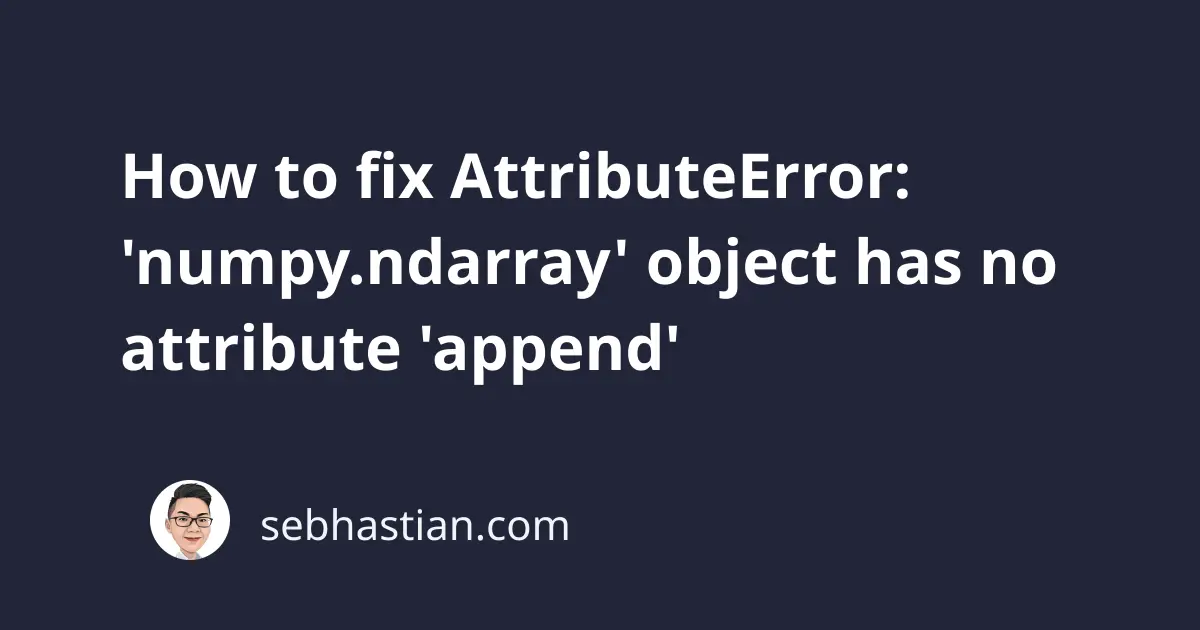
When running Python code that uses NumPy, you might encounter the following error:
AttributeError: 'numpy.ndarray' object has no attribute 'append'
This error occurs when you attempt to add an element to the NumPy array object using the append()
method.
This tutorial will show you an example that causes this error and how I fix it in practice.
How to reproduce this error
Suppose you have a NumPy array defined in your code as follows:
import numpy as np
arr = np.array([1, 2, 3])
Next, suppose you want to add a new element to the array. You called the append()
method on the array object as follows:
arr.append(4)
Output:
AttributeError: 'numpy.ndarray' object has no attribute 'append'
The call to append()
method causes an error because the NumPy array object doesn’t have the append()
method.
How to fix this error
To resolve this error, you need to use the numpy.append()
method instead of the ndarray.append()
method.
The numpy.append()
method accepts two parameters:
- The array you want to add new elements into
- The elements you want to add to the array
Here’s how to add new elements to the array:
import numpy as np
arr = np.array([1, 2, 3])
new_arr = np.append(arr, 4)
print("Original array:", arr)
print("Array after append:", new_arr)
Output:
Original array: [1 2 3]
Array after append: [1 2 3 4]
Here, the np.append()
method successfully assigned the new element 4 into the array.
Note that the np.append()
method doesn’t modify the original array. Instead, a new array is returned that has the new element appended.
When you need to add multiple elements to the array, you can specify an array of values as the second parameter:
import numpy as np
arr = np.array([1, 2, 3])
new_arr = np.append(arr, [4, 5, 6])
print("Original array:", arr)
print("Array after append:", new_arr)
Output:
Original array: [1 2 3]
Array after append: [1 2 3 4 5 6]
The np.append()
method is smart enough to append the values as part of the same array instead of creating a nested array.
Conclusion
The AttributeError: 'numpy.ndarray' object has no attribute 'append'
occurs in Python when you attempt to call the append()
method from the NumPy array object.
To resolve this error, you need to call the append()
method from numpy
directly.
I hope this tutorial is useful. Happy coding! 👍