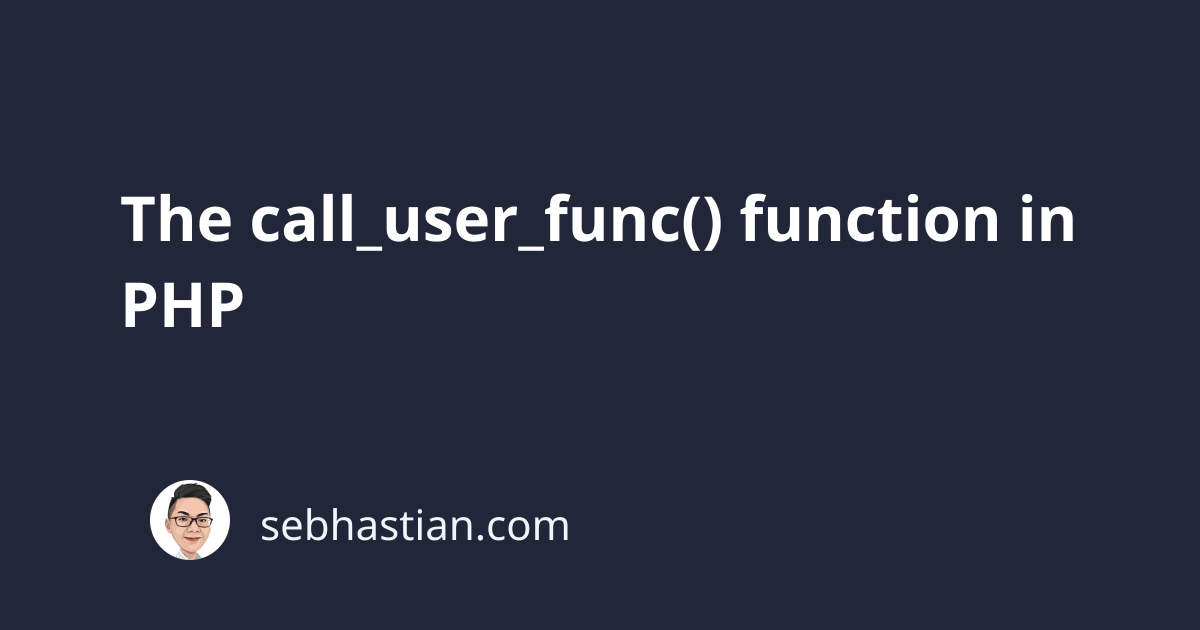
The PHP call_user_func()
function is used to call the function given by the first parameter.
The syntax of the function is as follows:
call_user_func(
callable $callback,
mixed ...$args
): mixed
The first parameter $callback
is the function you want to call.
The $args
are the parameters you want to pass to the $callback
function.
You need to specify the $callback
parameter as a string
as shown below:
function greetings() {
echo "Hello!";
}
call_user_func("greetings");
// Hello!
You can also pass parameters to the $callback
function like this:
function greetings($name) {
echo "Hello, $name!";
}
// 👇 pass the name parameter
call_user_func("greetings", "Nathan");
// Hello, Nathan!
Since PHP can call a function directly by name, the call_user_func()
is not needed until you need to call a function dynamically during runtime.
Back to the example above, you can call the greetings()
function directly as follows:
function greetings($name) {
echo "Hello, $name!";
}
greetings();
But suppose you don’t know the name of the function in advance, you can still call that function with something like this:
$function_name = "dynamic_function";
call_user_func($function_name);
The real $function_name
string could be something you generate at runtime.
You can also use this function to call a static class method as follows:
class Car {
static function start() {
echo "Start engine!";
}
}
$class_name = "Car";
$method_name = "start";
call_user_func("$class_name::$method_name");
// 👆 Start engine!
As you can see, you can have the class name and static method name dynamically stored inside variables. This is useful when you have them generated at runtime.
However, you shouldn’t use call_user_func()
when you have all the names available before running the code because it’s slower than a direct call.