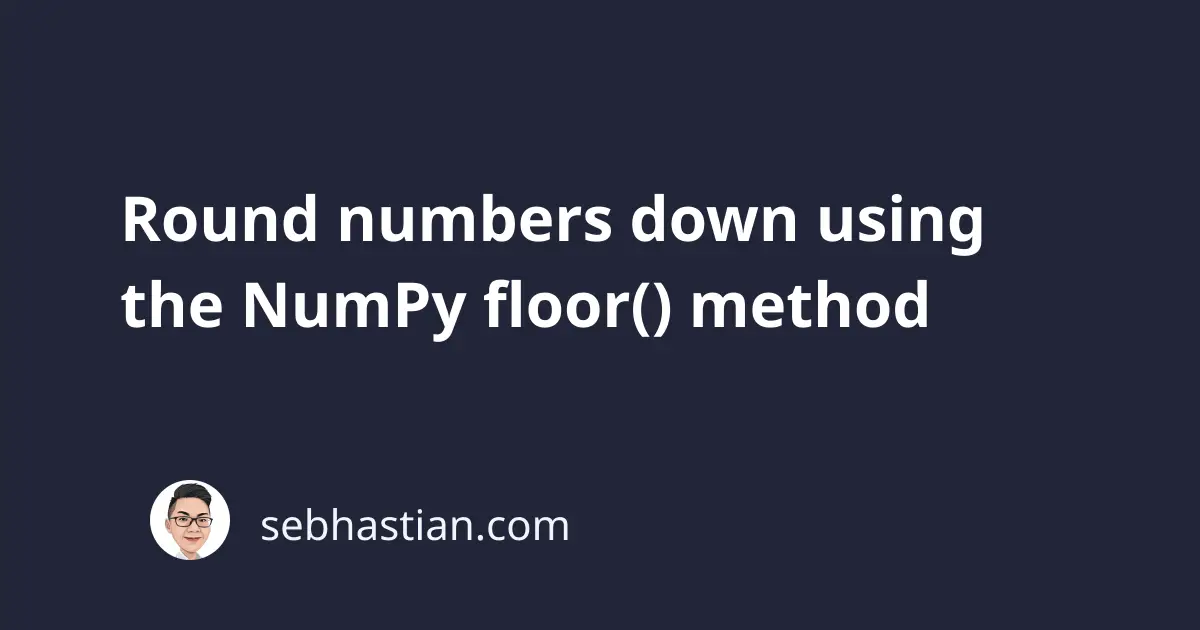
The NumPy floor()
method allows you to round a floating number down to the nearest integer number.
By default, the method will return a number or an array depending on the argument you pass into it:
import numpy as np
float_x = 5.5
whole_x = np.floor(float_x)
print(whole_x) # 5.0
print(np.floor(2.3314)) # 2.0
print(np.floor(-8.3314)) # -9.0
The floor method also works for both positive and negative numbers.
You can also pass an array of numbers into the floor()
method as shown below:
numbers = [1.873, 21.2232, 7.624]
print(numbers) # [1.873, 21.2232, 7.624]
floor_off_numbers = np.floor(numbers)
print(floor_off_numbers) # [ 1. 21. 7.]
When you pass a value that can’t be rounded down, the floor()
method will throw an error and stop the running program:
print(np.floor("c"))
"""
TypeError: ufunc 'floor' not supported for the input types,
and the inputs could not be safely coerced to any supported types
according to the casting rule ''safe''
"""
The same error above will be triggered when you pass an array with string types.
When you pass a bool
type as its argument, then the floor()
method will return the integer representation of the bool
value (True
returns 1.0
and False
returns 0.0
)
print(np.floor(True)) # 1.0
print(np.floor(False)) # 0.0
Finally, the NumPy floor()
method calculates the floor towards the lower value instead of towards zero.
In some computer programs, the floor value is calculated towards zero, where the negative numbers are actually raised to be nearer to zero:
floor(-3.4) = -3 # floor towards zero
floor(-5.2) = -6 # floor towards the lower value
The floor()
method calculates the floor towards the lower value. If you want to calculate the floor towards zero, you need to use the fix()
method.
Now you’ve learned how the NumPy floor()
method works. Good work! 👍