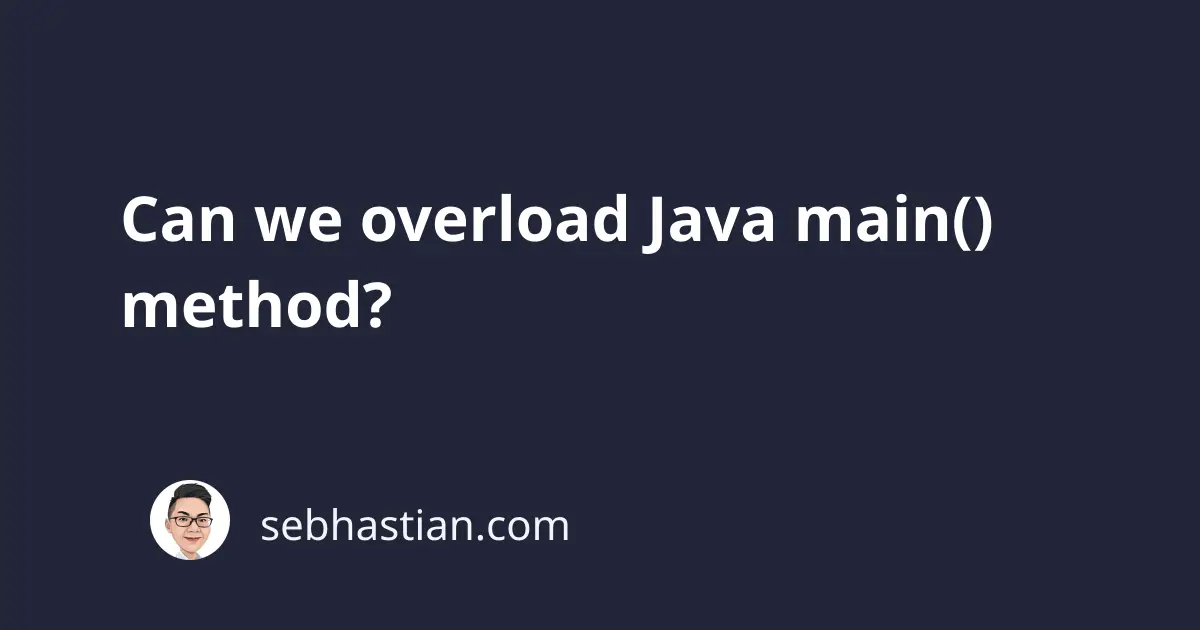
In Java, the main()
method of an entry class can be overloaded by defining multiple main()
methods in the class.
For example, suppose you have a Main
class with the following code:
public class Main {
public static void main(String[] args) {
System.out.println("The main method is called");
}
}
You can overload the main()
method above as shown below:
public class Main {
public static void main(String[] args) {
System.out.println("The main method is called");
}
// Overload 1
public static void main(int num) {
System.out.println("Overloaded main method with an int parameter");
}
// Overload 2
public static void main(Boolean bool) {
System.out.println("Overloaded main method with a Boolean parameter");
}
}
However, when you run the Main
class above using Java, the Java Virtual Machine (JVM) will always call the main()
method with the String[]
parameter.
The reason for this is that JVM will always pass an array of String
into the main()
method.
Even when you pass an int
or a Boolean
argument from the command line, JVM will pass them as an array of String
to the main()
method:
$ java Main.java
The main method is called
$ java Main.java 99
The main method is called
$ java Main.java true
The main method is called
If you want to call the overloaded main()
method(s) then you need to call it from the original main method like this:
public class Main {
public static void main(String[] args) {
System.out.println("The main method is called");
main(99);
}
// Overload 1
public static void main(int num) {
System.out.println("Overloaded main method with an int parameter");
}
}
Now when you run the Main
class from the command line, the output should be as shown below:
$ java Main.java
The main method is called
Overloaded main method with an int parameter
But then again, it’s better to just give the main(int num)
method another name instead.
To summarize, you can overload the main()
method in Java, but there’s almost no benefit in doing that. 😄