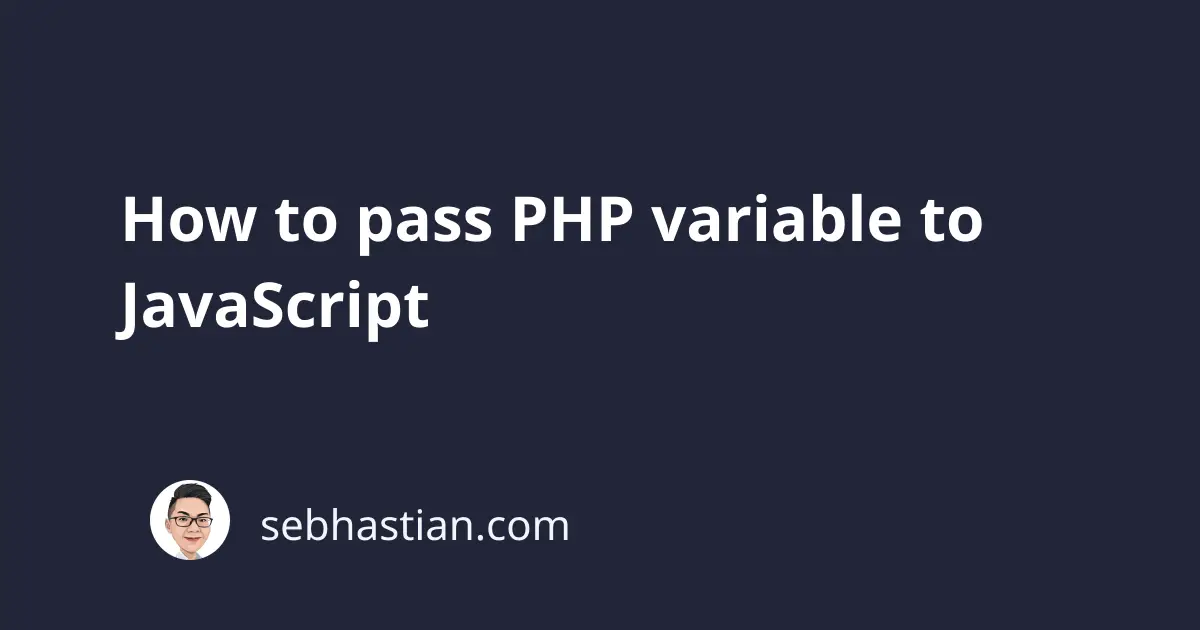
Before passing a variable from PHP to JavaScript, you need to understand the flow of events when you request a PHP page.
PHP is a server-side language. First, the PHP will execute your .php
file to generate the HTML.
After PHP process your code, it will be sent to the client’s browser.
Once the file is served to the browser, PHP can’t access the file anymore.
Since JavaScript runs in the browser, that means JavaScript is executed after PHP.
Knowing this process flow, there are three ways you can pass PHP variables to JavaScript:
Let’s start learning how to perform the three methods mentioned above.
Using echo to pass PHP variable to JavaScript
When you have a PHP variable on the same page as the JavaScript code, you can use echo
to pass the variable from PHP to JavaScript directly.
Suppose you have an index.php
file with the following content:
<?php
$name = "Nathan";
?>
<h1>Passing PHP variable to JavaScript</h1>
<h2 id="result"></h2>
<script>
const data = "<?php echo $name; ?>";
const result = document.getElementById("result");
result.innerText = data;
</script>
When you run the code above, the $name
variable value will be passed to the data
variable in JavaScript.
To show that is worked, the data
value is then assigned as the <h2>
tag content. The browser renders the following output:
With the echo
function, you can output a string by adding the quote symbols (single or double) before the <?php
tag above.
If you’re sending an int
value, you don’t need the quote symbols:
<?php
// 👇 sending a string
$name = "Nathan";
?>
<script>
const data = "<?php echo $name; ?>";
</script>
<?php
// 👇 sending an int
$age = 29;
?>
<script>
const data = <?php echo $age; ?>;
</script>
When you send a PHP array, you need to call the json_encode()
function before passing it to JavaScript:
<?php
$array = [1, 2, 3];
?>
<script>
const data = <?php echo json_encode($array); ?>;
</script>
And that’s how you pass a PHP variable to JavaScript by calling the echo
function.
Next, let’s see how to use the DOM to pass PHP variables to JavaScript.
Using DOM to pass PHP variables to JavaScript
Sometimes, you may need PHP variables after the user has done an action like clicking a button.
You may also have many PHP variables that you want to pass, and while echo
works to send PHP data to JavaScript, you may want a more clean solution.
Besides calling echo
function, you can also use the HTML DOM tag to store PHP data.
Here’s an example:
<div id="data-name" style="display: none;">
<?php
$name = "Nathan"; // this is where you get the data
echo htmlspecialchars($name); // put as the div content
?>
</div>
<div id="data-age" style="display: none;">
<?php
$age = 29; // this is where you get the data
echo htmlspecialchars($age); // put as the div content
?>
</div>
<div id="data-array" style="display: none;">
<?php
$array = [1, 2, 3]; // this is where you get the data
echo htmlspecialchars($array); // put as the div content
?>
</div>
By keeping your PHP data in the DOM tags as shown above, you can then retrieve the data using JavaScript as follows:
<script>
const name = document.getElementById("data-name").textContent;
const age = document.getElementById("data-age").textContent;
const arr = document.getElementById("data-array").textContent;
</script>
This method is similar to using echo
directly, but considered more clean since you echo
to the DOM instead of the JavaScript variables.
You can also choose when JavaScript should retrieve the PHP data. This is not the case when you echo
JavaScript variables.
Using fetch to get PHP data from JavaScript
When you want to separate the PHP template from your JavaScript code, then you can use the JavaScript Fetch API.
Fetch API is used to send an HTTP request, similar to AJAX and XMLHTTPRequest. This allows you to retrieve resources from anywhere on the Internet.
Fetch can be used to retrieve PHP data using JavaScript. Suppose you have a PHP file named data.php
with the following content:
<?php
/* put your process here:
* query the database
* get session, etc.
*/
$arr = [
"name" => "Nathan",
"age" => 27
];
// 👇 when done, echo the data
echo json_encode($arr);
Once you have the PHP file ready, create an index.php
file where you will fetch the data from.
Add a <script>
tag as shown below:
<div id="result"></div>
<script>
// 👇 URL to your data location
fetch("http://localhost:8080/data.php")
.then((res) => res.json())
.then((data) => {
const result = document.getElementById("result");
result.textContent = JSON.stringify(data);
});
</script>
The fetch()
function will send an HTTP request to the data.php
file.
Then, the json()
function will parse the response as a JSON object. Finally, the returned JSON object will be assigned as the content of the <div>
tag above.
The output will be as follows:
And that’s how you pass PHP variables or data to JavaScript using the fetch()
function.
Unlike the other two methods, this method creates an HTTP request, so it will have a delay before the data is fetched.
It’s recommended to use Fetch API when your PHP data requires complex processing before passing it to JavaScript.
Conclusion
To pass PHP variables and data to JavaScript, you need to make the data accessible to JavaScript.
You can echo
the data directly to JavaScript variable, or you can keep the data in the DOM before retrieving it using JavaScript Document
object.
For data with complex processing, you can create a separate file that processes your PHP data. Any part of your application that requires the data can send an HTTP request to fetch the data.
Finally, you need to treat your PHP data to make it accessible as JavaScript value:
- For a
string
, you need to add quotes - For an
int
ornumber
, you don’t need to add quotes - For an array or object, call the
json_encode()
function before passing to JavaScript
Now you’ve learned how to pass PHP variables to JavaScript. Nice work! 🤘