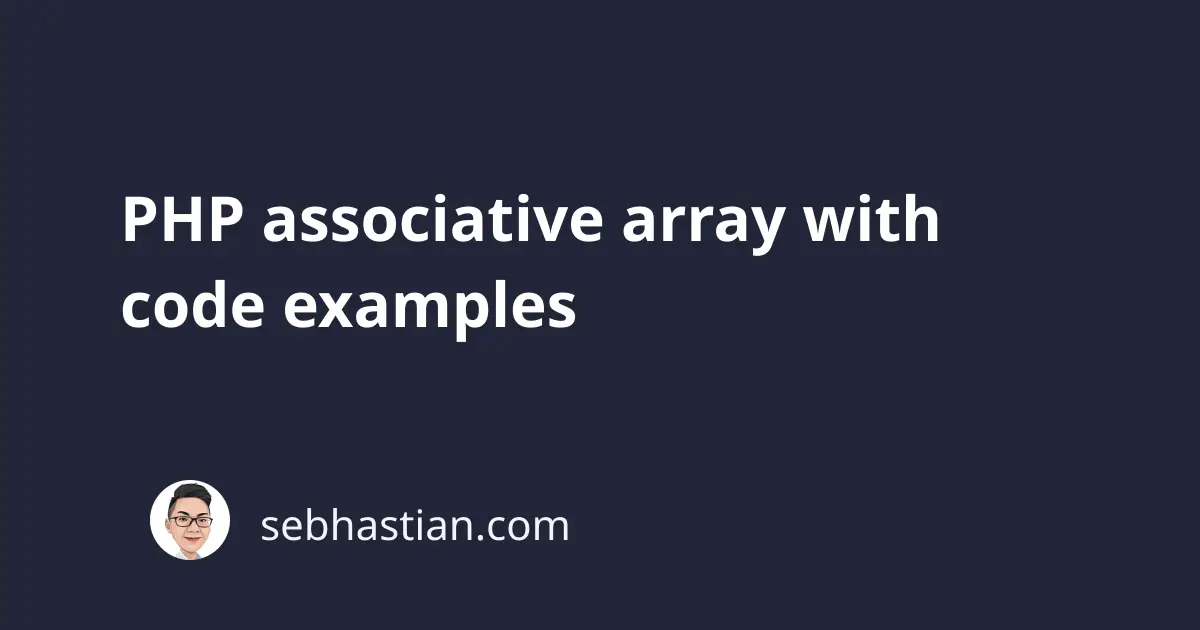
An associative array is an array that stores its values using named keys that you assign manually to the array.
The named key can then be used to refer to the value being stored in that specific key.
This tutorial will help you learn how to create an associative array in PHP.
PHP declare associative array
An associative array can be declared in PHP by calling the array()
function.
The following example shows how you create an associative array of each person’s age:
$age = array('John' => 40, 'Mia' => 29, 'Joe' => 20);
Or you can also use the array index assignment syntax as shown below:
$age['John'] = 40;
$age['Mia'] = 29;
$age['Joe'] = 20;
You can then call the array value using the array key like this:
echo "John is {$age['John']} years old.";
// 👆 John is 40 years old.
The associative array key can be a string
or an int
. When you put a boolean
value as a key, then it will be cast as an int
(true
as 1
and false
as 0
).
Override the stored value in PHP associative arrays
When you use the same key for several elements, then only the last element will be put in the array.
In the example below, the key John
is used twice:
$age = array(
'John' => 40,
'Mia' => 29,
'John' => 20
);
echo $age['John']; // 👉 20
You can override the value stored in a specific key by reassigning the key with a new value:
$age['Mia'] = 29;
$age['Mia'] = 17;
echo $age['Mia']; // 👉 17
Loop through PHP associative arrays
An associative array is treated as a regular array, so you can loop through the array using either foreach
or for
statement.
Here’s how to loop through an associative array using the foreach
syntax:
$age = array(
'John' => 40,
'Mia' => 29,
'Joe' => 20
);
foreach ($age as $key => $value){
echo "{$key} is {$value} years old.\n";
}
The output will be as follows:
John is 40 years old.
Mia is 29 years old.
Joe is 20 years old.
You can also use the for
statement to loop through an associative array in PHP.
The following code will produce the same result:
$age = array(
'John' => 40,
'Mia' => 29,
'Joe' => 20
);
$keys = array_keys($age);
for ($i = 0; $i < count($keys); $i++) {
echo "{$keys[$i]} is {$age[$keys[$i]]} years old.\n";
}
First, you need to get all the array keys as an array using the array_keys()
function.
Then, write a for
statement that loops until it reaches the $keys
count.
For each loop, echo the $keys
and the $age
value.
While you can achieve the same result using foreach
and for
statements, it’s more recommended to use the foreach
statement because the code is more intuitive.
Now you’ve learned how to loop through an associative array in PHP. Nice work! 👍