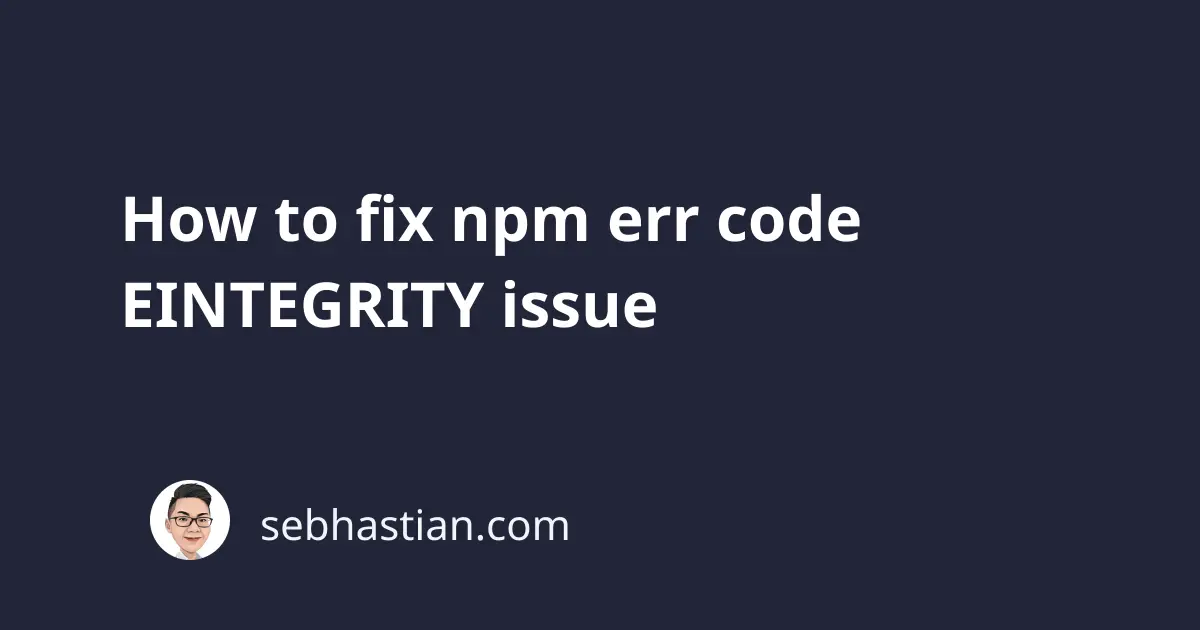
To fix the EINTEGRITY
issue with your npm package, use the following steps:
# 👇 remove node_modules and package-lock.json
rm -rf node_modules package-lock.json
# 👇 Clear and verify npm cache
npm cache clean --force
npm cache verify
# 👇 now run npm install again
npm install
You should be able to run the installation now. Read this article to learn more why you have such error.
npm integrity checksum error explained
When running the npm install
command, you may see the process failed with EINTEGRITY
error code.
Here’s an example output of the error:
npm ERR! code EINTEGRITY
npm ERR! sha512-vjA...Azq==
integrity checksum failed when using sha512:
wanted sha512-vjA...Azq==
but got sha512-MjAA...LNsqvg==. (161379 bytes)
npm ERR! A complete log of this run can be found in:
npm ERR! /Users/nsebhastian/.npm/_logs/2022-06-16T10_04_42_791Z-debug.log
When you run the npm install
command, npm will look for the packages listed as dependencies in your package.json
file to download and install.
When you have a package-lock.json
file in the directory, then npm will compare the checksum of the downloaded tarball .tgz
file with the one found in your lock file.
The EINTEGRITY
error as seen above happens when there is a mismatch of the checksum value between the .tgz
file and the lock file.
In the error message, you can see that npm says wanted sha512… but got sha512… instead.
The value in wanted… is the one found in the package-lock.json
file.
But got… is the value got when npm generates the checksum from the tarball file.
There are two ways you can resolve this error:
- Delete the
package-lock.json
file - Update the integrity value listed in your
package-lock.json
file
To easily fix the error, you can just delete the package-lock.json
file and let npm generate a new one once the installation is finished.
Or if you want to keep the package-lock.json
file, then you need to replace the integrity
value in the right package.
To do so, search and replace the value from the error message in your package-lock.json
file.
In the above case, replace the wanted value sha512-vjA… with sha512-MjAA…
Of course, this will be annoying when you have many EINTEGRITY
errors. It will be easier to just remove the package-lock.json
file since npm will generate a new one anyway.
If the method above doesn’t work, then follow the steps below to clear your node modules and npm cache folders:
# 👇 remove node_modules and package-lock.json
rm -rf node_modules package-lock.json
# 👇 Clear and verify npm cache
npm cache clean --force
npm cache verify
# 👇 now run npm install again
npm install
If you want to learn the detail of this error, then you need to understand what is a checksum value first.
What are checksum values?
A checksum value is an alphanumeric hash value generated from a specific file.
When the content of a file changes, then the checksum value will change as well.
In npm packages, checksums are used to verify the integrity of the files you downloaded.
The first time you install a package as a dependency, npm will generate and store the checksum of each package in the package-lock.json
file.
An example can be seen below:
{
"@babel/code-frame": {
"version": "7.16.7",
"resolved": "https://registry.npmjs.org/@babel/code-frame/-/code-frame-7.16.7.tgz",
"integrity": "sha512-iAXqUn8IIeBTNd72xsFlgaXHkMBMt6y4HJp1tIaK465CWLT/fG1aqB7ykr95gHHmlBdGbFeWWfyB4NJJ0nmeIg==",
"requires": {
"@babel/highlight": "^7.16.7"
}
}
}
The integrity
property in the dependency graph above is generated by running the sha512
hash function against the .tgz
file.
In the case above, the code-frame-7.16.7.tgz
file.
When you run npm install
in the future, npm will run the hash function again for the code-frame-7.16.7.tgz
file and see if the checksum is the same as the one in the integrity
property.
When the value is different, npm will throw the EINTEGRITY
error code.
This is why removing the package-lock.json
file works. npm will skip the checksum comparison process for the package (until the next time you run npm install
again)
Before npm version 5, the checksum is generated with sha1
instead of sha512
.
Sometimes, npm also generates an EINTEGRITY
warning because of different sha hash value as shown below:
npm WARN registry Unexpected warning for https://registry.npmjs.org/:
Miscellaneous Warning EINTEGRITY: sha1-UWbihk...TIIM=
integrity checksum failed when using sha1:
wanted sha1-UWbihk...TIIM=
but got sha512-yJHVQEh...pWft6kWBBcqh0UA==. (11423 bytes)
This means you have sha1 values in your lock file, but npm produces sha512 values.
Again, you need to remove the node_modules/
folder and package-lock.json
file to resolve the warning.
If you’re using an older version of npm, upgrade your npm version before trying the installation again:
# 👇 upgrade npm version
npm install -g npm@latest
# 👇 remove node_modules and package-lock.json
rm -rf node_modules package-lock.json
# 👇 Clear and verify npm cache
npm cache clean --force
npm cache verify
# 👇 now run npm install again
npm install
Run the above commands sequentially to resolve the EINTEGRITY
error.