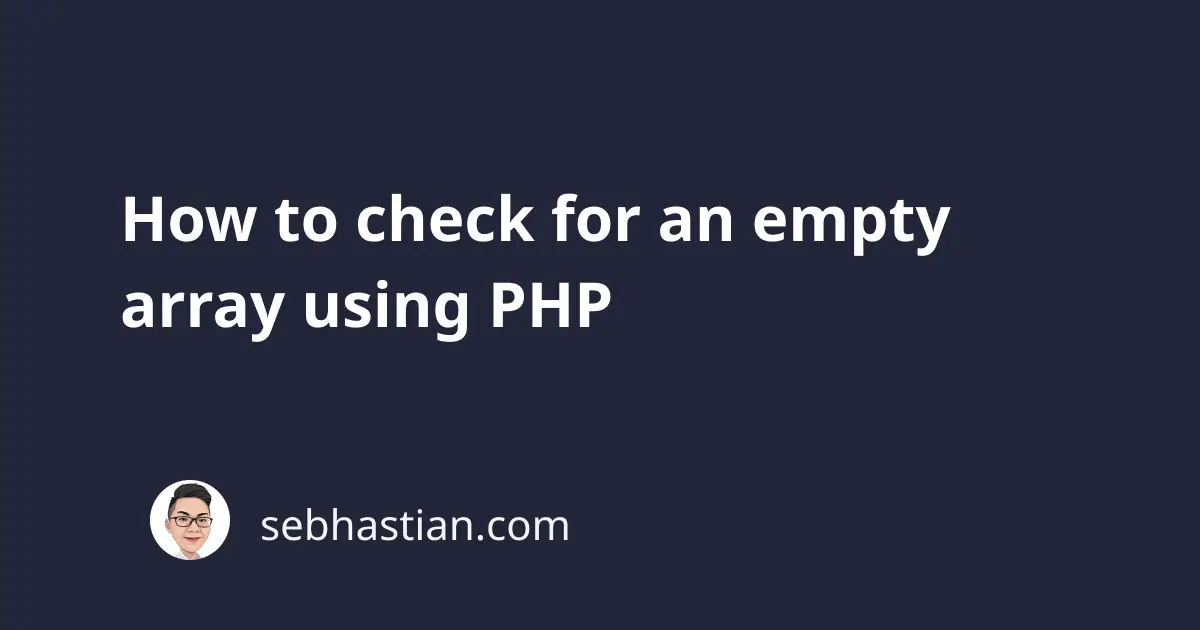
To check whether an array is empty or not in PHP, you can use the logical not operator using the bang symbol (!
).
Here’s an example of checking whether the $list
array is empty:
<?php
$list = [];
if (!$list) {
print "list is empty";
} else {
print "list has values!";
}
// Output: list is empty
To check whether the $list
array is empty or not, you create an if
condition that checks whether the $list
value is falsy (!$list
).
But if you haven’t declared the variable, the not operator will trigger a PHP warning:
<?php
// no declaration of $list in this code
// 👇 Warning: Undefined variable $list
if (!$list) {
print "list is empty";
} else {
print "list has values!";
}
To avoid PHP undefined variable warning, you can use the empty()
function in place of the not operator.
The empty()
function checks for two conditions:
- Whether a variable exists or not
- Whether the variable has value or not
The function returns false
when the variable exists and is not empty. Otherwise, it returns true
.
Here’s an example of using empty()
to check for an empty array:
<?php
if (empty($list)) {
print "list is empty";
} else {
print "list has values!";
}
// Output: list is empty
By calling the empty()
function, you avoid the PHP warning when the variable has not been declared.
Now you’ve learned how to check for empty arrays in PHP. Nice!