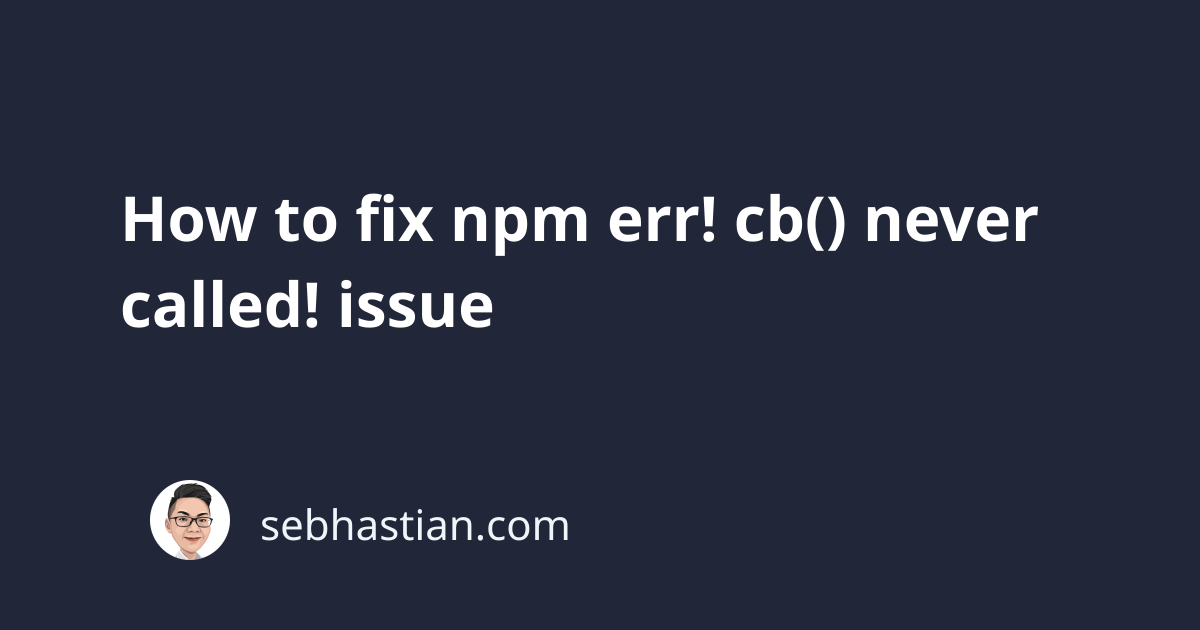
When running the npm install
command, sometimes you will have an npm error that causes the install to fail.
The error says the cb()
function is never called as follows:
npm ERR! cb() never called!
npm ERR! This is an error with npm itself. Please report this error at:
npm ERR! <https://github.com/npm/npm/issues>
npm ERR! A complete log of this run can be found in:
npm ERR! /Users/nsebhastian/.npm/_logs/2022-06-13T07_08_32_041Z-debug-0.log
The cb()
function mentioned above is a vanilla callback function. The function was used by the npm install
command to mark when an install is completed.
The error means that the installation process was interrupted and left unfinished.
The cause of this error varies from outdated npm
package and cache, a limited memory space, and an incompatible node version.
Here are some things you can try to fix the cb()
never called error:
- Check the
npm
version and cache - Delete the
package-lock.json
file - Check the
strict-ssl
config - Check if you have enough memory for the packages
- Define the
node
version inpackage.json
file (for Heroku)
Let’s see how you can fix the error with the steps above.
Check your npm version and cache
An outdated npm
cache may trigger the error, so you can try to fix the error by clearing the cache.
Run the following command from your terminal:
npm cache clean --force
Once the cache is cleared, run the npm install
command again.
If that doesn’t work, then make sure you are using the latest npm
version. At the time of this writing, the latest npm
version is 8.12.1.
Use the following commands to check and install the latest stable npm
version:
# 👇 check npm version
npm -v
# 👇 install the latest npm version
npm install -g npm@latest
# 👇 if blocked, add sudo command
sudo npm install -g npm@latest
Sometimes, a global installation with the -g
flag may get blocked because of a permission issue.
Add sudo
to perform the installation with admin privileges.
After the npm
is updated, run the npm install
command again.
Delete your package-lock.json file
If you still see the error, then you may need to delete the package-lock.json
file generated in your project directory.
The reason is that npm
generates two different lockfile versions depending on its version.
npm v5 and v6 generated lockfile version 1, and v7 onward generated lockfile version 2.
You can open the package-lock.json
file and see the lockfileVersion
property:
{
"name": "r-app",
"version": "0.1.0",
"lockfileVersion": 2,
// ...
}
Delete the package-lock.json
file and try running the npm install
command again. Some people say it fixed the issue.
Check the strict-ssl configuration
If you have firewall protection on your computer, then the error may appear when npm
do SSL key validation for the install process.
To resolve this error, set the strict-ssl
to false:
npm config set strict-ssl=false
And npm won’t do SSL certificate validation when running installation via https.
Check for your memory space
The npm install
command can generate a node_modules/
folder that is more than 1GB in size.
A React application generated using create-react-app
has a node_modules/
folder that’s around 200MB.
Your local or production environment may not have enough space, so the installation process was interrupted.
It’s not possible to determine the space needed for your node_modules/
folder, so you can only get an estimation.
Try to get at least a few gigabytes of empty space in your computer before running the installation again.
Define the node engines config in package.json
When you see the error on cloud platforms like Heroku and AWS, then it’s possible that the Node.js version used to run the installation is incompatible with your dependencies.
You can specify the node
version required by your application in the package.json
file.
Consider the example below:
{
"name": "example-app",
"description": "My application",
"version": "1.0.0",
"engines": {
"node": "16.x"
}
}
Without specifying the version, cloud platforms will use their own default version. As of this writing, Heroku uses Node v16. Not sure about AWS.
Run the node -v
command to find the node version installed on your computer, then specify the same version in the package.json
file as shown above.
Finally, commit and push the update. See if the issue is resolved.
Conclusion
The above methods are the most relevant fixes for cb()
never called issue.
Sometimes, the error also happens because of a connection timeout, so you can check if you have internet connectivity issues before running the install command again.
Make sure that you can access https://registry.npmjs.org/ from your browser.
If you need more help, you can open a new question on Stack Overflow. Add the detail of your error and mention that you have tried the fixes in this article.
Thanks for reading! 🙏