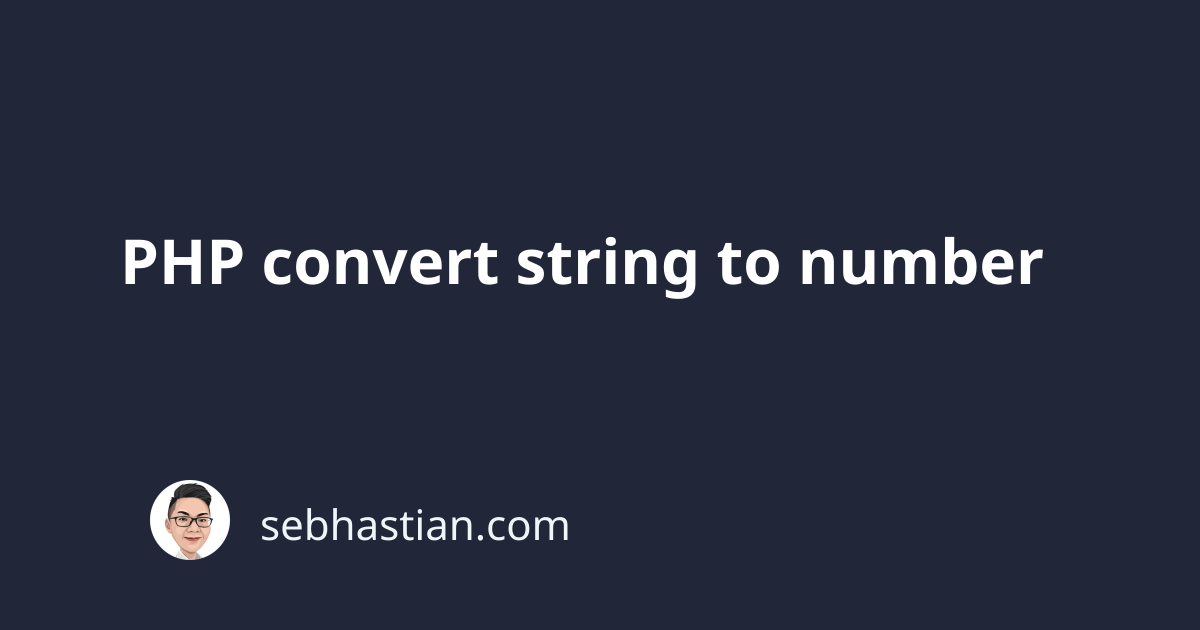
There are three different ways you can convert a string type to a number type in PHP:
Let’s see how to perform each method in this tutorial.
Convert string to number with (int) and (float) casting keyword
You can cast a string
type value into an int
type using the (int)
type casting syntax.
Consider the code examples below:
<?php
echo (int) "7E"; // 7
echo (int) "523.12"; // 523
echo (int) "true"; // 0
echo (int) "3.14"; // 3
echo (int) "abc89"; // 0
The (int)
casting syntax will remove any floating numbers you have in your string.
To keep the floating numbers, you can use the (float)
keyword to cast the string:
<?php
echo (float) "7E"; // 7
echo (float) "523.12"; // 523.12
echo (float) "true"; // 0
echo (float) "3.14"; // 3.14
echo (float) "abc89"; // 0
Convert string to number with intval() and floatval()
The intval()
and floatval()
functions are used for changing a data type to int
and float
respectively.
You need to pass the string data to convert as an argument to the function as follows:
<?php
echo intval("172.9521"); // 172
echo floatval("172.9521"); // 172.9521
echo intval("false"); // 0
echo floatval("false"); // 0
Any string that can be converted to a valid number will be converted with the intval()
and floatval()
functions.
Both functions will return 0
when no valid number can be interpreted from the given argument.
You can view the PHP documentation for intval()
and floatval()
functions for more info.
Convert string to number by adding zero
When you add a number to a string
type that has only numbers, then the string
will be converted implicitly to a number type.
Consider the following example:
<?php
// 👇 convert to double
echo "123.456" + 0; // 1000.314
// 👇 convert to integer
echo "123" + 0; // 123
// 👇 convert to integer but with warning:
echo "34tu" + 0; // 34
But keep in mind that when you have a string
without any number, then PHP will throw a TypeError
exception.
See the examples below:
echo "true" + 0; // Uncaught TypeError
echo "xyz67" + 0; // // Uncaught TypeError
While the implicit conversion works, you are recommended to use either type casting or intval()
function instead.
And now you’ve learned how to convert a string to a number using PHP type casting, intval()
and floatval()
functions.
You’ve also learned how to use PHP implicit conversion to convert a string to a number.