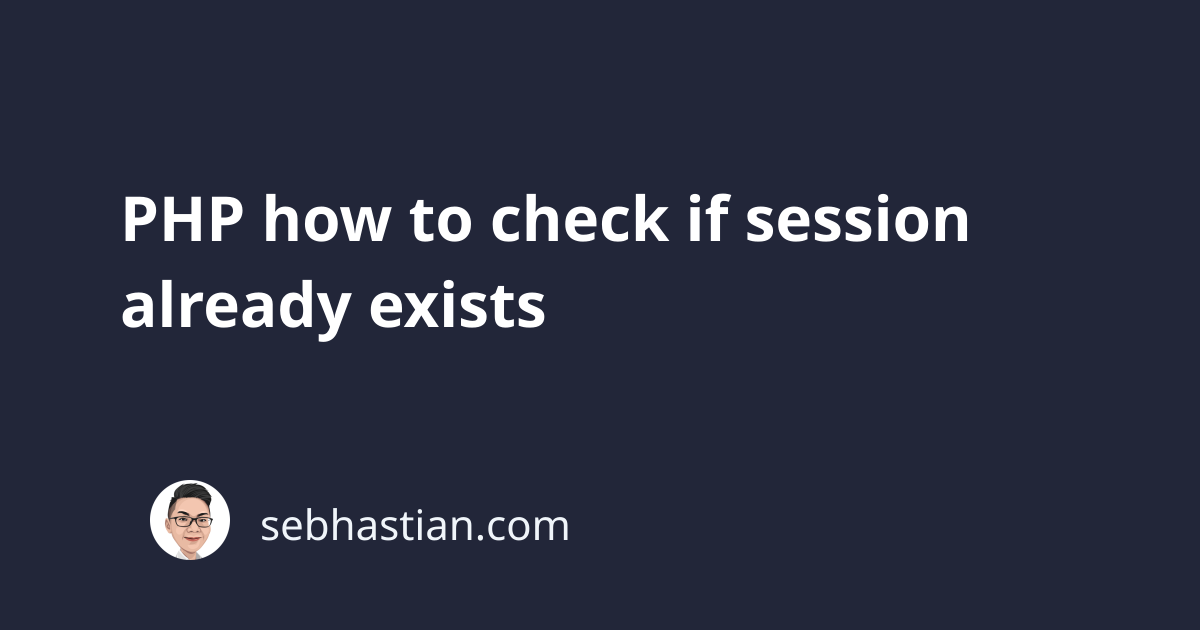
To check whether a session already exists or started in PHP, you can use the isset()
or session_status()
function.
The session_status()
function will return PHP_SESSION_ACTIVE
when you have sessions enabled and one exists.
You can use this function inside an if
block to check your session as follows:
<?php
// 👇 enable PHP session
session_start();
// 👇 check if session exists. returns true
if (session_status() == PHP_SESSION_ACTIVE) {
print "session already exists";
}
When you have called the session_start()
function, then a session has been created by PHP.
Besides using session_status()
, you can also use the isset()
function and check the $_SESSION
superglobal like this:
if (isset($_SESSION)) {
print "session exists";
}
Using the isset()
function also allows you to check if a specific key exists in the $_SESSION
array as shown below:
// 👇 check if userid key exists in $_SESSION
if (isset($_SESSION['userid'])) {
print "session exists";
}
Depending on your situation, you can choose to use the session_status()
or isset()
function to check if a session exists in PHP.