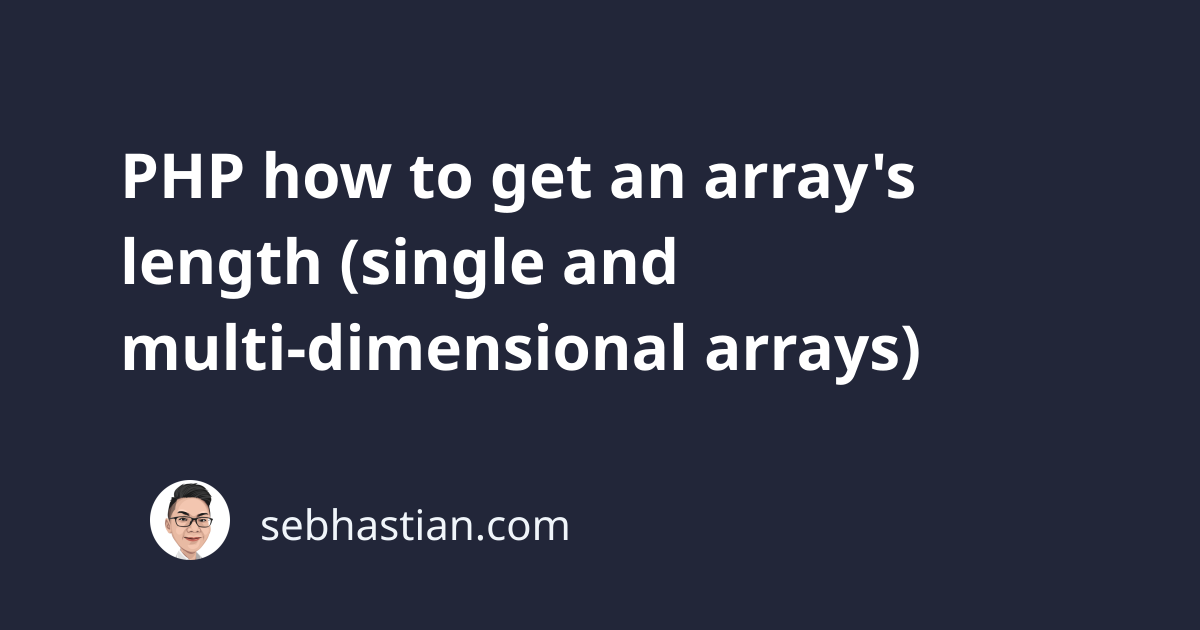
To get an array’s length in PHP, you can use either the sizeof()
or count()
function.
The sizeof()
function is just an alias of the count()
function.
When you want to find the length of a single-dimension array, you need to pass the array as an argument to the count()
function as shown below:
<?php
$days = ["Monday", "Tuesday", "Wednesday"];
// Printing array length
print count($days); // 3
print PHP_EOL;
print sizeof($days); // 3
When you want to find the length of a multi-dimensional array, you need to pass a second parameter to the count()
function.
The second parameter is the count_mode
parameter, and it allows you to count an array recursively.
Consider the following example:
<?php
$developers = [
["name" => "Nathan", "Skills" => ["PHP, MySQL"]],
["name" => "Jane", "Skills" => ["HTML, CSS"]],
["name" => "Emma", "Skills" => ["Ruby, JavaScript"]],
];
// Get array length with normal count mode
print count($developers, COUNT_NORMAL); // 3
print PHP_EOL;
// Get array length with recursive count mode
print count($developers, COUNT_RECURSIVE); // 12
When you didn’t pass the second parameter, PHP will use the default value COUNT_NORMAL
as the second parameter.
When you use COUNT_RECURSIVE
mode, then PHP will count all elements contained in a multi-dimensional array.
Now you’ve learned how to count an array’s length in PHP. Nice!