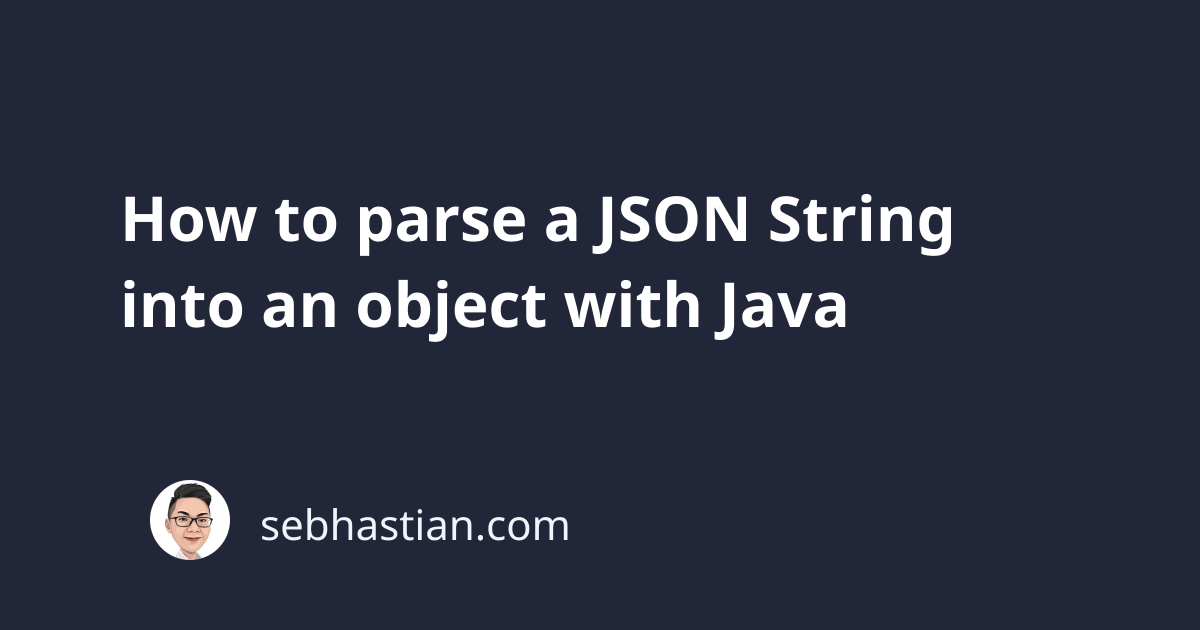
The JSON format is one of the most used data format for storing and exchanging information using computers.
Java has three popular libraries available to help you parse a string as a JSON object:
Although you can parse a JSON string as a JSON object without these libraries, it’s generally not recommended unless you are prepared to put in the required effort.
Without using third-party libraries, you need to implement the JSON parser specification according to the ECMA International specification that you can find here.
You need to test the parser library you’ve created and then be prepared to maintain and improve that library. You also need to write code that can handle the wrong JSON string format well or you might produce the wrong output.
In short, it’s better to use a tried-and-tested JSON library for Java that has been developed by many developers and is mature enough to handle many use cases.
This tutorial will help you learn how to parse JSON strings into objects and vice versa using the three libraries mentioned above.
Let’s start with the Gson library.
Parse JSON in Java using the Gson library
The Gson library is a Java library used to convert Java objects into their JSON representation and vice versa.
One notable feature of the Gson library is the full support for Java generics.
Once you add the library as a dependency to your Java project, you can use the fromJson()
method to convert a string into a Java object.
For example, suppose you have a Java class named Person
with the following definitions:
class Person {
String name;
int age;
boolean hasId;
}
You can parse a string as an instance of the Person
class as follows:
import com.google.gson.Gson;
String jsonStr = """
{
"name":"Nathan",
"age":22,
"hasId":"true"
}""";
Gson g = new Gson();
Person me = g.fromJson(jsonStr, Person.class);
System.out.println(me.name); // Nathan
System.out.println(me.age); // 22
System.out.println(me.hasId); // true
You can convert the object back into a String
with the toJson()
method of the Gson library as shown below:
String newStr = g.toJson(me);
System.out.println(newStr);
// Output:
// {"name":"Nathan","age":22,"hasId":true}
And that’s how easy it is to parse a JSON string into a Java object using Gson.
You can find more detailed information about the library on the Gson Github page
Parse JSON in Java using the Jackson library
The Jackson library is a data-processing tool for Java that can process not only JSON data format, but also others like CSV, TOML, YAML, or XML.
Still, Jackson became popular among Java and Android developers because of its ability to parse streaming JSON data.
To parse a JSON string into a Java object with Jackson, you need to create a class
with setters and getters for its fields as shown below:
class Person {
String name;
int age;
boolean hasId;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public boolean isHasId() {
return hasId;
}
public void setHasId(boolean hasId) {
this.hasId = hasId;
}
}
Without the setters and getters, Jackson will have no access to the Person class fields
The code below shows how to parse a string into a JSON object using Jackson:
import com.fasterxml.jackson.databind.ObjectMapper;
ObjectMapper mapper = new ObjectMapper();
String jsonStr = """
{
"name":"Nathan",
"age":22,
"hasId":true
}""";
try {
Person me = mapper.readValue(jsonStr, Person.class);
System.out.println(me.name); // Nathan
System.out.println(me.age); // 22
System.out.println(me.hasId); // true
} catch (IOException e) {
e.printStackTrace();
}
First, you need to create an instance of the ObjectMapper
class from Jackson. The instance is named mapper
in the above code.
Next, you need to call the readValue()
method from the mapper
instance, passing the String
variable as the first argument and the Java blueprint class
as the second argument.
To parse a Java object into a JSON string, you need to call the writeValueAsString()
method from the mapper
instance as follows:
String newStr = mapper.writeValueAsString(me);
System.out.println(newStr);
// {"name":"Nathan","age":22,"hasId":true}
And that’s how you can parse a JSON string as a Java object using the Jackson library.
For advanced use and other Jackson add-on libraries, you can visit the Jackson Github page.
Parse JSON in Java using the JSON-java library
The JSON-java library is a lightweight Java library that can be used to parse a JSON string into a Java object and vice versa.
The library is doesn’t require you to pass a Java class
as the JSON object blueprint.
Instead of your defined class
instance, the object will be of the JSONObject
instance.
The code below shows how you can use the JSON-java library to create an object from a JSON string:
import org.json.JSONObject;
String jsonStr = """
{
"name":"Nathan",
"age":22,
"hasId":true
}""";
JSONObject jsonObj = new JSONObject(jsonStr);
System.out.println(jsonObj.getString("name")); // Nathan
System.out.println(jsonObj.getInt("age")); // 22
System.out.println(jsonObj.getBoolean("hasId")); // true
To convert the JSONObject
back into a String
, you can call the toString()
method from the instance as follows:
String newStr = jsonObj.toString();
System.out.println(newStr);
// Output:
// {"hasId":true,"name":"Nathan","age":22}
The JSONObject
implementation is similar to the Java HashMap
class, so the order of the key-value pairs won’t be preserved.
This is why the string output of the toString()
method above is not in the same order as the original jsonStr
variable.
And that’s how you can parse a JSON string using the JSON-java library.
For more information, you can visit the JSON-java Github page.
Now you’ve learned how to parse JSON string using Java with the three most popular JSON libraries. Well done! 👍