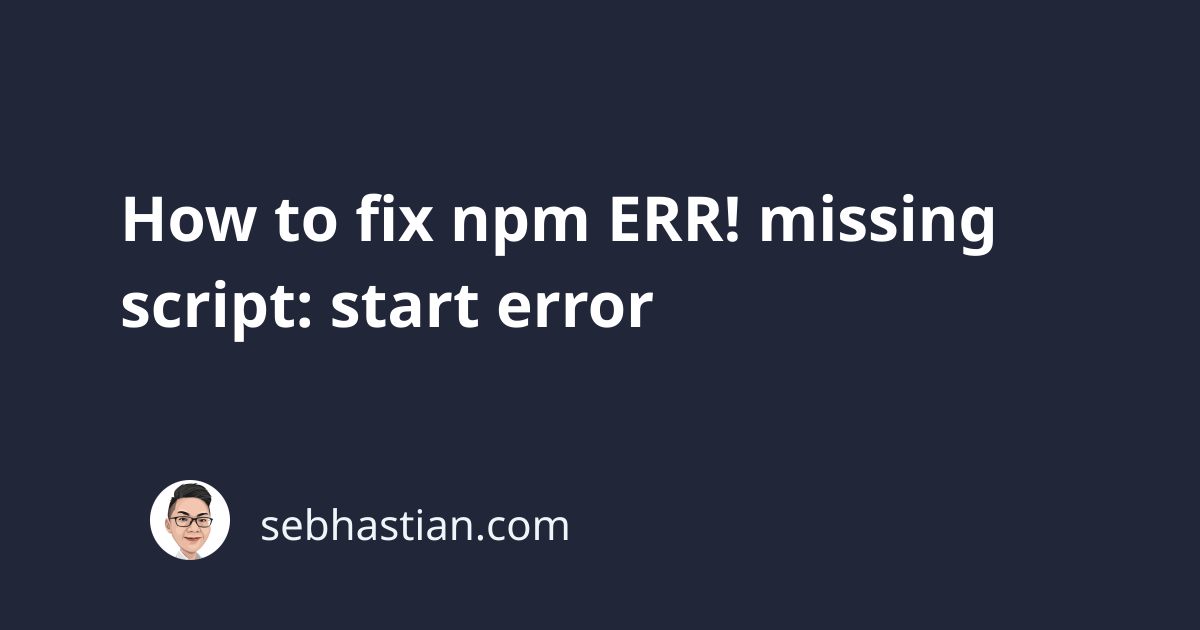
The npm ERR! missing script: start
error occurs when you have not defined the start
script in your package.json
file and you don’t have a server.js
script that Node will execute when running the command.
Here’s an example of the error message in the terminal:
$ npm run start
npm ERR! Missing script: "start"
npm ERR!
npm ERR! Did you mean one of these?
npm ERR! npm star # Mark your favorite packages
npm ERR! npm stars # View packages marked as favorites
npm ERR!
npm ERR! To see a list of scripts, run:
npm ERR! npm run
...
But don’t worry because this error can be fixed easily.
This article will show you five solutions you can try to fix this error:
Add a start script in your package.json file
The package.json
file is an important part of a Node.js application. This file is where all relevant information about the project is stored.
No matter if you have React, Vue, Angular, or any other JavaScript framework, the package.json
file should exist in your project.
It stores all important metadata such as the name
, version
, dependencies
, and scripts
defined for the project.
When you run the npm start
command, npm will look into the scripts
section in your package.json
file for the start
script.
Here’s an example of the start
script:
{
"name": "v-app",
"version": "1.0.0",
"scripts": {
"start": "node index.js"
}
}
The example above defines the start
script to run the code inside index.js
file using the node
command.
If the start
script is not defined, then npm will try to look for a server.js
file in the root directory of your project.
When npm can’t find the start
script and server.js
file, then it will trigger the missing script start error.
To resolve the missing script: “start” npm error, you need to define the start
script in your package.json
file like in the example.
What you write in the start
script depends on the configuration of your project.
If you don’t have a package.json
file, then it’s possible that you have accidentally deleted the file. You need to get the package.json
file back.
Once you added a start
script to the scripts
section as shown above, the npm start
command should work.
Add a server.js file to your project
When you don’t have a package.json
file, npm will try to look for a file named server.js
in your project and run it.
Suppose you have a Node project with the following structure:
.
├── App.css
├── App.js
├── App.test.js
├── index.css
└── index.js
Without defining a start
script, the npm start
command will look for a file named server.js
and run it.
If you want to run the index.js
file, then you need to rename it to server.js
so that it will be run
Run your entry point file directly
The start
script is actually only an alias for the actual command that gets executed by npm.
Usually, the file you designate as the entry point should be already available in your project.
If none of the above solutions worked, then you can try to run the file manually.
Run one of the following commands from your terminal:
node index.js
# or
node server.js
# or
node main.js
Once you successfully run the entry point file, you should edit the package.json
file and put the command you used inside the start
command.
Make sure you have no duplicate scripts section
Sometimes, the error happens because you have duplicate scripts
section in the package.json
file.
There are two scripts
section in the example below:
{
"name": "v-app",
"version": "1.0.0",
"scripts": {
"start": "node index.js"
},
"scripts": {
"dev": "gulp"
}
}
When you have more than one scripts
section, then npm will read only the latest entry at the bottom of the file.
You can check the available scripts using the npm run
command like this:
$ npm run
Scripts available in [email protected] via `npm run-script`:
dev
gulp
The above shows that npm can’t find the start
script.
To resolve this error, you need to merge the two scripts
section as one:
{
"name": "v-app",
"version": "1.0.0",
"scripts": {
"start": "node index.js",
"dev": "gulp"
}
}
Save the file changes, then run the npm run
command again to verify the error has been solved
$ npm run
Lifecycle scripts included in [email protected]:
start
node index.js
available via `npm run-script`:
dev
gulp
As you can see, the terminal output from npm run
is different. The start
script is now available.
Missing start script because of create-react-app
The missing script start error is also known to happen when you create a new React project using create-react-app
as follows:
create-react-app my-app
This is because create-react-app
no longer supports the global install, as stated on its website.
To resolve the error, you need to use the latest version of create-react-app
with npx.
Run the following commands step by step:
# 👇 remove the globally installed package
npm uninstall -g create-react-app
# 👇 clear npx cache to ensure you use the latest cra version
npx clear-npx-cache
# 👇 run create-react-app with npx
npx create-react-app my-app
npx will fetch the latest version of create-react-app
to create the new project.
Once the project is created, you can open the package.json
file and verify that the scripts
section is available inside the package.json
file as follows:
{
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
},
}
Now you should be able to run npm start
from your React application.
Conclusion
Encountering the npm ERR! missing script: start
error when using npm can be frustrating, but it is a common problem that can be easily fixed.
The solutions discussed in this article include adding the start
script to the package.json
file, adding a server.js
file, ensuring the main file is runnable by executing it directly with node
, and removing a duplicate scripts
section in your package.json
file.
You should now be able to fix the error and successfully run your Node project.
Cheers! 🍻