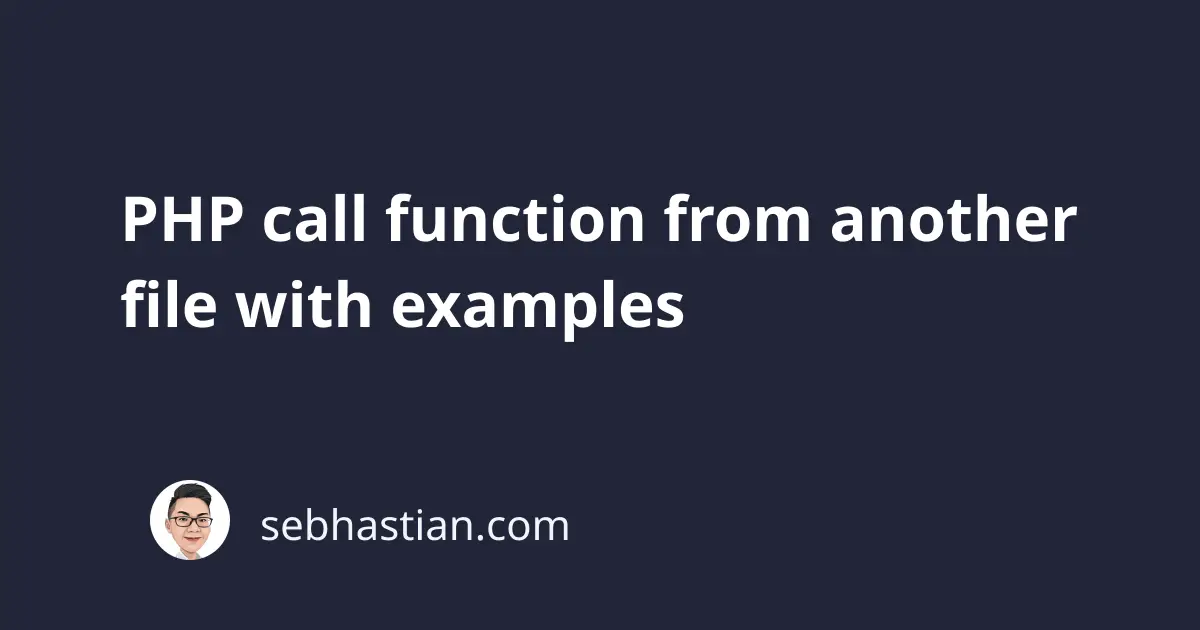
To call a function from another file in PHP, you need to import the file where the function is defined before calling it.
You can import a PHP file by using the require
statement.
For example, suppose you have a file named library.php
with the following content:
// library.php
function greetings() {
echo "Hello World!";
}
To call the greetings()
function from another file, you need to import the library.php
file as shown below:
// 👇 import the PHP file
require "library.php";
// 👇 call the function
greetings();
By using the require
statement, PHP will evaluate the code in that file before running the current file.
The same method works when you have a function with parameters as well:
// library.php
<?php
function sum(int $x, int $y) {
return $x + $y;
}
Just add the parameters when calling the function in another file as follows:
// index.php
<?php
require "library.php";
// 👇 call the function with parameters
$n = sum(10, 9);
echo $n; // 19
Now you’ve learned how to call a function from another file in PHP. Nice work! 👍