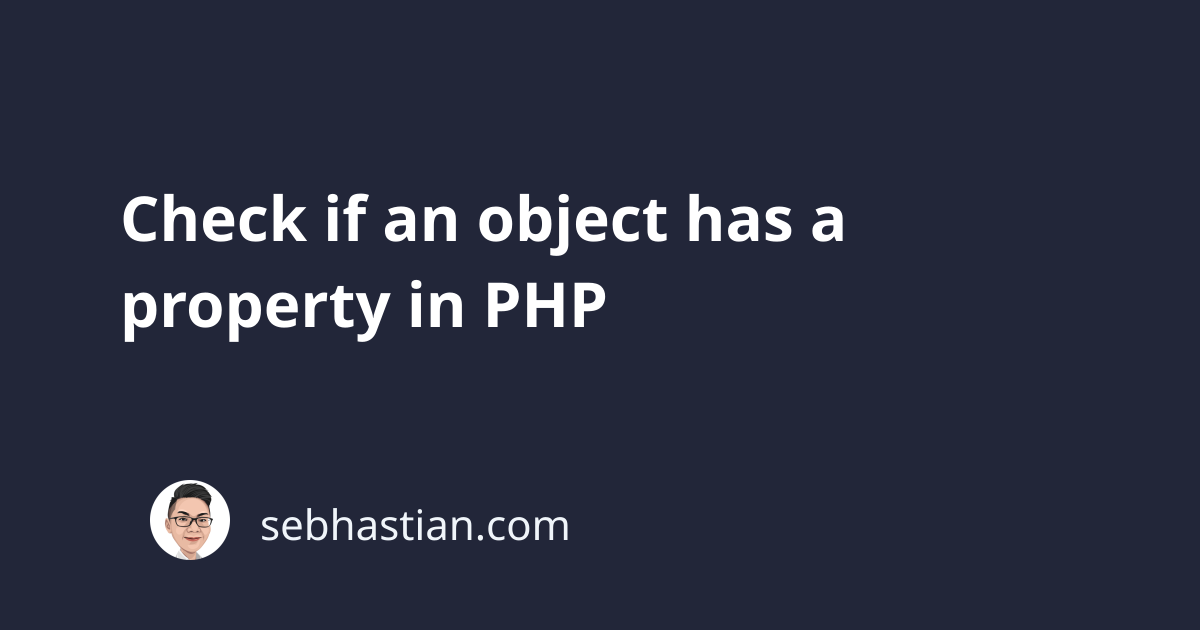
You can check whether a PHP object has a property or not by using the property_exists()
function.
The property_exists()
function can be used to check whether a property exists in a class or an object. The syntax is as follows:
property_exists(
object|string $object_or_class,
string $property
): bool
You need to pass two things:
- The object or class as the first argument
- The property in string as the second argument
Here’s an example of calling the function:
<?php
class Car {
public $type;
private $color;
}
// 👇 check class property
var_dump(property_exists("Car", "type")); // true
// 👇 check object property
var_dump(property_exists(new Car(), "color")); // true
// 👇 instantiate the object first
$car = new Car();
var_dump(property_exists($car, "wheels")); // false
?>
When checking the property of a class, you pass the class name as a string. When checking an object, you need to pass the object instance.
By passing the property name as a string as shown above, the property_exists()
function will check whether the property exists in the given class name or object.
Now you’ve learned how to check if an object has a certain property in PHP. Great!