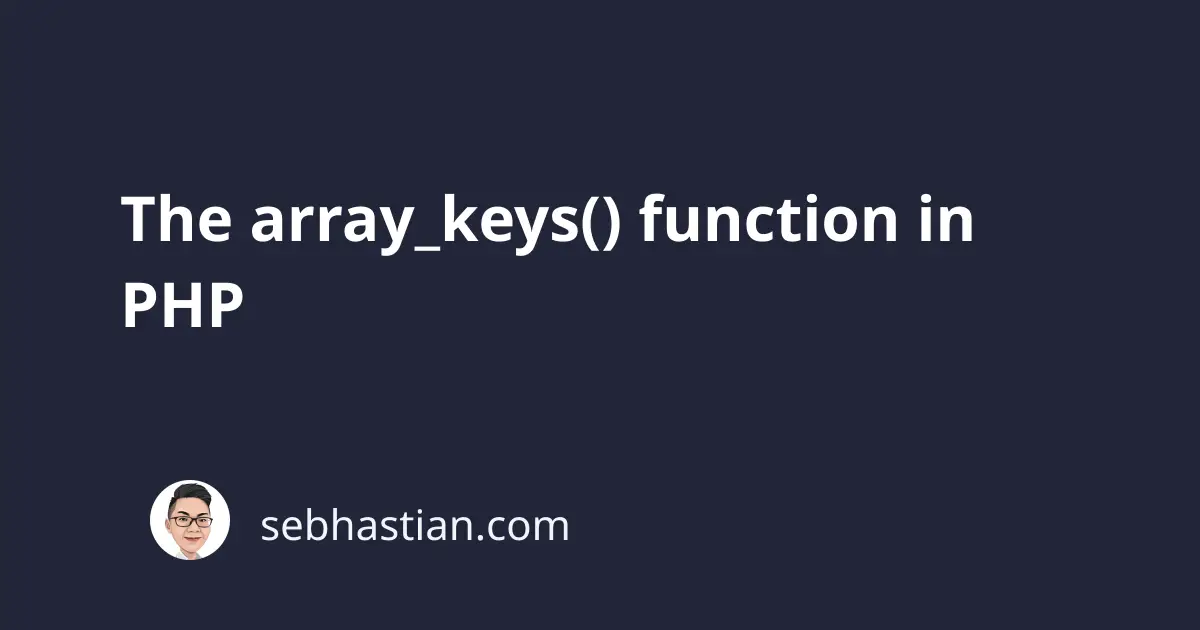
The PHP array_keys()
function is used to retrieve the keys or index of an array.
The function syntax is as shown below:
array_keys(
array $array,
mixed $search_value,
bool $strict = false
): array
This function has three parameters:
$array
is the array you want to get the keys from$search_value
when specified, only keys containing this value are returned (optional)$strict
variable, use===
strict comparison for the$search_value
(optional)
Here’s an example of calling the array_keys()
function:
$arr = [
"name" => "Nathan",
"age" => 27,
"skills" => [
"PHP",
"JavaScript"
]
];
print_r(array_keys($arr));
The code above produces the following output:
Array
(
[0] => name
[1] => age
[2] => skills
)
When you have an array with no custom keys, then this function will return the default numeric keys starting from 0
:
$arr = ["Lion", "Tiger"];
print_r(array_keys($arr));
The output of the above code will be as follows:
Array
(
[0] => 0
[1] => 1
)
You can also choose to retrieve only keys of a specific value as shown below:
$arr = [
"name" => "Nathan",
"age" => 27,
"role" => "PHP dev",
"username" => "Nathan",
];
print_r(array_keys($arr, "Nathan"));
Because name
and username
have the same value as specified in the array_keys()
function, both will be retrieved:
Array
(
[0] => name
[1] => username
)
One good use of the $search_value
is to retrieve all keys with non-null values:
$arr = [
"name" => "Nathan",
"age" => 27,
"role" => "PHP dev",
"username" => null,
];
print_r(array_keys($arr, !null));
array_keys()
will exclude username
from the code above:
Array
(
[0] => name
[1] => age
[2] => role
)
And that’s how the PHP array_keys()
function works.
You can also get only array values using the PHP array_values() function.