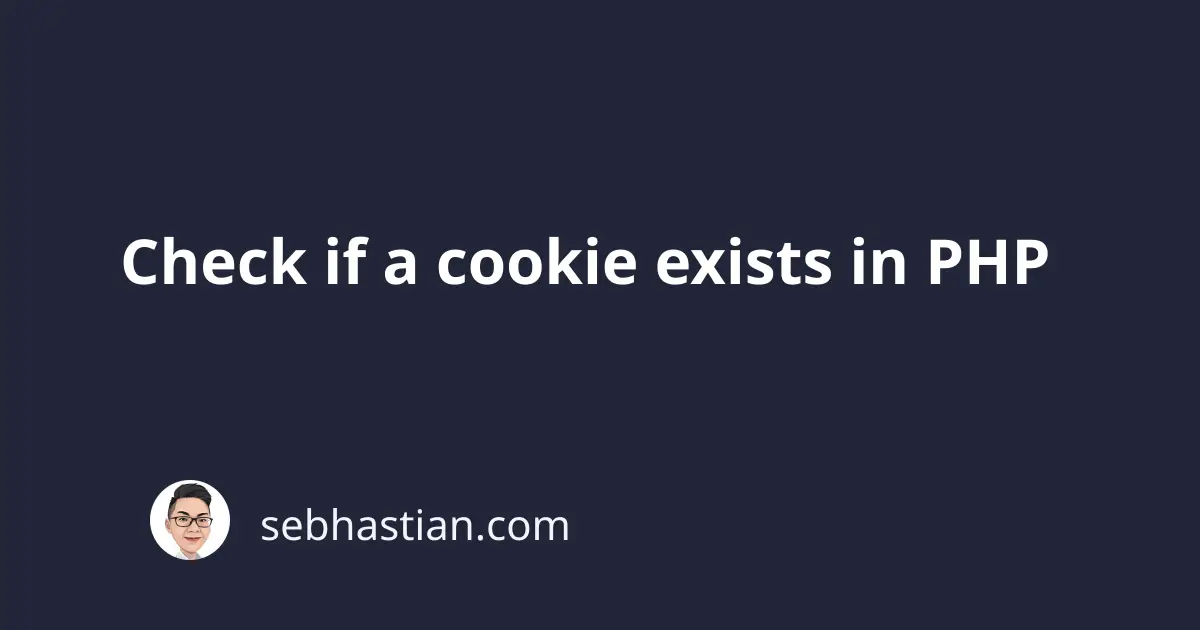
To check if a cookie exists in PHP, you need to call the isset()
function on the $_COOKIE
array variable.
For example, suppose you want to check for a cookie named lang
. You need to call the isset()
function inside an if
statement as shown below:
// ๐ check if cookie exists
if (isset($_COOKIE["lang"])) {
print "lang cookie value: " . $_COOKIE["lang"];
} else {
print "lang cookie doesn't exists";
}
Keep in mind that a cookie can only be accessed after the page has been reloaded.
This is because cookies are sent as part of the header response when you request a page.
Checking for the cookie existence immediately after setting it with setcookie()
will cause PHP to return undefined array key warning:
<?php
if (!isset($_COOKIE["lang"])) {
setcookie("lang", "ja");
}
// ๐ Warning: Undefined array key "lang"
// until next request
print $_COOKIE["lang"];
?>
If you want to check for the cookie without reloading, then you need to call the setcookie()
function and set the $_COOKIE
value in the next line.
This way, you can use that cookie without a page reload:
<?php
if (!isset($_COOKIE["lang"])) {
setcookie("lang", "ja");
$_COOKIE["lang"] = "ja";
}
// ๐ prints "ja"
print $_COOKIE["lang"];
?>
By setting the $_COOKIE
key-value pair manually, you tricked the code to think that the cookie is set.
But this may also introduce a bug since your code thinks that the user has the cookie in the first place.
As an alternative, you can add a fallback value as a variable instead:
if (!isset($_COOKIE["lang"])) {
setcookie("lang", "ja");
}
$fallback_lang = "en";
// ๐ if lang not set, prints fallback value
print isset($_COOKIE["lang"]) ? $_COOKIE["lang"] : $fallback_lang;
Using the ternary condition as shown above is considered the right approach because it doesnโt trick the code with a made-up cookie.
Now youโve learned how to check if a cookie exists in PHP. Good job! ๐