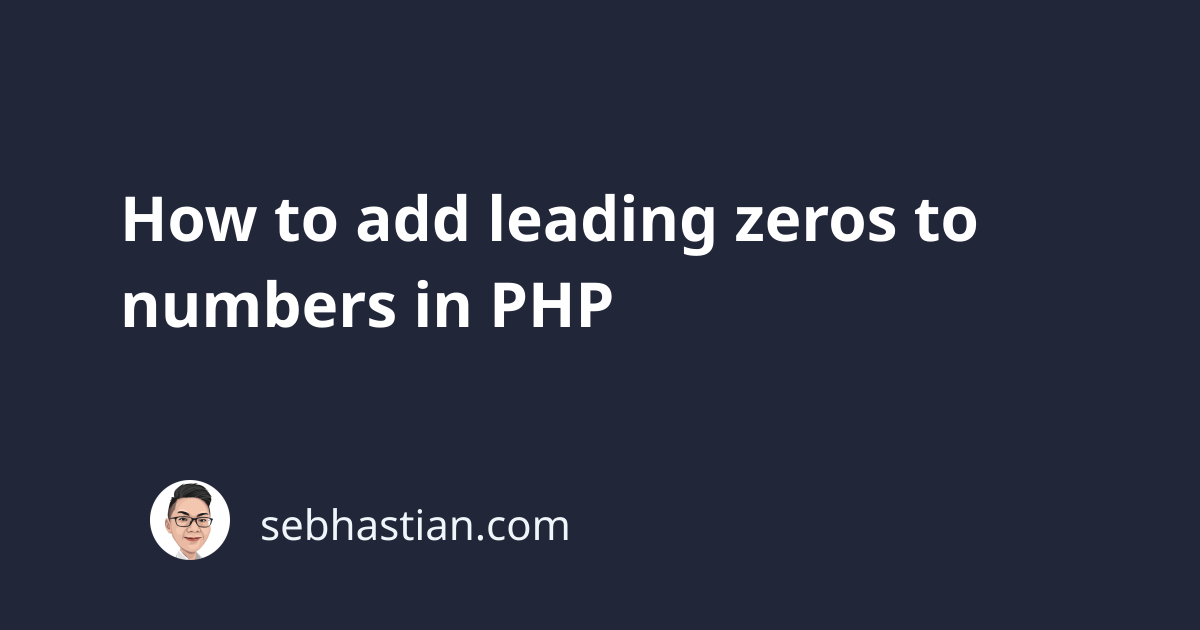
Sometimes, you need to add pad a number with leading zeros like numbers displayed in electrical devices.
To add leading zeros to numbers in PHP, you need to format your number as a string using the sprintf()
function.
Hereโs an example of creating a 4 digit number with sprintf()
:
<?php
// ๐ 4 digit pad numbers
print sprintf("%04d", 333); // 0333
print PHP_EOL;
print sprintf("%04d", 1234); // 1234
print PHP_EOL;
print sprintf("%04d", 12); // 0012
?>
In the sprintf()
function above, the format of the string is defined as โformat the integer with 4 digits (4d
) and pad it with leading zeros (0
)โ.
To change the format, you only need to adjust the number before the d
letter.
The example below shows how to create 6
and 8
digit numbers:
<?php
// ๐ 6 digit pad numbers
print sprintf("%06d", 1234); // 001234
print PHP_EOL;
// ๐ 8 digit pad numbers
print sprintf("%08d", 1234); // 00001234
?>
Because numbers in PHP canโt have leading zeros naturally, you need to format the numbers using the sprintf()
function.
You can also format negative numbers with leading zeros:
<?php
// ๐ 6 digit pad numbers
print sprintf("%06d", -123); // -00123
print PHP_EOL;
print sprintf("%06d", -654); // -00654
?>
Alternatively, you can also use the str_pad()
function to pad numbers with leading zeros.
But this function canโt be used to pad negative numbers as shown below:
<?php
// ๐ 6 digit pad numbers
print str_pad(1234, 6, 0, STR_PAD_LEFT); // 001234
print PHP_EOL;
// ๐ 8 digit pad numbers
print str_pad(1234, 8, 0, STR_PAD_LEFT); // 00001234
print PHP_EOL;
// ๐ negative number has wrong format
print str_pad(-1234, 6, 0, STR_PAD_LEFT); // 0-1234
?>
Padding negative numbers with the str_pad()
function will produce an invalid format.
But if you never need to format negative numbers with leading zeros, then itโs fine to use the str_pad()
function.