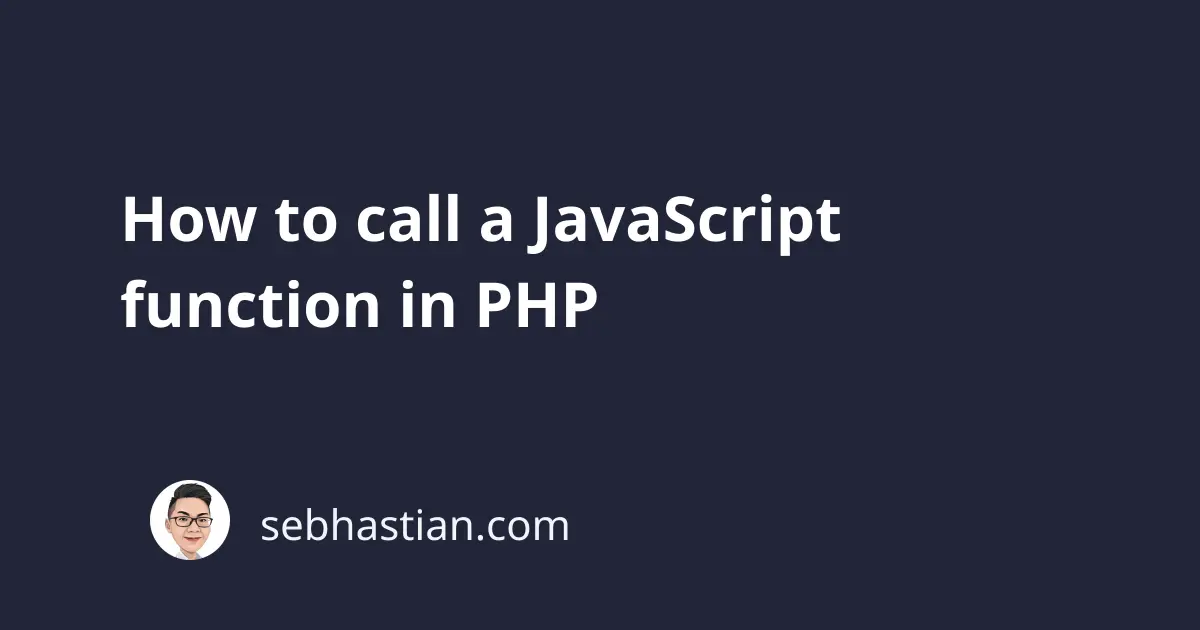
Sometimes, you might want to call a JavaScript function from the PHP part of your code.
A JavaScript function can be called from PHP using the echo
construct.
For example, here’s how you call a JavaScript function called hello()
:
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>Call JS Function</title>
<script>
function hello() {
alert('Hello World!');
}
</script>
</head>
<body>
<?php echo '<script>
hello();
</script>'; ?>
</body>
</html>
First, the JavaScript function is defined in the <head>
section of the HTML page.
Then, you can write the PHP code in the <body>
tag. You need to echo
an HTML <script>
tag that calls the JavaScript function.
Actually, you can remove the PHP tag and echo
construct from the HTML page as shown below:
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>Call JS Function</title>
<script>
function hello() {
alert('Hello World!');
}
</script>
</head>
<body>
<script>
hello();
</script>
</body>
</html>
PHP is a server-side language and JavaScript is a client-side language.
PHP can’t run in the browser, so your best option is to run the JavaScript function using echo
.
But since echo
is just putting things in the HTML page, that means you can call the JavaScript function from HTML directly as shown above.
PHP has already finished execution when the HTML/ JavaScript part is processed.
Anyway, that’s how you call a JavaScript function using PHP code.