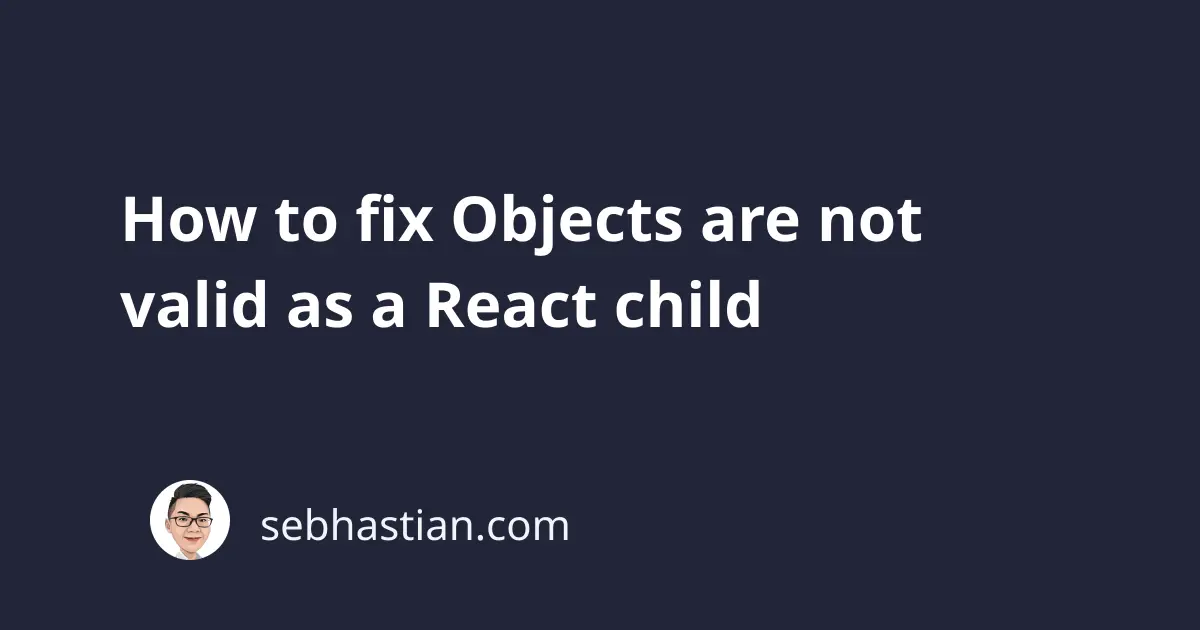
One error that you might encounter when working with React is:
Uncaught Error: Objects are not valid as a React child
This error occurs when you use a vanilla JavaScript object inside JSX directly.
This tutorial will show you examples that cause this error and how to fix it.
1. Embedding an object in JSX causes this error
To understand why this error occurs, suppose you have a vanilla JavaScript object that you pass into a component as follows:
const userData = { name: "John", age: 21 };
function App() {
return <div>{userData}</div>;
}
The above code embeds the userData
object inside the App
component’s JSX syntax.
React doesn’t know what to render when provided with an object. Do you want to render the whole object as a string? Or do you want to render the values only?
This is why instead of assuming something, React throws an error:
Uncaught Error: Objects are not valid as a React child
(found: object with keys {name, age}).
React can only render primitive types like a string, a number, or a boolean.
To fix this error, you need to add more details in your JSX code on how to render the object you provided.
For example, you might want to render the object values, so you can improve the code like this:
const userData = { name: "John", age: 21 };
function App() {
return (
<div>
{userData.name}: {userData.age}
</div>
);
}
The above code renders John: 21
to the screen.
As an alternative, you might want to render the key and the value in multiple div tags.
You need to use the entries()
and map()
functions as shown below:
const userData = { name: "John", age: 21 };
function App() {
return Object.entries(userData).map(([key, value]) => (
<div key={key}>
{key}: {value}
</div>
));
}
The rendered App
component would be similar to this:
<div id="root">
<div>name: John</div>
<div>age: 21</div>
</div>
If you want to render the whole object to the screen, you need to use JSON.stringify
to convert the object to a string first.
Since a string is a primitive type, React will render it as-is without any modifications:
const userData = { name: "John", age: 21 };
function App() {
return <div>{JSON.stringify(userData)}</div>
}
React will render {"name":"John","age":21}
to the screen with the above code.
2. Rendering an array of objects
The same error occurs when you try to render an array of objects without telling React how to render it.
Suppose you pass an array of objects to React component as follows:
const users = [
{ name: "John", age: 21 },
{ name: "Jane", age: 20 },
{ name: "Lisa", age: 24 },
];
function App() {
return <div>{users}</div>;
}
React again won’t know how to handle the given users
array.
To fix this error, you can use the map()
function and render the array values as shown below:
const users = [
{ name: "John", age: 21 },
{ name: "Jane", age: 20 },
{ name: "Lisa", age: 24 },
];
function App() {
return (
<div>
{users.map(user => (
<p>
{user.name}: {user.age}
</p>
))}
</div>
);
}
Output:
<div>
<p>John: 21</p>
<p>Jane: 20</p>
<p>Lisa: 24</p>
</div>
As you can see, the map()
function comes in handy when you need to render an array of objects.
3. Don’t convert your primitive type into an object
This error also occurs when you mistakenly convert a primitive type into an object using curly braces.
Suppose you have a name
variable that you want to render as follows:
const name = "John"
function App() {
return <div> {{name}} </div>
}
The error happens because there are two sets of curly braces {{}}
surrounding the name
variable. These curly braces convert the name
variable into an object as {name: 'John'}
.
You need to fix the code by using only one set of curly braces:
const name = "John"
function App() {
return <div> {name} </div>
}
When you run the application again, notice the error doesn’t appear.
Conclusion
React shows “Objects are not valid as a React child” error when you attempt to pass a JavaScript object inside JSX directly.
As React doesn’t know how you want the object to be presented on the screen, it chooses to show an error and render nothing instead. To fix this error, you need to tell React how to render the object by adding more code to the component.
This tutorial has shown you three common causes for this error and the solution for each error.
I hope this tutorial is helpful. Happy coding! 🙌