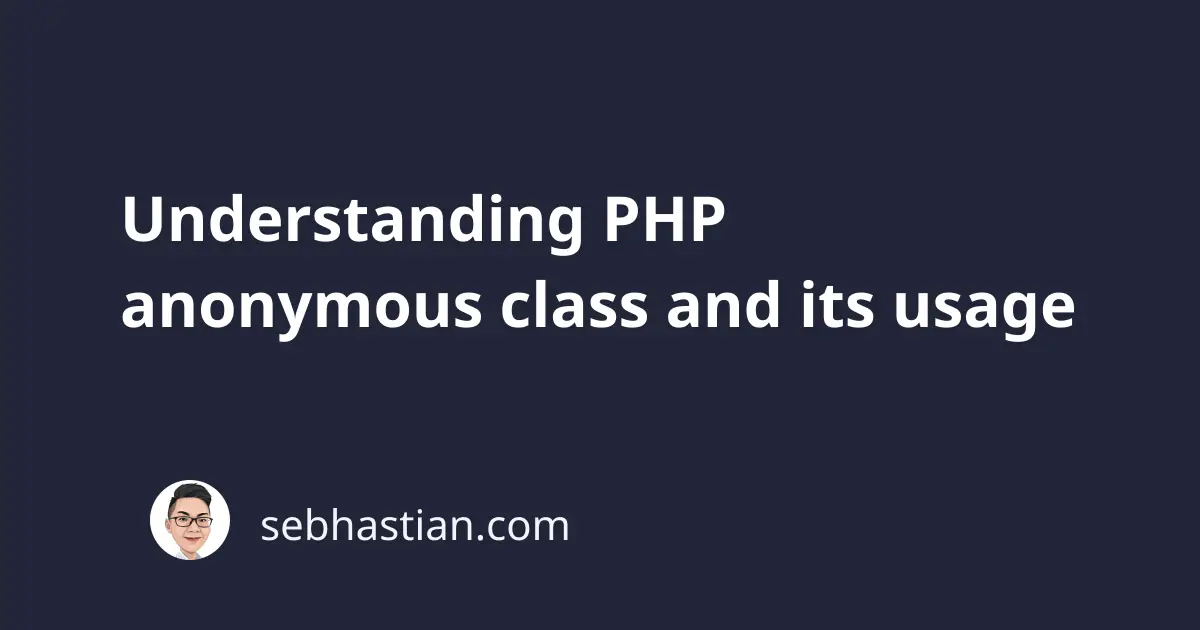
PHP anonymous classes are a relatively recent addition to the PHP language, having been introduced in PHP version 7.0.
An anonymous class is a class that has no name. It is defined and instantiated in a single expression, making it useful for short, one-time use cases.
Anonymous classes are similar to regular classes in that they can have properties and methods, and they can extend other classes and implement interfaces.
However, because they are anonymous, they cannot be referenced by name and can only be used in the context in which they are created.
One of the primary uses of anonymous classes is to create simple, one-time objects.
For example, the following code uses a regular class to create a Human
object:
class Human {
public function greet() {
echo "Hello World!";
}
}
$john = new Human();
$john->greet();
The above code defines a Human
class, which has a single greet()
method for printing the “Hello World!” message.
A Human
object is then created and the greet()
method is called.
The code can be rewritten using an anonymous class, like this:
$john = new class {
public function greet() {
echo "Hello World!";
}
};
$john->greet();
In the above code, there is no definition of the Human
class.
Instead, an anonymous class is defined and instantiated is defined and instantiated in a single expression using the new class
syntax.
This anonymous class has the same greet()
method as the original Human
class, but it is defined inline and cannot be referenced by name.
While a named class can have many instances, an anonymous class can only have one instance.
This is why it’s useful for one-time use in a specific context.
Implementing an interface to an anonymous class
Anonymous classes can also implement an interface like a named class.
For example, the following Dog
class implements the Animal
interface:
interface Animal {
public function sound();
}
class Dog implements Animal {
public function sound() {
echo "Dog goes bark! Woof!";
}
}
$poodle = new Dog();
$poodle->sound(); // Output: Dog goes bark! Woof!
The above example defines an Animal
interface with a single sound()
method. It then defines a Dog
class that implements this interface, providing an implementation of the sound()
method.
The example can be rewritten using an anonymous class, like this:
interface Animal {
public function sound();
}
$poodle = new class implements Animal {
public function sound() {
echo "Dog goes bark! Woof!";
}
};
$poodle->sound();
In the above code, the Dog
class has been replaced with an anonymous class that implements the Animal
interface.
This anonymous class has the same sound()
method as the regular class, but you can’t create another instance without repeating the new class
syntax.
Extending another class
Anonymous classes can also extend other classes and override their methods.
For example, the following code defines a regular class named AdminUser
that extends the User
class and overrides the getFullName()
method:
class User {
protected $firstName;
protected $lastName;
public function __construct($firstName, $lastName) {
$this->firstName = $firstName;
$this->lastName = $lastName;
}
public function getFullName() {
return $this->firstName . " " . $this->lastName;
}
}
class AdminUser extends User {
public function getFullName() {
return "Admin " . parent::getFullName();
}
}
$user = new AdminUser("Salmon", "Rue");
echo $user->getFullName(); // output: "Admin Salmon Rue"
This code defines a User
class with a getFullName()
method that returns the firstName
and lastName
values combined.
The AdminUser
class then extends the User
class and overrides the getFullName()
method to prefix the user’s name with “Admin”.
Finally, it creates an instance of the AdminUser
class and uses it to get the user’s full name.
This code can be rewritten using an anonymous class, like this:
class User {
protected $firstName;
protected $lastName;
public function __construct($firstName, $lastName) {
$this->firstName = $firstName;
$this->lastName = $lastName;
}
public function getFullName() {
return $this->firstName . " " . $this->lastName;
}
}
$user = new class ("Salmon", "Rue") extends User {
public function getFullName() {
return "Admin " . parent::getFullName();
}
};
echo $user->getFullName(); // output: "Admin Salmon Rue"
In this code, the AdminUser
class has been replaced with an anonymous class that extends the User
class and overrides the getFullName()
method.
This anonymous class has the same functionality as the AdminUser
class, but it is defined inline and cannot be referenced by name.
Conclusion
In conclusion, PHP anonymous classes are a convenient way to define and instantiate classes in a single expression.
An anonymous class can do many things that a named class does, such as implementing an interface or extending a class.
It can be useful when you only create one object instance of a class, decreasing the amount of code you write and making it easier to read.
Also, anonymous classes can be used to avoid naming conflicts. Because they have no name, they cannot clash with other classes with the same name in your codebase.
This can be useful in situations where you need to create multiple classes that have the same functionality, but with different behavior.
Now you’ve learned what is an anonymous class and how to use it in PHP. Great work!