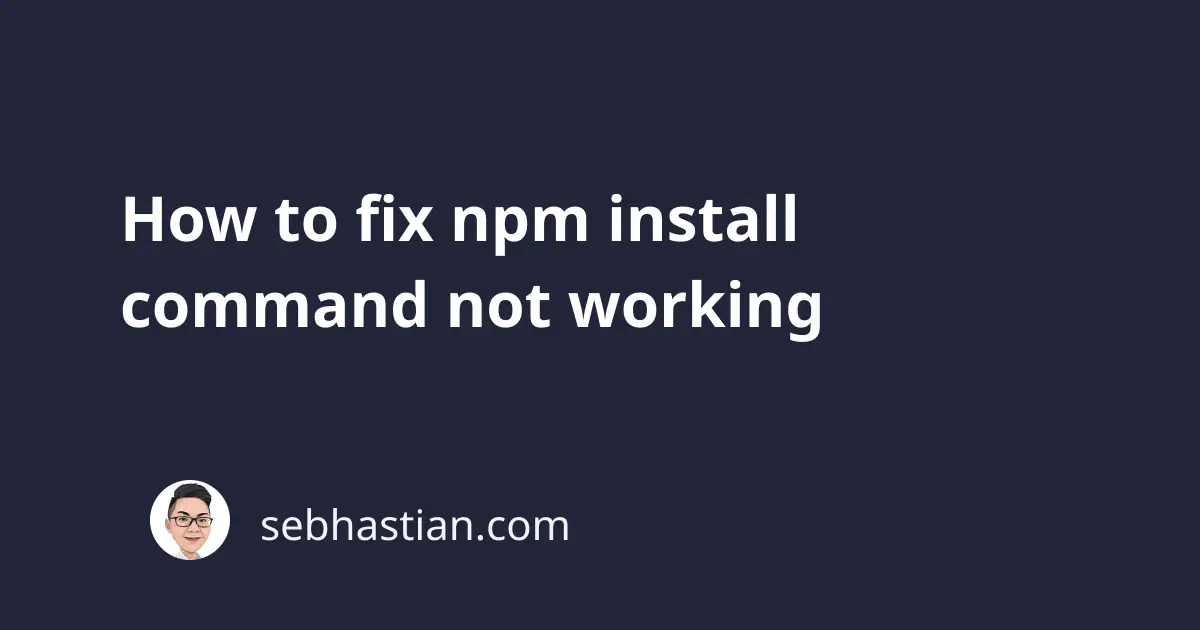
The npm install
command is used for installing JavaScript packages on your local computer.
When developing web projects, you may see issues that cause the npm install
command to fail.
You need to see the error message generated in the terminal for clues to resolve the error.
Some of the most common issues for npm install
not working are as follows:
- npm command not found
- No
package.json
file describing thedependencies
- Integrity check failed error
- For Windows: Python not found
Let’s see how you can resolve these errors next.
The npm command not found error
To run the npm install
command, you need to have npm installed on your computer
$ npm install
sh: command not found: npm
The error above happens when npm can’t be found under the PATH environment variable.
First, you need to make sure that npm is installed on your computer.
npm is bundled with Node.js server, which you can download from the nodejs.org website.
Once you downloaded and installed Node.js, open the terminal and run the npm -v
command.
You should see the version of npm installed on your computer as follows:
$ npm -v
8.11.0
Once you see the npm version, you should be able to run the npm install
command again.
For more details, visit the fixing npm command not found error article I’ve written previously.
ENOENT: No package.json file
The npm install
command looks for a package.json
file in order to find the packages it needs to install.
You need to make sure you have a package.json
file right in the current directory where you run the command.
You can run the ls -1
command as follows:
$ ls -1
index.js
package-lock.json
package.json
Once you see there’s a package.json
file in the output as shown above, then you can run the npm install
command.
For this reason, you should always run the npm install
command from the root directory of your project.
By the way, npm install
will install packages already listed under the dependencies
object of your package.json
file.
Suppose you have a package.json
file as shown below:
{
"name": "n-app",
"version": "1.0.0",
"description": "A Node application",
"dependencies": {
"jest": "^28.1.1",
"typescript": "^4.5.5",
"webpack": "^2.7.0"
}
}
Then npm install
will install jest, typescript, and webpack to the generated node_modules/
folder.
If you need to install a new package, then you need to specify the name like these examples:
# 👇 install react package
npm install react
# 👇 install react, react-dom, and axios packages
npm install react react-dom axios
You can install one or many packages with the npm install
command.
Integrity check failed error
When you have a package-lock.json
file in your project, then npm will check the integrity of the package you downloaded with the one specified in the lock file.
When the checksum of the package is different, then npm will stop the installation and throw the integrity check failed error.
The following is an example of the error:
npm ERR! code EINTEGRITY
npm ERR! Verification failed while extracting @my-package@^1.2.0:
npm ERR! Verification failed while extracting @my-package@^1.2.0:
npm ERR! Integrity check failed:
npm ERR! Wanted: sha512-lQ...HA==
npm ERR! Found: sha512-Mj...vg==
When this happens, you can fix the issue by verifying the cache:
npm cache verify
When the command is finished, run npm install
again.
If you still see the error, then delete your lock file and clean the npm cache.
Run the following commands from your project’s root directory:
# 👇 remove the lock file
rm package-lock.json
# 👇 remove the node_modules folder
rm -rf node_modules
# 👇 Clear the npm cache
npm cache clean --force
# 👇 run npm install again
npm install
That should fix the issue with the integrity check.
For Windows only: Python not found
When running npm install
on Windows, you may see an error that says Can’t find Python executable as shown below:
> node-gyp rebuild
/n-app>node "\..\node_modules\node-gyp\bin\node-gyp.js" rebuild
npm http 304 https://registry.npmjs.org/bcrypt
gyp ERR! configure error
gyp ERR! stack Error: Can't find Python executable "python",
you can set the PYTHON env variable.
The node-gyp
module is a Node.js tool for compiling native modules written in C and C++. It requires Python to run properly.
Instead of installing Python by yourself, you can install the Windows Build Tools which has Python included.
This is because Windows also requires Visual Studio build tools to make node-gyp
run successfully.
If you install only Python, then you may find that you need the build tools later.
Please note that the latest version of Node.js for Windows installer already includes the build tools by default.
But if you can’t update your Node.js version yet, then installing the build tools manually should help you fix this error.
Conclusion
The npm install
command may fail to work because of many reasons.
When the command doesn’t work, you need to check the output first and see what specific error you have.
Try a Google search to fix the issue. When you don’t see a solution, try posting a new question at Stack Overflow mentioning the things you have tried to fix the issue.
Good luck resolving the issue! 👍