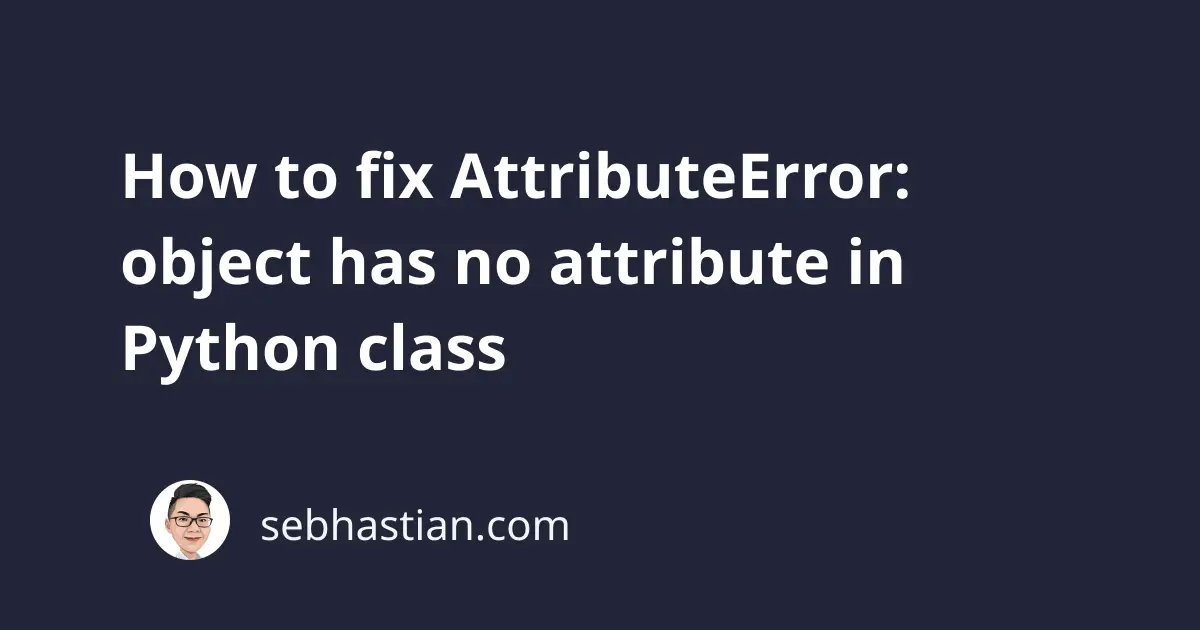
One error that you might encounter when working with Python classes is:
AttributeError: 'X' object has no attribute 'Y'
This error usually occurs when you call a method or an attribute of an object. There are two possible reasons for this error:
- The method or attribute doesn’t exist in the class.
- The method or attribute isn’t a member of the class.
The following tutorial shows how to fix this error in both cases.
1. The method or attribute doesn’t exist in the class
Let’s say you code a class named Human
with the following definitions:
class Human:
def __init__(self, name):
self.name = name
def walk(self):
print("Walking")
Next, you created an object from this class and called the eat()
method:
person = Human("John")
person.eat()
You receive an error because the eat()
method is not defined in the class:
Traceback (most recent call last):
File "main.py", line 10, in <module>
person.eat()
AttributeError: 'Human' object has no attribute 'eat'
To fix this you need to define the eat()
method inside the class
as follows:
class Human:
def __init__(self, name):
self.name = name
def walk(self):
print("Walking")
def eat(self):
print("Eating")
person = Human("John")
person.eat() # Eating
Now Python can run the eat()
method and you won’t receive the error.
The same goes for attributes you want the class to have. Suppose you want to get the age
attribute from the person
object:
person = Human("John")
print(person.age) # ❌
The call to person.age
as shown above will cause an error because the Human
class doesn’t have the age
attribute.
You need to add the attribute into the class:
class Human:
age = 22
def __init__(self, name):
self.name = name
def walk(self):
print("Walking")
person = Human("John")
print(person.age) # 22
With the attribute defined inside the class, you resolved this error.
2. The method or attribute isn’t a member of the class
Suppose you have a class with the following indentations in Python:
class Human:
def __init__(self, name):
self.name = name
def walk():
print("Walking")
Next, you created a Human
object and call the walk()
method as follows:
person = Human("John")
person.walk() # ❌
You’ll receive an error as follows:
Traceback (most recent call last):
File "main.py", line 9, in <module>
person.walk()
AttributeError: 'Human' object has no attribute 'walk'
This error occurs because the walk()
method is defined outside of the Human
class block.
How do I know? Because you didn’t add any indent before defining the walk()
method.
In Python, indentations matter because they indicate a block of code, like curly brackets {}
in Java or JavaScript.
When you write a member of the class, you need to indent each line according to the class structure you want to create.
The indentations must be consistent, meaning if you use a space, each indent must be a space. The following example uses one space for indentations:
class Human:
def __init__(self, name):
self.name = name
def walk(self):
print("Walking")
This one uses two spaces for indentations:
class Human:
def __init__(self, name):
self.name = name
def walk(self):
print("Walking")
And this uses four spaces for indentations:
class Human:
def __init__(self, name):
self.name = name
def walk(self):
print("Walking")
When you incorrectly indent a function, as in not giving any indent to the walk()
method, then that method is defined outside of the class:
class Human:
def __init__(self, name):
self.name = name
def walk():
print("Walking")
# Valid
walk() # ✅
# Invalid
person = Human("John")
person.walk() # ❌
You need to appropriately indent the method to make it a member of the class. The same goes when you’re defining attributes for the class:
class Human:
age = 22
def __init__(self, name):
self.name = name
def walk(self):
print("Walking")
# Valid
person = Human("John")
person.walk() # ✅
print(person.age) # ✅
You need to pay careful attention to the indentations in your code to fix the error.
I hope this tutorial is helpful. Have fun coding! 😉