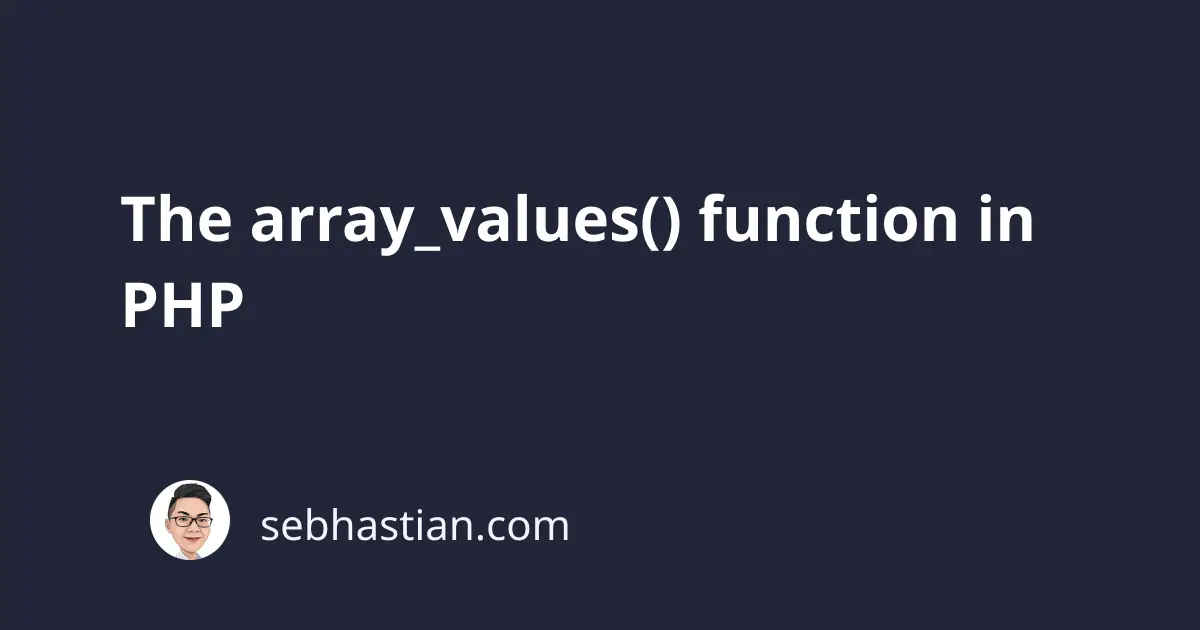
The PHP array_values()
function is used to get all elements present in an array.
This function accepts an array
and returns an array
:
array_values(array $arr): array
The array_values()
function returns an array with numbered keys.
Here’s an example of calling the function:
$arr = [
"name" => "Nathan",
"age" => 27
];
print_r(array_values($arr));
The above code will produce the following output:
Array
(
[0] => Nathan
[1] => 27
)
As you can see, the keys of the original array will not be carried to the new array, only the values.
array_values()
function will ignore keys in your array, whether they are numeric or string.
You can also call this function on a multi-dimensional array:
$arr = [
"name" => "Nathan",
"age" => 27,
"skills" => [
"React",
"Vue",
"JavaScript"
]
];
print_r(array_values($arr));
The code above produces the following output:
Array
(
[0] => Nathan
[1] => 27
[2] => Array
(
[0] => React
[1] => Vue
[2] => JavaScript
)
)
And that’s how the array_values()
function works in PHP.
If you want to get the keys of the array instead of values, you can use the array_keys() function.