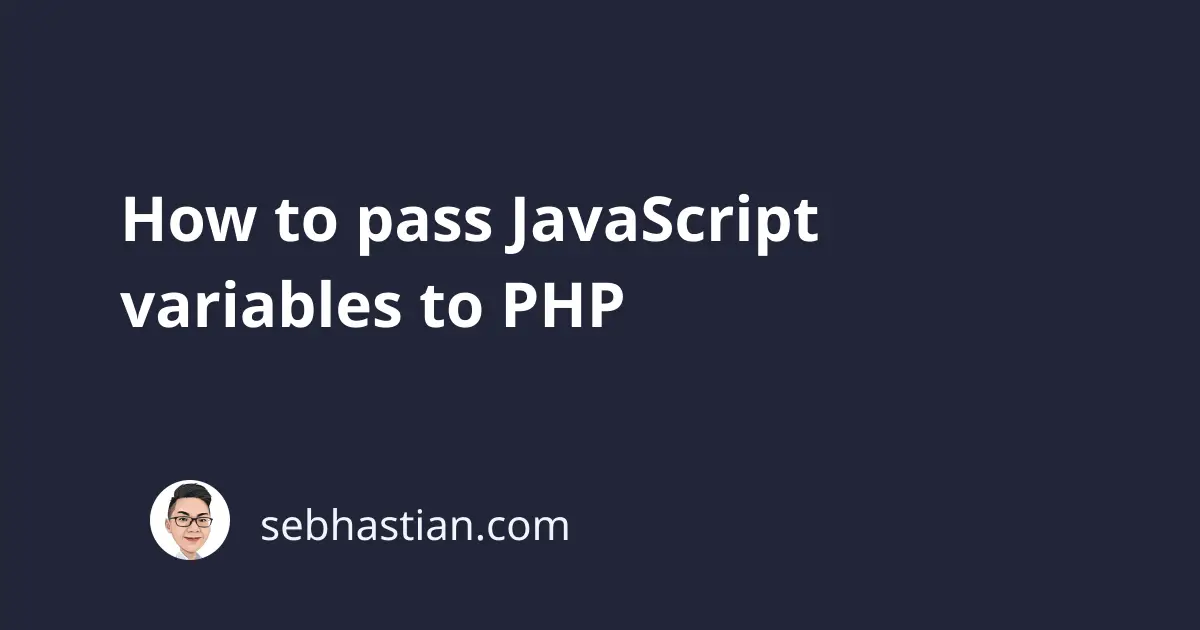
Sometimes, you might want to pass JavaScript variable values to your PHP code.
Before we get to the answer, please note that it is not possible to directly pass variables from JavaScript to PHP.
This is because PHP code is executed on the server, while JavaScript code is executed in the browser.
By the time JavaScript code is executed by the browser, PHP code has already finished running on the server.
However, there are ways to work around this limitation and pass data from JavaScript to PHP:
This article will help you learn the above solutions.
Use AJAX to Pass Data from JavaScript to PHP
AJAX (Asynchronous JavaScript and XML) is a technique for sending and receiving data from a server asynchronously, without having to refresh the page.
This makes it possible to update part of a web page without reloading the whole page. Itβs commonly used for things like form validation and dynamic content updates.
In JavaScript, AJAX is done using the XMLHttpRequest
object.
The code below shows how to use the XMLHttpRequest
object to send the data to your PHP script:
function sendData() {
var data = {
name: "Nathan",
email: "[email protected]",
};
var xhr = new XMLHttpRequest();
//π set the PHP page you want to send data to
xhr.open("POST", "index.php", true);
xhr.setRequestHeader("Content-Type", "application/json");
//π what to do when you receive a response
xhr.onreadystatechange = function () {
if (xhr.readyState == XMLHttpRequest.DONE) {
alert(xhr.responseText);
}
};
//π send the data
xhr.send(JSON.stringify(data));
}
In this example, we have defined a sendData()
function that contains the data we want to pass to PHP, along with the XMLHttpRequest
that will be executed to send the data.
This data is represented as a JavaScript object, but it could also be an array or a string.
The XMLHttpRequest
object is then used to send the data to the index.php
file on the server using an HTTP POST
request.
In your index.php
file, you can access the data that was sent from JavaScript with the following code:
<?php
$data = json_decode(file_get_contents("php://input"), true);
echo "Hello, " . $data["name"] . "!";
echo PHP_EOL;
echo "Your email address is " . $data["email"];
In the above code, the json_decode()
function converts the JSON-encoded data that was sent from JavaScript into a PHP array.
You can then access the individual elements of the array and use them in your PHP code.
When you run the sendData()
function, the PHP code will be executed and a response will be given as shown below:
You can run the sendData()
function by creating a <button>
HTML element as follows:
<button onClick="sendData()">Send Data</button>
<!-- <script> element here.. -->
You can change the alert()
function called in the xhr.onreadystatechange
function to fit your requirements.
Use fetch API to send HTTP request
The fetch
API is a modern alternative to XMLHttpRequest
that you can use to pass data from JavaScript to PHP.
Here is an example of how you might use the fetch
API to pass data from JavaScript to PHP:
<button onClick="sendData()">Send Data</button>
<script>
function sendData() {
var data = {
name: "Nathan",
email: "[email protected]",
};
//π call the fetch function
fetch("index.php", {
method: "POST",
body: JSON.stringify(data),
headers: {
"Content-Type": "application/json",
},
})
//π receive the response
.then((response) => response.text())
.then((data) => alert(data));
}
</script>
The code above does the same thing as the XMLHttpRequest
code.
The fetch
API is a more modern and flexible way to send data from JavaScript to PHP. It has the added advantage of being able to handle asynchronous requests.
However, it is not supported by all browsers, so you may need to use a polyfill if you want to support older browsers.
Use a Form to Pass Data from JavaScript to PHP
Another way to pass data from JavaScript to PHP is to use an HTML form.
When a user submits a form, the data is sent to the server as an HTTP request. This data can be accessed by the PHP script that processes the form submission.
To use a form to pass data from JavaScript to PHP, you will need to create a form on your web page and add a submit button.
Then, you can use JavaScript to populate the form fields with the data you want to pass to PHP, and trigger the form submission when the user clicks the submit button.
Consider the example below:
<form action="index.php" method="POST">
<input type="text" name="name" value="" id="name-field" />
<input type="email" name="email" value="" id="email-field" />
<input type="submit" value="Submit" id="submit-button" />
</form>
<button onClick="sendData()">Send Data</button>
<script>
function sendData() {
//π create data object
var data = {
name: "Nathan",
email: "[email protected]",
};
//π populate the form fields with the data
document.getElementById("name-field").value = data.name;
document.getElementById("email-field").value = data.email;
//π submit the form
document.getElementById("submit-button").click();
}
</script>
In this example, we have created an HTML form with two hidden input
fields and a submit button.
The formβs action
attribute specifies the PHP script that will process the form submission, and the method
attribute specifies that the data should be sent using an HTTP POST
request.
In the JavaScript code, we have defined a sendData
function that contains the data we want to pass to PHP.
This data is represented as a JavaScript object, but it could also be an array or a string.
We then use the document.getElementById()
function to get references to the form fields and the submit button.
Finally, we use the click()
method to trigger the form submission.
When the user clicks the submit button, the form will be submitted to the index.php
file on the server, and the data will be sent as part of the HTTP request.
In your PHP script, you can access the data that was sent from JavaScript using the $_POST
variable, just as we did in the previous example.
Note that since a form submission will refresh the current page, you may not see the response from PHP.
This solution is recommended only when you need to send data to the same page.
You can then write the code that will output the response as follows:
<form
action="<?php echo htmlspecialchars($_SERVER['PHP_SELF']); ?>"
method="POST">
<input type="text" name="name" value="" id="name-field" />
<input type="email" name="email" value="" id="email-field" />
<input type="submit" value="Submit" id="submit-button" />
</form>
<button onClick="sendData()">Send Data</button>
<?php
//π output response with php
if (isset($_POST["name"])) {
echo "<p>Hello, {$_POST["name"]}!</p>";
}
if (isset($_POST["email"])) {
echo "<p>Your email address is: {$_POST["email"]}</p>";
}
?>
<script>
function sendData() {
var data = {
name: "Nathan",
email: "[email protected]",
};
document.getElementById("name-field").value = data.name;
document.getElementById("email-field").value = data.email;
document.getElementById("submit-button").click();
}
</script>
The action
attribute of the form above will submit the form to the current page.
Using a form requires you to write the HTML element on your page, then use JavaScript to manipulate the form.
You can submit the form using JavaScript or do it manually, but the output will not be shown on the page if you are sending data to another page.
Thatβs why this solution is effective only when you send data to the current page.
Conclusion
To pass variables or data from JavaScript to PHP, you can use the AJAX implementation in JavaScript (which is XMLHttpRequest
) or the fetch
API.
If you need to send data to the current page, then you can also use an HTML form to send the data.
You are free to use the solutions that fit your project requirements.
Now youβve learned how to pass JavaScript variables to PHP. Nice work!