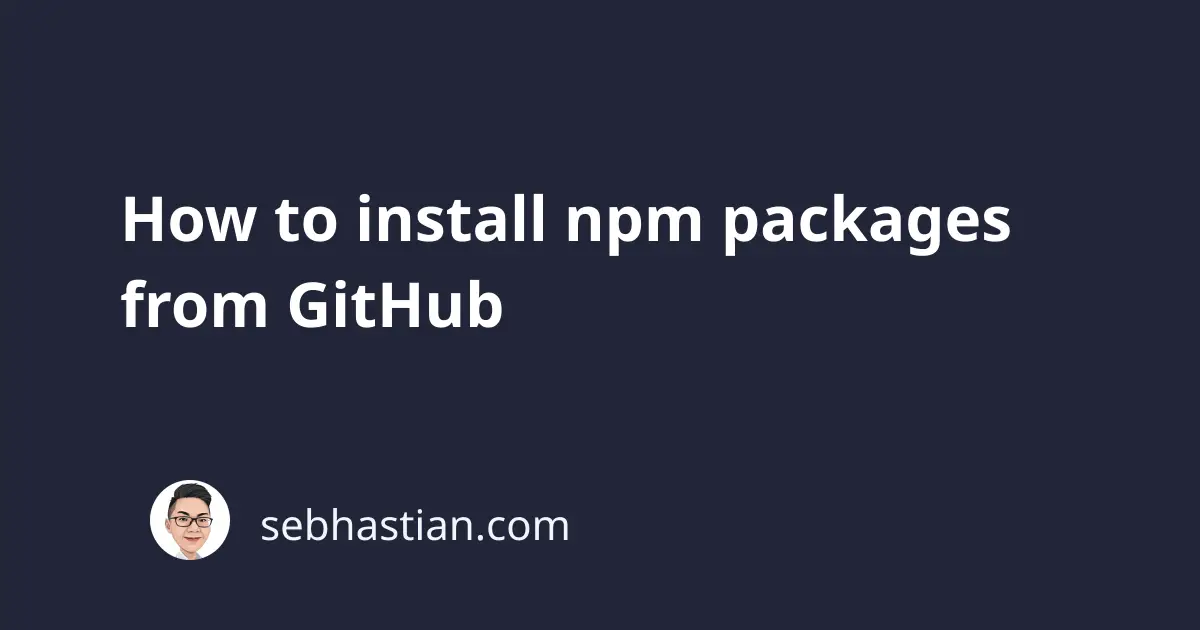
npm packages are usually installed from the npm registry located in registry.npmjs.org
.
You can perform a package installation using the npm install
command like this:
npm install lodash
But the registry is not the only source for installing packages.
According to the npm install
documentation, a package
can be a folder containing a JavaScript project that has a package.json
file as its description.
npm can also install from a Git remote URL, as long as the target has a package.json
file in the root directory.
For example, you can install the same lodash
package from its GitHub homepage as follows:
npm install https://github.com/lodash/lodash
npm will look into the package.json
file located in the root project and install the lodash
package from the GitHub URL above.
You can also choose to install a specific branch by defining the /tree/{branch}
in your URL.
The example below installs express
version 4.18
from its GitHub URL:
npm install https://github.com/expressjs/express/tree/4.18
You can also install a specific commit by replacing the {branch}
with the {commit}
hash like this:
npm install https://github.com/axios/axios/tree/934f390cc3
The packages you installed using GitHub URLs will be listed as dependencies
with the following URI format:
github:{username}/{repo}
#or
github:{username}/{repo}#{branch}
#or
github:{username}/{repo}#{commit}
Here’s an example of the dependencies
list in the package.json
file:
{
"dependencies": {
"axios": "github:axios/axios#934f390cc3",
"express": "github:expressjs/express#4.18",
"lodash": "github:lodash/lodash"
}
}
When you didn’t mention a specific branch or commit, then the installation will proceed using the default branch (generally main
or master
branch)
You can also use the GitHub URI to install packages from GitHub:
npm install github:axios/axios
The URI github:axios/axios
will resolve to https://github.com/axios/axios
URL and install the axios
package for you.
Installing from a GitHub private repository
You can install a package from a GitHub private repository as long as you have access to the repo as an authenticated user.
For example, I can install packages from my private repository as follows:
npm install https://github.com/nsebhastian/private-package
The repository URL above is a private repo, but because I’m an authenticated user, I can access my own repos.
If you have an SSH key set up for your GitHub user or organization, you can also use the SSH URL to install the package:
npm install [email protected]:nsebhastian/private-package.git
And that’s how you install packages from GitHub private repos.
Conclusion
Although you can install packages available from GitHub, installing from the npm registry should always be your first choice.
This is because the npm registry provides you with packages in tarball format, compressing the package size to allow faster download time.
The npm registry also stores all available versions that you can get with the npm view {package} versions
command:
$ npm view axios versions
[
'0.1.0', '0.2.0', '0.2.1', '0.2.2',
'0.3.0', '0.3.1', '0.4.0', '0.4.1',
'0.4.2', '0.5.0', '0.5.1', '0.5.2',
'0.5.3', '0.5.4', '0.6.0', '0.7.0',
'0.8.0', '0.8.1', '0.9.0', '0.9.1',
'0.10.0', '0.11.0', '0.11.1', '0.12.0',
'0.13.0', '0.13.1', '0.14.0', '0.15.0',
'0.15.1', '0.15.2', '0.15.3', '0.16.0',
'0.16.1', '0.16.2', '0.17.0', '0.17.1',
'0.18.0', '0.18.1', '0.19.0-beta.1', '0.19.0',
'0.19.1', '0.19.2', '0.20.0-0', '0.20.0',
'0.21.0', '0.21.1', '0.21.2', '0.21.3',
'0.21.4', '0.22.0', '0.23.0', '0.24.0',
'0.25.0', '0.26.0', '0.26.1', '0.27.0',
'0.27.1', '0.27.2'
]
Instead of rolling back to a specific branch or commit, you can roll back to the previous release versions, which is safer for production builds.
Finally, packages installed from GitHub will be skipped when you run the npm outdated
command, so you can’t check for the latest version.
But if your package is a private package, then installing from Github may be a viable alternative. You need a paid user account to publish private packages to npm registry.
Now you’ve learned how to install npm packages from GitHub URLs. Good work! 👍