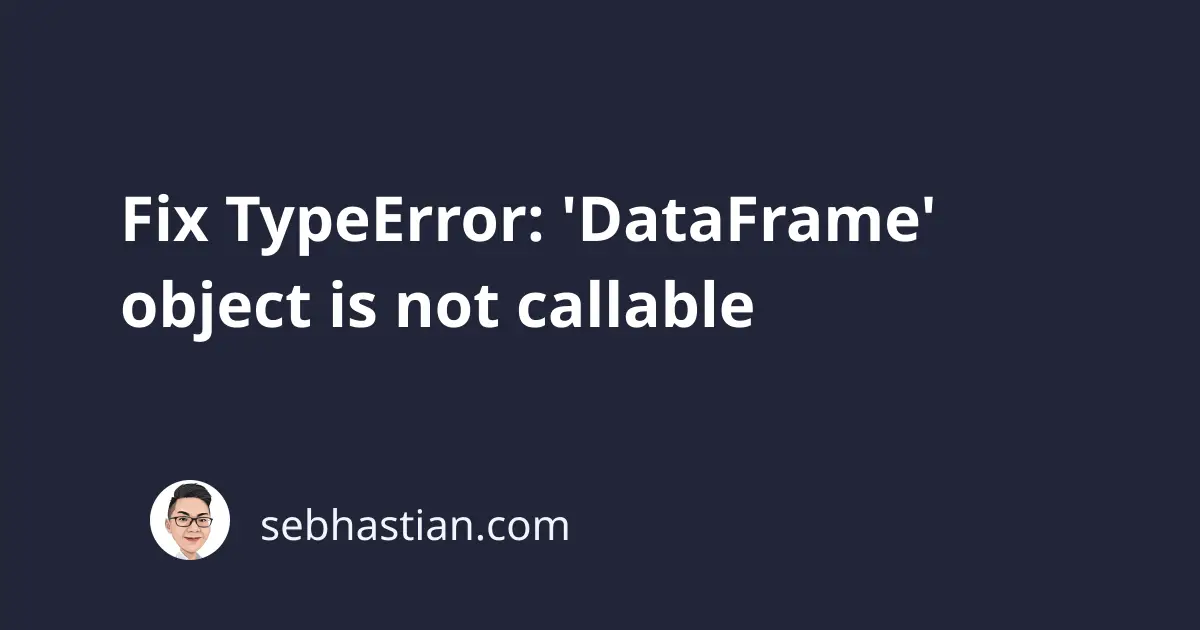
If you work with the Python library pandas
, you might get the following error:
TypeError: 'DataFrame' object is not callable
This error occurs when you use parentheses ()
to access a pandas DataFrame column when you should use the square brackets []
.
For example, suppose you have a DataFrame object as follows:
import pandas as pd
df = pd.DataFrame({
"name": ["Nathan", "Jane", "John", "Lisa"],
"age": [29, 26, 28, 22],
"gold": [5000, 2200, 3200, 1000]
})
# Show the DataFrame
print(df)
Output:
name age gold
0 Nathan 29 5000
1 Jane 26 2200
2 John 28 3200
3 Lisa 22 1000
Next, want to display only the values in the “gold” column, so you specify it in the print()
function:
print( df('gold') )
But you’ve wrongly used the round brackets ()
when accessing the column! This causes Python to respond with:
Traceback (most recent call last):
File "main.py", line 10, in <module>
print( df('gold') )
^^^^^^^^^^
TypeError: 'DataFrame' object is not callable
In Python, square brackets are used to call a function, and it’s no different when you’re using the pandas library.
A DataFrame
is an object, so you receive an error when calling it like a function.
How to fix this error
To fix this error, you just need to replace the round brackets ()
with square brackets []
when accessing a DataFrame column.
Here’s an example:
print( df['gold'] )
Output:
0 5000
1 2200
2 3200
3 1000
Name: gold, dtype: int64
Beside using square brackets []
, you can also use the dot notation .
to access a DataFrame column:
print( df.gold ) # ✅
Now the error is resolved!
When using pandas, you can use the round brackets ()
when you need to call a function.
For example, suppose you need to sum the values in the “gold” column. Here’s how you call the sum()
function:
total_gold = df['gold'].sum()
# or
total_gold = df.gold.sum()
print(total_gold) # 11400
I hope this tutorial gives you a clear understanding of why the error occurs and how to fix it. See you again! 👋