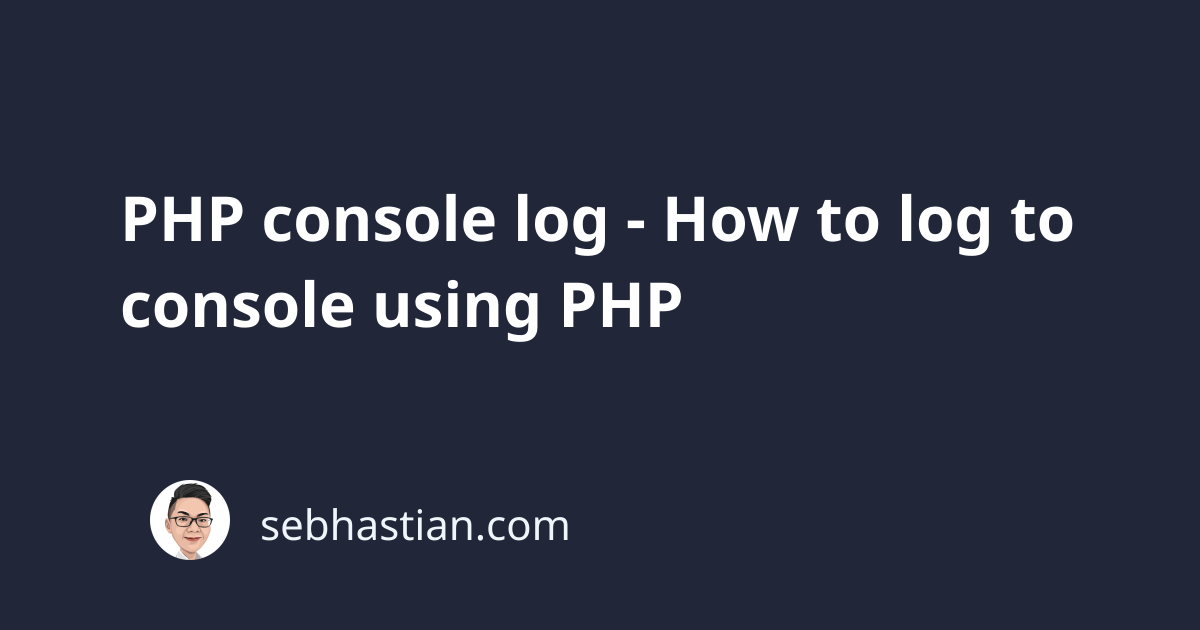
Sometimes, you need to log your PHP variable values to the browser console for debugging purposes.
Unfortunately, the PHP echo
function will render output in the browser’s window or the command line terminal, depending on where the PHP script is executed.
In this tutorial, you will learn how to write a log to the browser console using PHP.
Write a log to the console using PHP
As a server-side scripting language, PHP doesn’t have a built-in function to interact with the browser’s API directly.
To write logs to the browser console, you need the help of JavaScript like this:
<?php
$data = "Nathan";
echo "<script>console.log('{$data}' );</script>";
Running the PHP script below will produce the following HTML output:
<html>
<head>
<script>
console.log("Nathan");
</script>
</head>
<body cz-shortcut-listen="true"></body>
</html>
By adding <script>
tag to the echo
function’s argument, you will generate a <script>
tag in the rendered HTML.
This way, the PHP variable $data
value will be logged to the browser console as shown below:
Because the echo
function outputs a string
, you will receive a warning when you log an array, and a fatal error when you log an object.
To print arrays and objects successfully, call the json_encode()
function to transform the data as follows:
$data = "Nathan";
// 👇 create a JSON string to encode the variable value
$output = json_encode($data);
echo "<script>console.log('{$output}' );</script>";
Now you can print variable values to the console, including an array and an object.
To avoid repeating the code each time you need to log to the console, you can create a reusable function named log_to_console()
as shown below:
function log_to_console($data) {
$output = json_encode($data);
echo "<script>console.log('{$output}' );</script>";
}
Let’s test the log_to_console()
function and see its output:
// 👇 create an array
$users = ["Nathan", "Jane", "John", "Sarah"];
// 👇 create an object
$person = new stdClass();
$person->name = "Nathan";
$person->age = 28;
$person->knowsPHP = true;
log_to_console($users);
log_to_console($person);
The output in the console is as follows:
To keep the array and object format in the console, you need to remove the quotation mark around the console.log()
argument.
You can add a second parameter to the log_to_console()
function that decides whether a quote will be added to the output or not:
function log_to_console($data, bool $quotes = true) {
$output = json_encode($data);
if ($quotes) {
echo "<script>console.log('{$output}' );</script>";
} else {
echo "<script>console.log({$output} );</script>";
}
}
Then, you need to pass a second argument when you call the log_to_console()
function to log arrays and objects:
log_to_console($users, false);
log_to_console($person, false);
log_to_console("Hello World!");
Now the output will be as follows:
That looks much better than the previous output.
PHP console log using the script tag
Depending on your preference, you can also log your PHP values using the <script>
tag in your PHP view files like this:
<?php
$users = ["Nathan", "Jane", "John", "Sarah"]; ?>
<script>
console.log('<?= json_encode($users) ?>');
</script>
The <?= ?>
tag is the shorthand syntax for the echo
function.
If the function above doesn’t work, check the quotation marks you used around your variable and console.log()
function.
If the PHP variable use double quotes, the console.log()
function needs to use the single quote mark, and vice versa.
For arrays and objects, you can omit the quotes inside the console.log()
function so that the browser doesn’t log them as strings.
PHP console log using the PHPDebugConsole library
Besides writing your own function, PHP also has open source libraries to write logs to the console.
Two of the most popular libraries are PHPDebugConsole and PHPConsole. Let’s see how PHPDebugConsole works.
First, you need to install the PHPDebugConsole
library in your project. You can download the release zip or you can use Composer.
Here’s how to install it using Composer:
composer require bdk/debug:3.0b1 --update-no-dev
Note that you need to explicitly use version 3 because version 2 doesn’t generate output to the browser console.
Next, import the library into your code using require
and set the debug configuration as follows:
<?php
require __DIR__ . "/vendor/autoload.php"; // if installed via composer
// require 'path/to/Debug.php'; // if not using composer
$debug = new \bdk\Debug([
"collect" => true,
"output" => true,
"route" => "script",
]);
Now you can log into the browser console as follows:
$debug->alert("PHPDebugConsole Alert");
$debug->warn("This is a warning log");
$debug->info("This is an info log");
$debug->table(
[
["city" => "Atlanta", "state" => "GA", "population" => 472522],
["city" => "Buffalo", "state" => "NY", "population" => 256902],
["city" => "Chicago", "state" => "IL", "population" => 2704958],
["city" => "Denver", "state" => "CO", "population" => 693060],
],
"Table"
);
And here’s the output of the code above:
By default, the PHPDebugConsole library will generate an output to the HTML page.
This is changed by setting the route
option to script
when instantiating the Debug
class as shown above.
The PHPDebugConsole has many options to help you debug PHP code.
It can also be integrated with PHP frameworks like Laravel, Symfony, and Yii, so head over to PHPDebugConsole documentation if you’re interested to try it out.
Conclusion
To generate a log output using PHP, you need to create a custom function that calls the JavaScript console.log()
function.
The code for the function is as follows:
function log_to_console($data, bool $quotes = true) {
$output = json_encode($data);
if ($quotes) {
echo "<script>console.log('{$output}' );</script>";
} else {
echo "<script>console.log({$output} );</script>";
}
}
Alternatively, you can also install the PHPDebugConsole library to your PHP project so that you can generate a more detailed output.
But for simple projects, using PHPDebugConsole may be too much, so you can stick with the log_to_console()
function created above.
Now you’ve learned how to log to the console using PHP. Good work! 👍