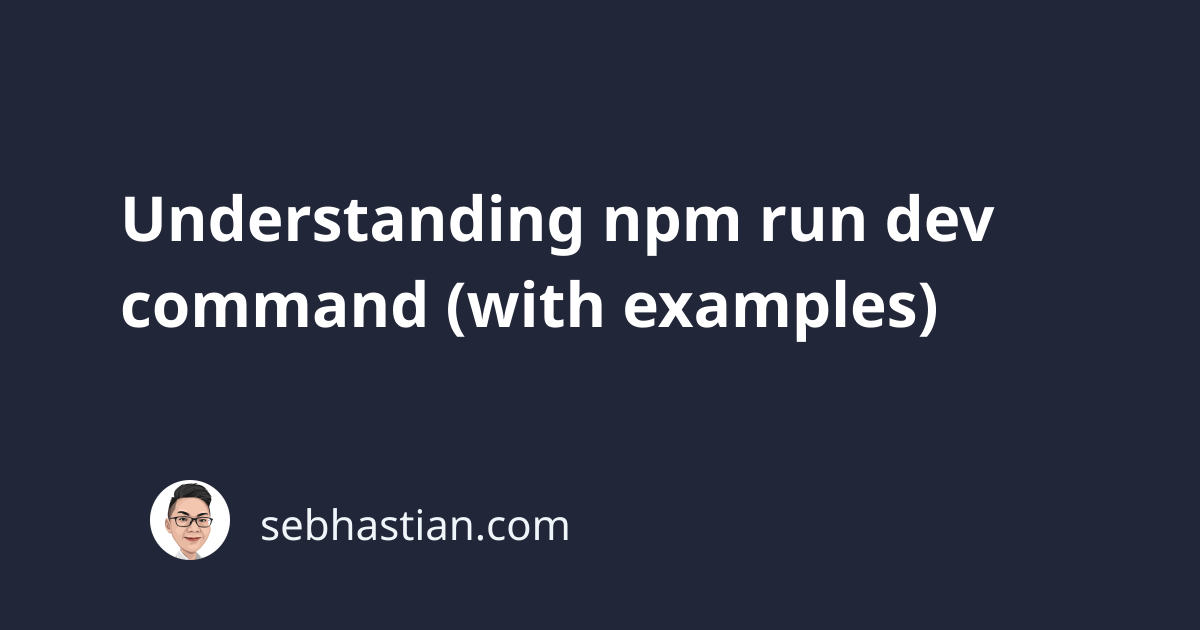
The npm run dev
command is a generic npm command that you can find in many modern web application projects.
This command is used to run the dev
script defined in the project’s package.json
file.
To know what is exactly being run by the command, first you need to open the package.json
file.
For example, a Next.js application has the following scripts
property defined:
{
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start",
"lint": "next lint"
}
}
The above example means that the npm run dev
command will run next dev
from the terminal.
That command will start a development server so that you can open the Next.js application from your browser.
As to how the next dev
command works, you need to look into the source code inside the node_modules/
folder. But that is beyond the scope of this tutorial.
In short, the npm run
command is part of the npm program used to run scripts. The name of the scripts themselves is user-defined inside the package.json
file.
This means that npm run dev
will run the dev
command, while npm run production
will run the production
command.
You can change the name of the script from dev
to development
as shown below:
{
"scripts": {
"development": "next dev",
"build": "next build",
"start": "next start",
"lint": "next lint"
}
}
This means that npm will execute the next dev
command when you call the npm run development
command.
When you call an undefined script, npm will throw an error saying missing script as shown below:
$ npm run prod
npm ERR! Missing script: "prod"
npm ERR!
npm ERR! To see a list of scripts, run:
npm ERR! npm run
To know the available commands for the project, you can open the package.json
and take a look.
Or when you only have access from the terminal, run the npm run
command as shown below:
$ npm run
Lifecycle scripts included in [email protected]:
start
next start
available via `npm run-script`:
dev
next dev
build
next build
lint
next lint
Let’s see another example of a project that used the npm run dev
command.
When you create a new Laravel project, you will see the following scripts
property in its package.json
file:
{
"scripts": {
"dev": "npm run development",
"development": "mix",
"watch": "mix watch",
"watch-poll": "mix watch -- --watch-options-poll=1000",
"hot": "mix watch --hot",
"prod": "npm run production",
"production": "mix --production"
}
}
Notice that both dev
and development
commands are defined in the JSON file above.
In Laravel’s case, the npm run dev
command is an alias for the npm run development
command.
The development
command is used to run the mix
module, which is used to compile the JavaScript and CSS files written for the Laravel application.
Conclusion
The npm run dev
command is used to run the dev
script defined inside a package.json
file.
Although the names for the scripts are arbitrary, the developer community has some conventions used for naming the script. Usually, the dev
or development
command is used for two things:
- To start a local development server
- Or to compile required assets without optimizing them.
Scripts like build
or production
usually relate to building the application for the production environment, where the JavaScript and CSS files are optimized for speed and performance.
You can see all available scripts by looking into the package.json
file content. Running the npm run
command can also be a good alternative when you are limited to the terminal access.
To learn what is actually being done by the command, you need to look into the documentation of your library (or framework)
I hope this tutorial has helped you to understand how the npm run dev
command works. 🙏