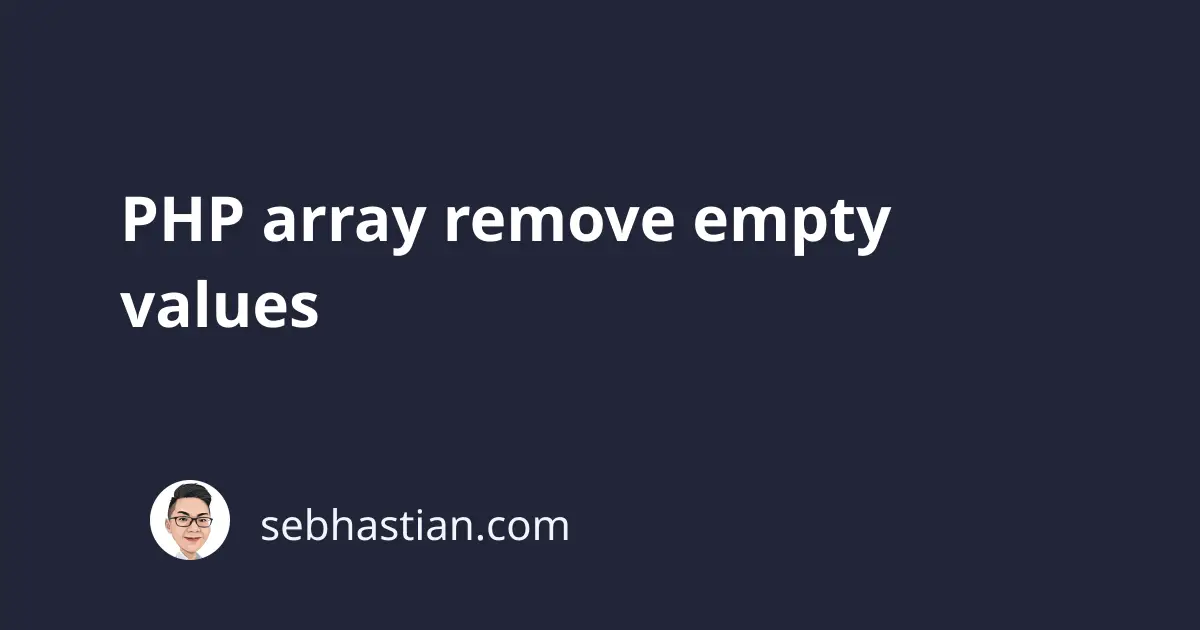
To remove empty values from your PHP array, you need to call the array_filter()
function without passing a callback function.
When no callback function is supplied, array_filter()
will remove any element that is empty.
PHP considers the following values to be empty:
0
0.0
false
null
""
''
array()
[]
Let’s see an example of removing the empty values in an array:
<?php
$arr = [
"Nathan",
"",
29,
0,
null,
true,
false,
"Jane",
[]
];
$new_arr = array_filter($arr);
print_r($new_arr);
The output of the code above will be:
Array
(
[0] => Nathan
[2] => 29
[5] => 1
[7] => Jane
)
If you want to include values like FALSE
and 0
in your array, then you need to pass a callback function to the array_filter()
as shown below:
$new_arr = array_filter($arr, function($val){
return ($val !== NULL && $val !== "" && $val !== []);
});
print_r($new_arr);
Running the callback to the same array will give the following output:
Array
(
[0] => Nathan
[2] => 29
[3] => 0
[5] => 1
[6] =>
[7] => Jane
)
The index 6
in the output is empty because PHP doesn’t print out false
by default.
Keep in mind that the array index now has gaps, as shown in both examples above.
If you need to remove the gaps, you can call the array_values()
function to reset the keys:
$new_arr = array_filter($arr);
$new_arr = array_values($new_arr);
print_r($new_arr);
The print_r()
output will have the keys reset as follows:
Array
(
[0] => Nathan
[1] => 29
[2] => 1
[3] => Jane
)
Now you’ve learned how to remove empty values from a PHP array. Nice work! 😉