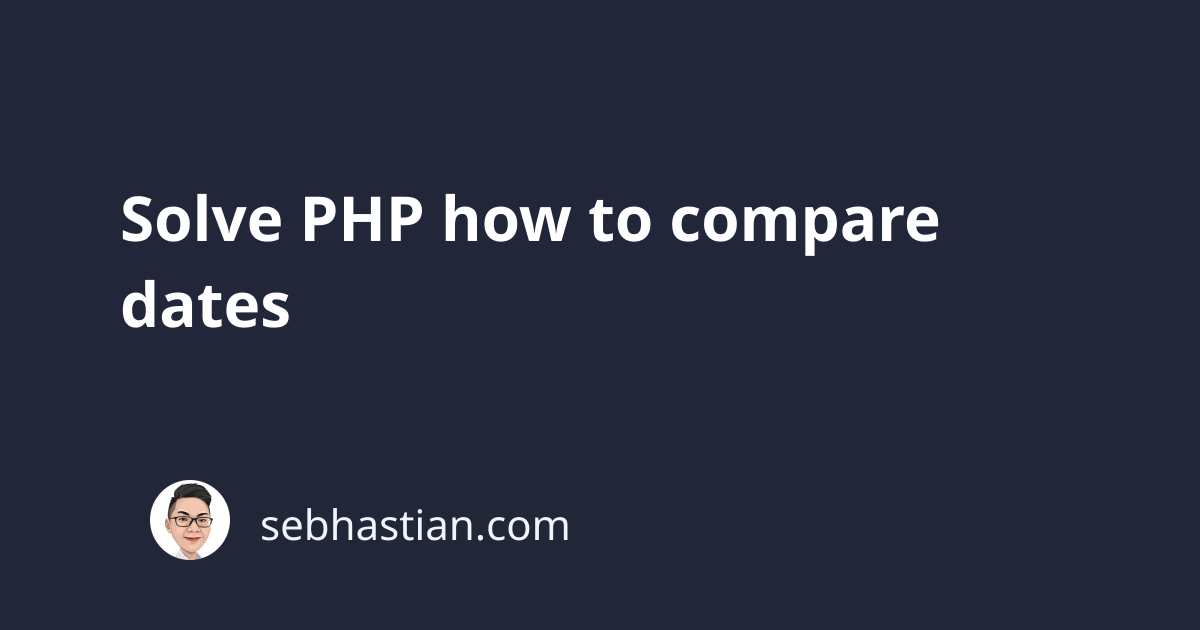
Most web applications today require you to work with date values, whether an e-commerce app or a ticket booking app.
This tutorial will help you learn how to compare dates in PHP.
PHP Compare dates in strings
When your dates are strings with the format YYYY-MM-DD
, then you can use the comparison operator to compare their values:
<?php
// compare dates in PHP
// 👇 declare two dates using string format
$date1 = "2020-10-11";
$date2 = "2019-09-15";
// 👇 compare if date1 is greater than date2
if ($date1 > $date2) {
print "$date1 is greater than $date2";
} else {
print "$date1 is less than $date2";
}
?>
PHP knows how to compare the two string dates above, so the output will be “2020-10-11 is greater than 2019-09-15”.
But this method won’t work when you have dates in other formats, so let’s see an alternative way to compare dates in PHP next.
Compare PHP dates using strtotime()
The PHP strtotime()
function converts an English description of time into a Unix timestamp.
A Unix timestamp is an integer
value representing the datetime value, so you can compare these timestamps using the comparison operator.
Here’s an example of using the strtotime()
function on dates:
// 👇 declare dates
$date1 = "12 January 2019";
$date2 = "today";
// 👇 convert to Unix timestamps
$date1_unix = strtotime($date1);
$date2_unix = strtotime($date2);
// compare dates
if ($date1_unix > $date2_unix) {
print "$date1 is in the future";
} else {
print "$date1 is in the past";
}
The value of date2
will change depending on when you run the code above, but the output will most likely be “12 January 2019 is in the past” (because this article is written in 2022 😉)
Converting time descriptions using strtotime()
allows you to compare dates accurately using Unix timestamps.
But since these timestamps are hard to read by humans, you should keep the original dates. That’s why there are $date1
and $date1_unix
variables in the example above.
Alternatively, you can also convert the result back to readable dates using date()
function:
$date = strtotime("today");
// 👇 after comparison is done, format back the timestamp
$date = date("Y-m-d", $date);
print $date; // 2022-09-23
And that’s how you use strtotime()
to compare dates in different formats.
Using DateTime object to compare dates
The PHP DateTime
class can be used to create DateTime
objects.
Like the strtotime()
function, you can compare DateTime
objects using the comparison operator.
The difference is that you need to use the format()
function to format the object when calling the print
construct.
Consider the following example:
// 👇 declare dates
$date1 = "2021/10/12 11:00:00";
$date2 = "2021-10-12 10:00:00";
// 👇 convert to DateTime objects
$date1 = new DateTime($date1);
$date2 = new DateTime($date2);
// compare dates
if ($date1 > $date2) {
print $date1->format("Y-m-d H:i:s") . " is greater";
} else {
print $date2->format("Y-m-d H:i:s") . " is greater";
}
When using the strtotime()
function, you need to use the date()
function to convert the timestamps into a readable format.
But creating DateTime
objects allows you to use the format()
function from the object to convert the dates
What’s more, the DateTime
object also enables you to find the difference between two dates:
// 👇 declare dates
$date1 = "2021/10/12 11:00:00";
$date2 = "2021-10-12 10:00:00";
// 👇 convert to DateTime objects
$date1 = new DateTime("2021/10/12 11:00:00");
$date2 = new DateTime("2021-10-12 10:00:00");
$diff = $date1->diff($date2);
print "Time difference: " . $diff->format("%H:%I:%S");
The code above will print “Time difference: 01:00:00”.
Now you’ve learned how to compare dates using DateTime
objects.
You are free to choose the method that fits your situation best.