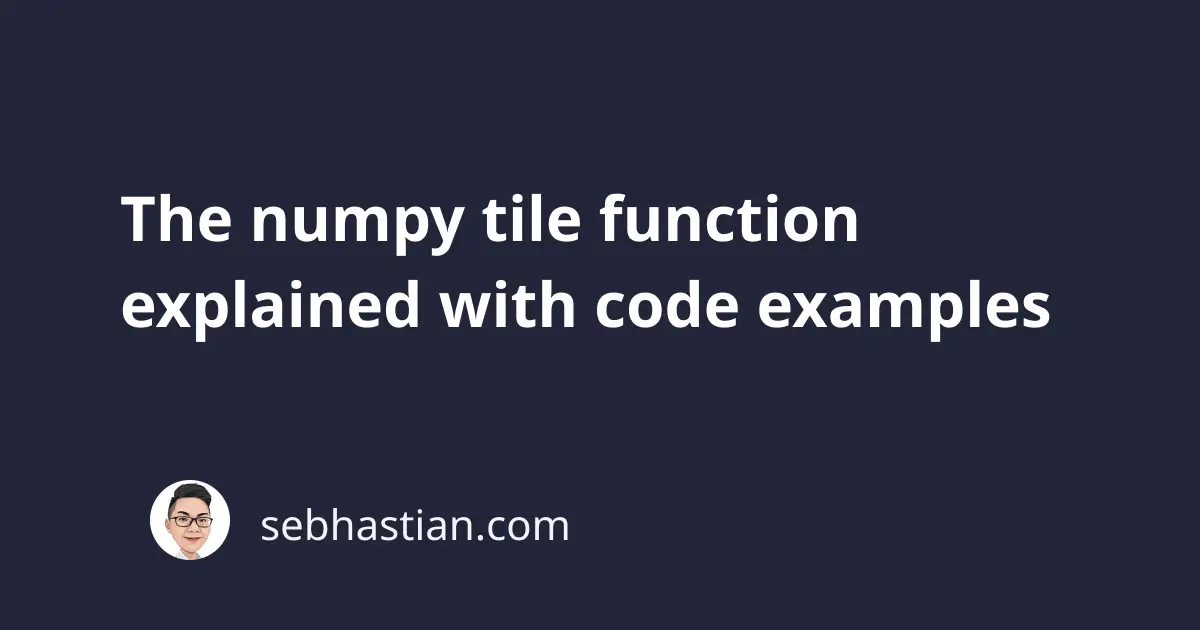
The numpy.tile()
function allows you to create a copy of an array where the array’s elements are repeated along each axis.
The syntax of the function is as follows:
numpy.tile(array, reps)
The function accepts two arguments:
- The
array
you want to copy - The
reps
number of repetitions you want the array elements to have.
Consider the following code example:
import numpy
array = [1, 2, 3]
# Set reps to 1
array_reps = numpy.tile(array, 1)
print(array_reps) # array([1, 2, 3])
# Change the reps to 2
array_reps = numpy.tile(array, 2)
print(array_reps) # array([1, 2, 3, 1, 2, 3])
The reps
parameter of the tile()
function accepts array-like numbers that will be used to repeat the elements of the array for each axis.
You can pass two numbers as the reps
argument as shown below:
array_reps = numpy.tile(array, (2, 2))
print(array_reps)
# array([[1, 2, 3, 1, 2, 3],
# [1, 2, 3, 1, 2, 3]])
You can even pass three numbers to make a three-dimensional array of frames, rows, and columns:
array_reps = numpy.tile(array, (2, 1, 1))
print(array_reps)
"""
array([[[1, 2, 3]],
[[1, 2, 3]]])
"""
And that’s how the numpy tile()
function works.