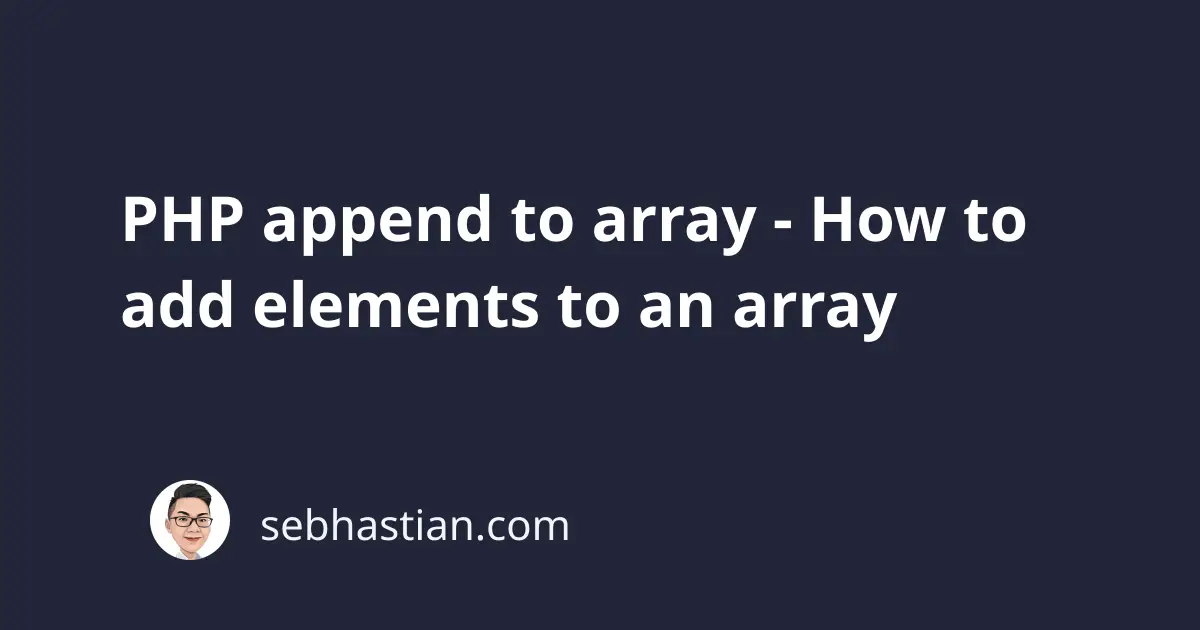
To append an element to an array, you can use either the array_push()
function or the index assignment syntax.
The array_push()
function accepts at least two arguments:
- The
$array
you want to add new elements into - The
$values
you want to append to the$array
You can pass as many $values
that you want to append to the array.
Hereโs an example of the array_push()
function in action:
<?php
$arr = [1, 2];
// ๐ append to array
array_push($arr, 3, 4, 5);
print_r($arr);
// ๐ output:
// Array
// (
// [0] => 1
// [1] => 2
// [2] => 3
// [3] => 4
// [4] => 5
// )
The array_push()
function will add new array elements to the end of the array.
Alternatively, you can also use the array index assignment syntax in the form of $arr[] =
to append an element to the array.
Consider the code example below:
<?php
$arr = [1, 2];
// ๐ append to array
$arr[] = 3;
$arr[] = 4;
$arr[] = 5;
print_r($arr);
// ๐ output:
// Array
// (
// [0] => 1
// [1] => 2
// [2] => 3
// [3] => 4
// [4] => 5
// )
As you can see, both the array_push()
function and the index assignment syntax produce the same result.
Which method should you use to append elements to an array?
When you need to add a single element to your array, itโs better to use the index assignment syntax to eliminate the overhead of calling a function.
But if you have several elements to add to an existing array, then you should use the array_push()
function. Using the index assignment syntax causes you to repeat your code as seen above.
And now youโve learned how to append elements to an array. Nice! ๐