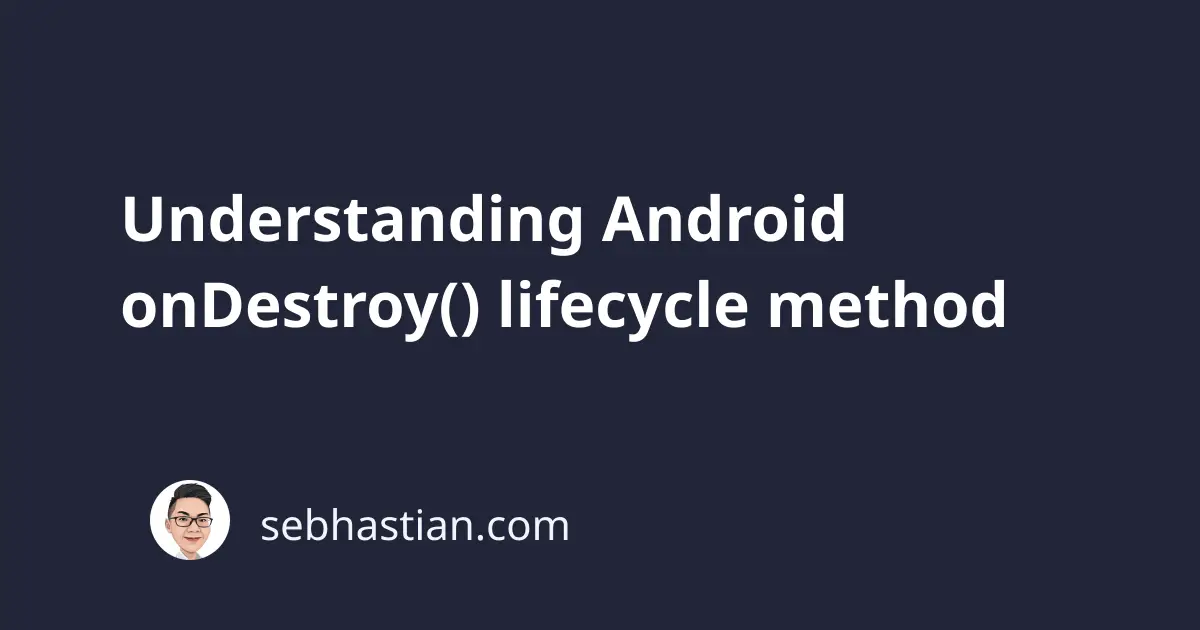
The onDestroy()
method is a method of the Activity
class that’s called right before the application is about to be removed from the memory (RAM).
The onDestroy()
method is executed right before Android shuts down the Activity
.
The image below is from Android documentation:
Usually, Android will release all resources used by the Activity
here before removing your application from the RAM.
To see an example of the method in action, you can define the onDestroy()
method in the MainActivity
class of your application.
The class code is as shown below:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@Override
protected void onDestroy() {
super.onDestroy();
Log.e("METAPX","onDestroy is CALLED");
}
}
In the above code, the onDestroy()
method simply creates a log that will be printed in Android Studio’s Logcat window.
It’s the super.onDestroy()
that will release all resources used by the Activity
class.
Here’s an example of the method in action:
The onDestroy()
method is commonly called when these two conditions occur:
- When you close the application from the Overview window as shown above
- When you run an application that uses the device resources intensively
When you open an application that consumes more memory than what’s available, Android may close other apps that run in the background in an attempt to give enough memory for the application you’re currently running.
Android applications commonly stay in the background when you press the back or home button. The app consumes a very small amount of memory so that you can resume where you left off when you open the app again.
But when a new application is opened that requires a large amount of memory, then Android can kill the applications to free up the device’s memory.
The onDestroy()
method is called to let your application finalize and clean its own process from your device.
For example, when you have a social media application, you can send a signal to your server to know when a user closes the application:
@Override
protected void onDestroy() {
super.onDestroy();
Client.offline(); // send signal to the server
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
The signal can then be forwarded to the user’s friends so they are notified when the user is offline.
Please note that you should not save any data inside the onDestroy()
method.
Instead, you should save persistent data in the onPause()
method as written in Android documentation
And that’s how the onDestroy()
method works in Android. 👍