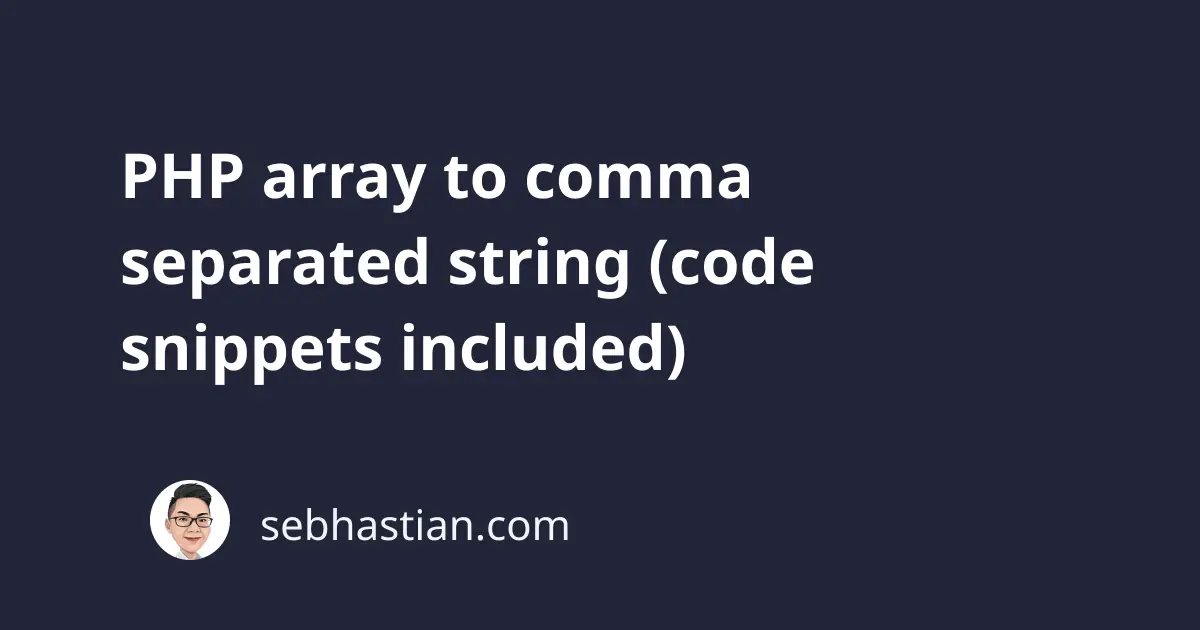
To convert a PHP array into a comma separated string, you need to use the implode()
function.
For a multi dimension array, you need to use the array_column()
function to grab the array values you want to convert to string.
This tutorial will help you convert:
Letβs begin.
PHP single dimension array to comma separated string
The implode()
function is used to join array elements as a string.
The function syntax is as follow:
implode(string $separator, array $array): string
To create a comma separated string from an array, pass a comma (,
) as the $separator
argument into the implode()
function.
Consider the example below:
<?php
// π create a PHP array
$animals = ["eagle", "leopard", "turtle"];
// π create a comma separated string from array
$array_string = implode(",", $animals);
// π print the string
print $array_string;
?>
The code above will produce the following output:
eagle,leopard,turtle
You can also add a space after the comma to make the string easier to read:
<?php
// π create a PHP array
$animals = ["eagle", "leopard", "turtle"];
// π create a comma separated string from array
$array_string = implode(", ", $animals);
// π print the string
print $array_string;
?>
The output:
eagle, leopard, turtle
PHP multi dimension array to comma separated string
The implode()
function canβt handle a multi dimension array.
When you pass a multi dimension array, the function produces a warning and returns the wrong result.
Consider the following example:
<?php
// π create multi dimension array
$users = [
[
"id" => 2135,
"first_name" => "John",
],
[
"id" => 3245,
"first_name" => "Sally",
],
[
"id" => 5342,
"first_name" => "Jane",
],
[
"id" => 5623,
"first_name" => "Peter",
],
];
// π call implode
$array_string = implode(", ", $users);
// π print the string
print $array_string;
The code above will produce the following output:
Warning: Array to string conversion in ... on line ...
Array, Array, Array, Array
To create a comma separated string from a multi dimension array, you have to extract the column you want to process using the array_column()
function first.
The syntax is as follows:
array_column(
array $array,
int|string|null $column_key,
int|string|null $index_key = null
): array
The array_column()
function return the values of an array that has a specific $column_key
.
Once you have a single array returned from array_column()
, call the implode()
function.
For example, suppose you want to create a comma separated string from the first_name
data. Hereβs how you do it:
<?php
// π create multi dimension array
$users = [
[
"id" => 2135,
"first_name" => "John",
],
[
"id" => 3245,
"first_name" => "Sally",
],
[
"id" => 5342,
"first_name" => "Jane",
],
[
"id" => 5623,
"first_name" => "Peter",
],
];
// π get the first_name data
$first_names = array_column($users, 'first_name');
// π call implode
$array_string = implode(", ", $first_names);
// π print the string
print $array_string;
The code above will have the following output:
John, Sally, Jane, Peter
You can also pass an int
as the $column_key
argument to extract the value at a specific index.
Hereβs an example:
// π a multi dimension array without string keys
$animals = [
["eagle"],
["leopard"],
["turtle"]
];
// π get the animals data using index
$animal_names = array_column($animals, 0);
// π call implode
$array_string = implode(", ", $animal_names);
// π print the string
print $array_string;
The output:
eagle, leopard, turtle
Now youβve learned how to convert a PHP array to a comma separated string.
Youβve also seen examples of converting a single dimension and multi dimension PHP array.
I hope this tutorial has been useful for you π