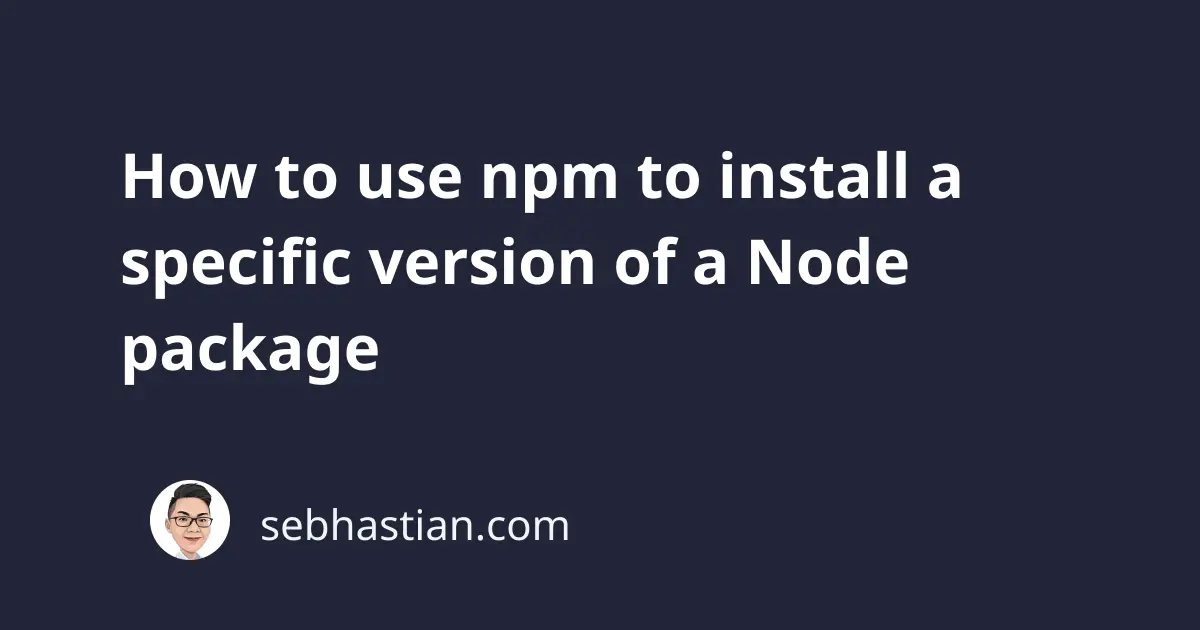
By default, npm will install the latest stable version of a package when you run the npm install
command.
This tutorial will walk you through the steps of using npm to install a specific version of a Node package.
How to install a specific version of npm package
To install a specific version of a Node package, you need to run this command:
npm install <package-name>@<version-number>
Replace <package-name>
with the name of your desired package, and <version-number>
with the version number of your package.
Since npm uses semantic versioning, a package version number is specified using the major.minor.patch
version format.
For example, suppose you want to install react
version 15.5.0
.
Here’s how you do it:
npm install [email protected]
# or shorten the command
npm i [email protected]
The command above will fetch the react
package with the exact version you stated using the @x.x.x
format.
You can also select the latest major version of a package by stating only the major version as follows:
npm install react@15
The command above will install react
version 15
with the latest minor and patch version, which is 15.7.0
.
You can also specify the major and minor versions but without the patch version:
npm install [email protected]
The latest patch of react
version 15.5
is 15.5.4
so that’s the version that will be installed.
Install a specific Node package version globally
Unless specified as a global install, npm will install the package in the current working directory.
To install a specific version of a package globally, just add the -g
option after the install
command:
npm install -g gulp@3
The command above will install version 3
of the gulp
package globally.
Use semantic versioning symbols (caret and tilde)
The caret (^) and tilde (~) symbols can be used when specifying your package versions in the package.json
file to ensure that the latest minor and patch versions are installed.
This is especially useful if you want to stay up to date with bug fixes and new features without having to update the package.json
file each time manually.
Let’s start with the caret (^) symbol. To use it, simply add it after the version number in your npm install
command.
For example, suppose you have the following dependencies specified:
{
"dependencies": {
"react": "^15.1.0"
}
}
When you run the npm install
command, npm will install the latest minor version (15.x) of react
package, which could be anything from 15.1 to 15.5 - but not version 16 or higher as this would be a major version update.
The tilde symbol (~) works similarly but applies to patch updates instead of minor ones.
You can specify the version in the dependencies section like this:
{
"dependencies": {
"express": "~4.16.1"
}
}
This will tell npm to install the most recent patch version (4.16.x) available for express
package, but never anything higher than 4.16 since this would be a minor version update.
View all available versions for install
You can also see all versions available for installation using the npm view <package-name> versions
command.
Here’s an example of seeing available gulp versions:
$ npm view gulp versions
[
'0.0.1', '0.0.2', '0.0.3', '0.0.4', '0.0.5',
'0.0.7', '0.0.8', '0.0.9', '0.1.0', '0.2.0',
'1.0.0', '1.1.0', '1.2.0', '1.2.1', '2.0.0',
'2.0.1', '2.1.0', '2.2.0', '2.3.0', '2.4.0',
'2.4.1', '2.6.0', '2.6.1', '2.7.0', '3.0.0',
'3.1.1', '3.1.2', '3.1.3', '3.1.4', '3.2.0',
'3.2.1', '3.2.2', '3.2.3', '3.2.4', '3.2.5',
'3.3.0', '3.3.1', '3.3.2', '3.3.4', '3.4.0',
'3.5.0', '3.5.1', '3.5.2', '3.5.5', '3.5.6',
'3.6.0', '3.6.1', '3.6.2', '3.7.0', '3.8.0',
'3.8.1', '3.8.2', '3.8.3', '3.8.4', '3.8.5',
'3.8.6', '3.8.7', '3.8.8', '3.8.9', '3.8.10',
'3.8.11', '3.9.0', '3.9.1', '4.0.0', '4.0.1',
'4.0.2'
]
You can define the specific version you want to install based on the output above.
If you only want to see the latest stable version, you can run the command below:
npm view <package-name> version
Here’s an example output:
$ npm view gulp version
4.0.2
You can use this information to see if you already have the latest version installed.
See the versions of installed packages
You can see the versions of Node packages you’ve already installed by running the following command:
npm list
# or shortened version:
npm ls
# For globally installed packages
npm list --global
# or
npm ls -g
For example, here’s the response when I run the command from my terminal:
$ npm list
[email protected] /Users/nsebhastian/react-app
├── @testing-library/[email protected]
├── @testing-library/[email protected]
├── @testing-library/[email protected]
├── [email protected]
├── [email protected]
├── [email protected]
└── [email protected]
If you see the output of npm list
command differs from the versions specified in your package.json
file, then the versions shown in npm list
command are the truth.
To use the versions specified in your package.json
file, you need to run the npm install
command.
Installing the next version of Node packages
Additionally, you can also use @next
to install the next version of a package.
The next
version is commonly used to tag an experimental package build that’s not yet released to the public. This version is usually unstable and may contain bugs.
Since npm packages are open-sourced, the developers rely on the community to provide feedback and report bugs.
Generally, you can install the latest unstable version using the following command:
npm install react@next
# or
npm i react@next
Please keep in mind that you are not recommended to use the next
version for the production environment. This version is recommended only for testing purposes.
Conclusion
Installing a specific version of a package is useful when you need to roll back a package update because of breaking changes in the latest version.
Here are some commands that will help you:
- To install a specific version, use the
npm install <package-name>@<version-number>
command. - To view all available versions of a package, use the
npm view <package-name> versions
- To view the latest stable version, use the
npm view <package-name> version
- You can check the versions of installed packages using the
npm list
command - To install the latest unstable version, use the
npm install <package-name>@next
command
And now you’ve learned how to install a specific version of a Node package using npm.
I hope this tutorial is helpful. Cheers! 🍻