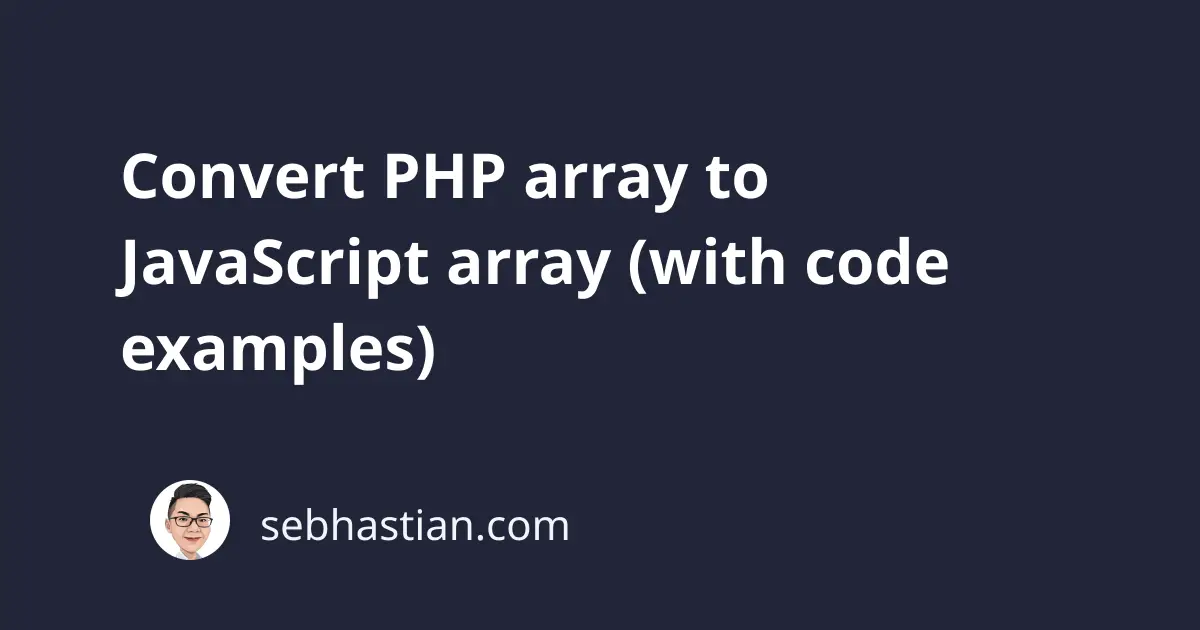
A PHP array can be converted into a JavaScript array by using the json_encode()
function.
This tutorial will help you learn how to convert a single, multi-dimensional, indexed, and associative PHP array to a JavaScript array.
Convert PHP single array to JavaScript array
When you have a single PHP array, you can convert it to a JavaScript array by calling the json_encode()
PHP function.
You need to store the result of json_encode()
in a JavaScript variable as follows:
<?php
// π create a PHP array
$users = ["Nathan", "Jack", "Jane"];
?>
<body>
<!-- π Add a script tag for JavaScript -->
<script>
const users = <?php echo json_encode($users); ?>;
console.log(Array.isArray(users));
console.log(users);
</script>
</body>
The console log results will be as follows:
true
(3) ['Nathan', 'Jack', 'Jane']
Thatβs how you convert a single PHP array to a JavaScript array.
Convert PHP multi-dimensional array to JavaScript array
Since JavaScript also has a multi-dimensional array concept, you can convert a multi-dimensional PHP array to JavaScript using json_encode()
too.
Consider the following example:
<?php
// π a PHP multi-dimensional array
$users = [
["apple", "orange"],
["cucumber", "lettuce"],
["rose", "jasmine"]
];
?>
<body>
<!-- π Add a script tag for JavaScript -->
<script>
const users = <?php echo json_encode($users); ?>;
console.log(Array.isArray(users));
console.log(users);
</script>
</body>
The console log output is as shown below:
true
(3) [Array(2), Array(2), Array(2)]
(2) ['apple', 'orange']
(2) ['cucumber', 'lettuce']
(2) ['rose', 'jasmine']
Convert PHP associative array to JavaScript array
When you have an associative PHP array, calling json_encode()
will transform the array into a JavaScript object.
Since a JavaScript object is similar to an associative array, so you can access the value using the key as well.
Consider the example below:
<?php
// π create a PHP associative array
$my_array = [
"name" => "nathan",
"age" => 28,
];
?>
<body>
<!-- π Add a script tag for JavaScript -->
<script>
const arr = <?php echo json_encode($my_array); ?>;
// π the result will not be an array according to JavaScript
console.log(Array.isArray(arr));
// π access the object properties like associative array
console.log(arr["name"]);
console.log(arr["age"]);
</script>
</body>
The console logs above will produce the following output:
false
nathan
28
As you can see, the Array.isArray()
function returns false
because the arr
variable is identified as an object, not an array.
In JavaScript, an associative array is identified as an object but not an array.
Although itβs not identified as an array, you can use the same syntax to access the associative arrayβs value as shown in the example above.
Convert PHP indexed array to JavaScript array
PHP and JavaScript have the same default indexing system for arrays, so you can convert an indexed array like a regular array:
<?php
// π create a PHP array
$users = [
0 => "Nathan",
1 => "Jack",
2 => "Jane"
];
?>
<body>
<script>
const users = <?php echo json_encode($users); ?>;
console.log(Array.isArray(users));
console.log(users);
</script>
</body>
When you have an array with custom index numbers, then JavaScript interprets the array as an object.
But itβs okay because you can still access the array value using the index as shown below:
<?php
// π custom index for the array
$users = [
11 => "Nathan",
22 => "Jack",
33 => "Jane"
];
?>
<body>
<script>
const users = <?php echo json_encode($users); ?>;
console.log(Array.isArray(users));
console.log(users);
console.log(users[33]);
</script>
</body>
The code above will produce the following logs:
false
{11: 'Nathan', 22: 'Jack', 33: 'Jane'}
Jane
Even though the indexed array is converted into an object, you can access the values just like an array.
Now youβve learned how to convert a PHP array to a JavaScript array. Nice work! π