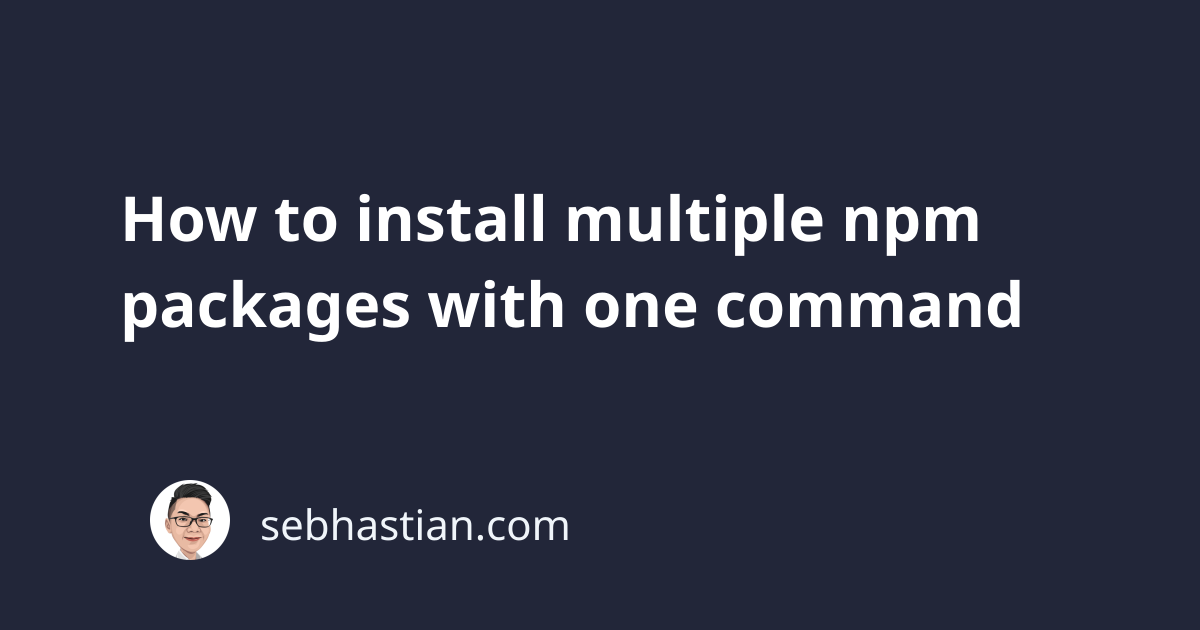
The npm install
command is used to install npm packages to a node_modules/
folder.
To install multiple npm packages at once, you need to specify the package names separated by a space between each name:
npm install vue express gulp
To install multiple packages globally, add the -g
or --global
flag to your command:
npm install -g vue express gulp
# or
npm install --global vue express gulp
You can also install a specific version of each package by adding the @version
keyword after the package names.
Here’s an example of installing specific versions of vue
, express
, and gulp
:
npm install [email protected] [email protected] [email protected]
npm will install the exact versions you defined in the example above.
Installing multiple packages using package.json
When you run the npm install
command without specifying any package name, then npm will look for an existing package.json
file in the current working directory.
npm will install all packages listed as dependencies of the project. This includes dependencies
, devDependencies
, peerDependencies
, optionalDependencies
, and bundleDependencies
property defined in your package.json
file.
Suppose you have the following package.json
file:
{
"dependencies": {
"express": "^4.8.4",
"gulp": "^3.9.0",
"vue": "^2.6.14"
},
"devDependencies": {
"jest": "^28.1.0",
"mocha": "^10.0.0"
},
"peerDependencies": {
"cors": "^2.8.5"
}
}
Then running npm install
will install all packages in the node_modules/
folder.
To speed up the build for the production environment, you can also opt to not install packages listed as devDependencies
by using the --production
flag:
npm install --production
The command above will ignore the devDependencies
property of your application.
Uninstall multiple packages with npm
To uninstall multiple packages using npm, use the npm uninstall
command followed by the package names:
npm uninstall vue express gulp
To uninstall packages in the global installation, add the -g
or --global
flag to the command:
npm uninstall -g vue express gulp
# or
npm uninstall --global vue express gulp
Now you’ve learned how to install multiple packages using npm.
You’ve also learned how to uninstall multiple packages from your node_modules/
folder at once. Good job! 👍