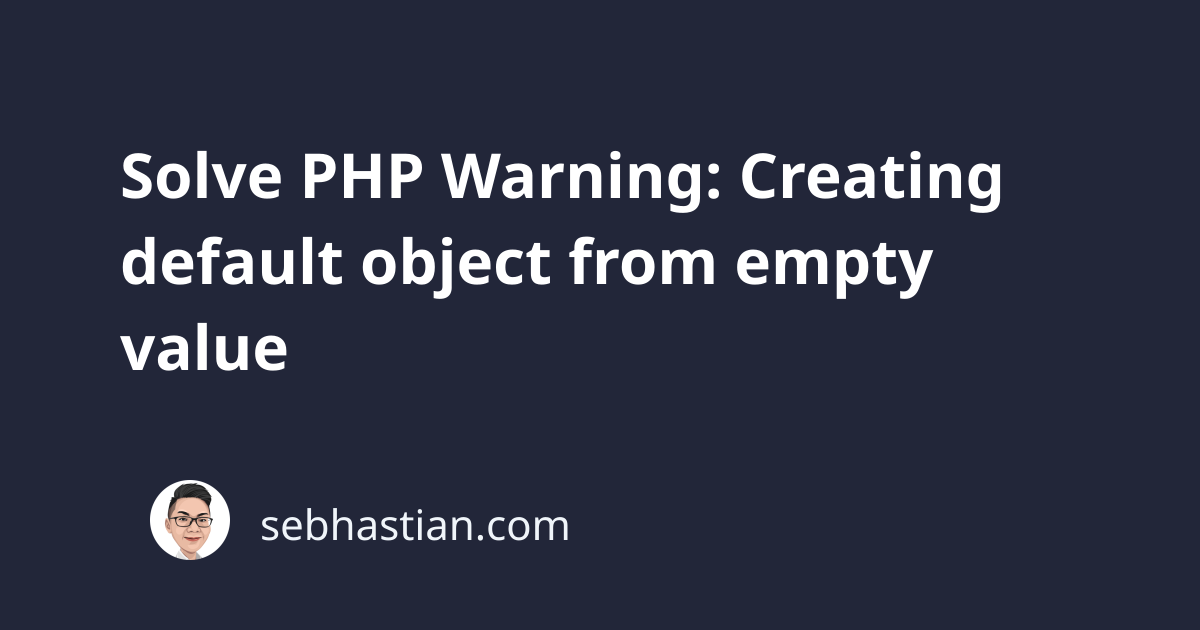
The message PHP Warning: Creating default object from empty value appears when you assign property to an object without initializing the object first.
Here’s an example code that triggers the warning:
<?php
$user->name = "Jack";
In the code above, the $user
object has never been initialized.
When assigning the name
property to the $user
object, the warning message gets shown:
Warning: Creating default object from empty value
To solve this warning, you need to initialize the $user
object before assigning properties to it.
There are three ways you can initialize an object in PHP:
Let’s learn how to do all of them in this tutorial.
PHP initialize object with stdClass
The stdClass
is a generic empty class provided by PHP that you can use for whatever purpose.
One way to initialize an object is to create a new instance of the stdClass()
like this:
<?php
$user = new stdClass();
$user->name = "Jack";
After initializing the $user
variable as an object instance, you can assign any property to that object without triggering the warning.
PHP initialize object by casting an array as an object
You can also cast an empty array as an object like this:
<?php
$user = (object)[];
$user->name = "Jack";
By casting an empty array as an object, you can assign properties to that object.
PHP initialize object by creating a new anonymous class instance
PHP also allows you to create a new anonymous class instance by using the new class
keyword:
<?php
$user = new class {};
$user->name = "Jack";
The anonymous class instance will be an empty object, and you can assign properties to it.
Now you’ve learned how to solve the PHP Warning: Creating default object from empty value. Nice job! 👍