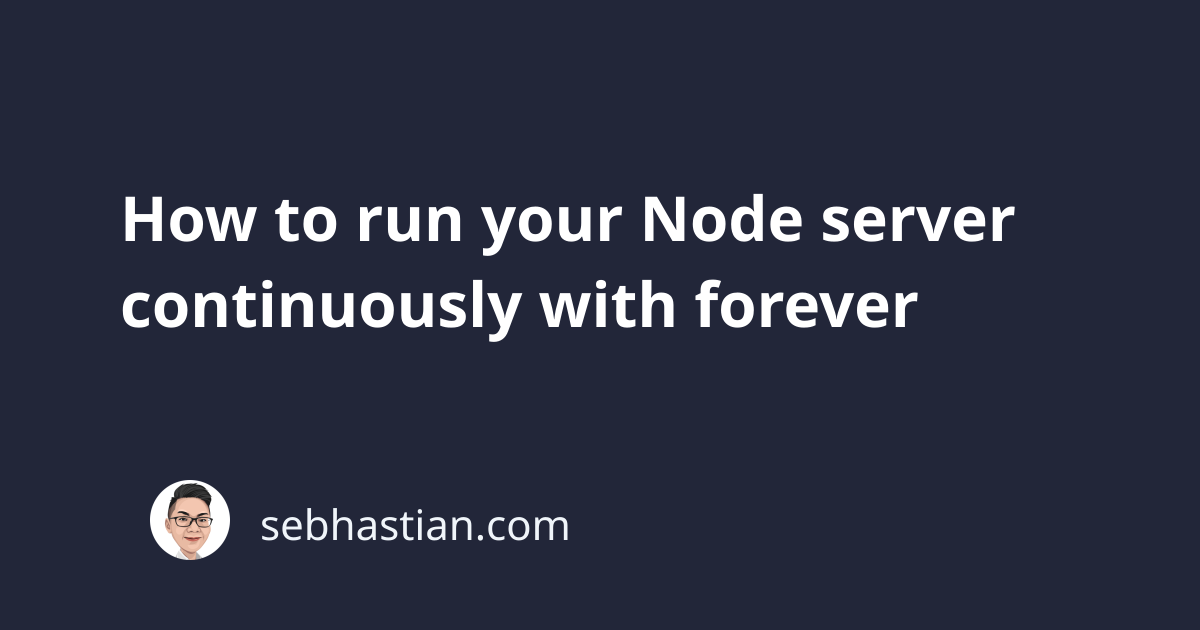
What is npm forever?
Forever is an npm package used to keep your script running continuously in the background.
It’s a handy CLI tool that helps you to manage your application in the development and production stages.
To use forever
, you need to install it globally:
npm install -g forever
To start running a script with forever, use the forever start
command, followed by the script name.
Here’s an example:
# 👇 running a script with forever
forever start index.js
# 👇 running multiple scripts with forever
forever start index.js app.js
You can run as many scripts as you need using forever
.
To check if there’s any running forever process, run the forever list
command.
You will see an example output as follows:
$ forever list
info: Forever processes running
data: uid
data: [0] 4FX5
The forever process will be registered and keep running in the background.
To stop a running forever process, run the forever stop [script name]
command:
# 👇 stop a running forever script
forever stop index.js
When your application throws an error, forever
will automatically restart the node server for you.
This article will show you some practical example of using forever.
Using forever watch mode in development
When developing an application using Node, you can use forever
to monitor and watch your source code.
When there’s a change in your code, forever
will restart your server so you can test the change.
To enable watch mode, add the -w
or --watch
option when running forever start
as follows:
forever start -w server.js
The watch mode is very handy when you are developing your application.
Suppose you have an Express application as shown below:
const express = require("express");
const app = express();
const port = 3000;
app.get("/", (req, res) => {
res.send("Hello World!");
});
app.listen(port, () => {
console.log(`Example app listening on port ${port}`);
});
Then, you change the response sent from the default route from Hello World!
to Bonjour!
as shown below:
res.send("Hello World!");
// 👆 replace with 👇
res.send("Bonjour!");
When you save the changes, forever
will automatically restart the Express server.
If you run the server using the node server.js
command, then you need to stop the running process and execute the command again to see the changes take effect.
And that’s how you use forever
to help your development. There are other options that you can add to the command.
You can see all available options and more information about forever on the forever npm page
Thanks for reading!