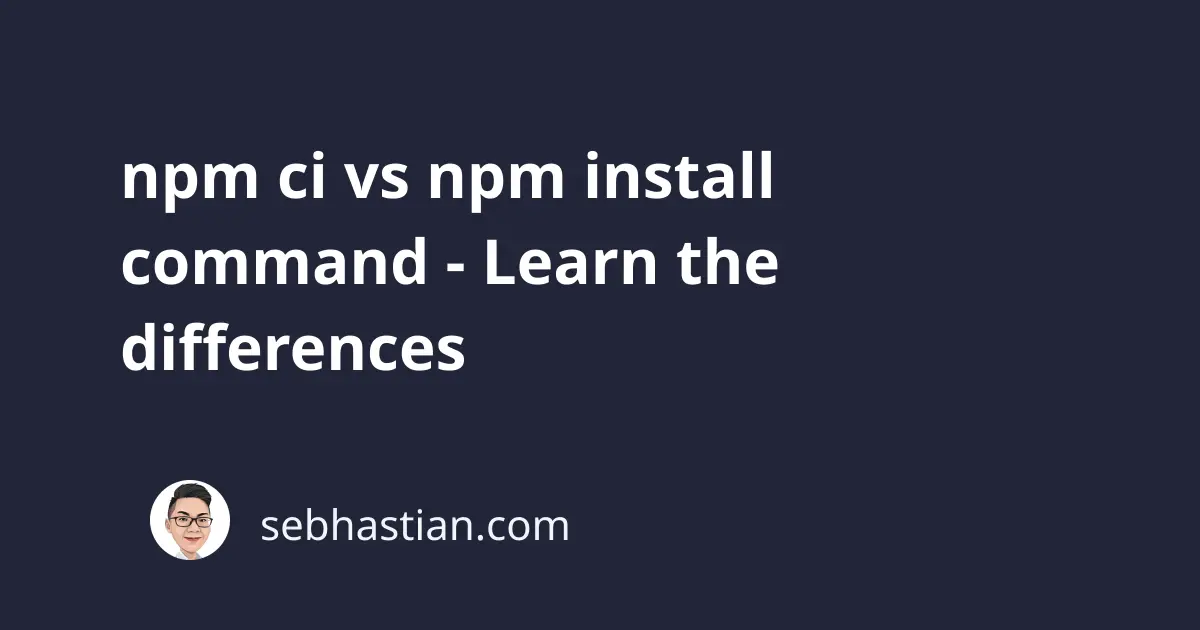
The npm install
and npm ci
commands are both used to install packages needed by your application.
But these two commands run the installation process with some differences.
Here are the differences between npm install
and npm ci
:
The npm install command
The npm install
command is the default command used to install dependencies for your project.
Features of npm install
include:
- You can install new dependencies not listed in your
package.json
file - Controls the
package.json
file by adding and removing packages from the dependencies list - Generates a
package-lock.json
ornpm-shrinkwrap.json
lock file
With npm install
, you can install packages not yet included in your package.json
file.
Suppose you have a package.json
file with the following content:
{
"name": "n-app",
"version": "1.0.0",
"main": "index.js",
"dependencies": {
"axios": "^0.27.2",
},
}
Then, you install webpack as a dependency to your project as follows:
npm install webpack
Once the package is installed successfully, then the dependencies list will be updated:
{
"name": "n-app",
"version": "1.0.0",
"main": "index.js",
"dependencies": {
"axios": "^0.27.2",
"webpack": "^5.73.0"
},
}
Furthermore, npm install
also updates any dependency versions when available.
In the example above, you see the package.json
listed webpack as a dependency version ^5.73.0.
The caret symbol (^) besides the version number means that when installing the package, npm will look for the latest minor version available from the registry and install it for you.
When you install webpack, the latest version is 5.73.0. But in the future, webpack may release a new version (5.75.0 for example) to introduce minor updates.
Instead of installing 5.73.0, npm will install 5.75.0 for you.
npm will also update the package-lock.json
file accordingly, so that the next time you run npm install
, npm knows that the latest version installed is 5.75.0.
The package-lock.json
is a json file that keeps track of the exact dependency versions installed in your project.
The npm ci command
The npm ci
command is short for clean-install. It was designed to install package dependencies in automated environments, such as when you do continuous integration for your project.
The npm ci
command is available in npm v6 and above:
- You can’t install individual packages like with
npm install
npm ci
never modifies yourpackage.json
andpackage-lock.json
files- The command requires
package-lock.json
ornpm-shrinkwrap.json
to be available. It will not run when a package lock file is not present
npm ci
also deletes your node_modules
folder if it’s present to ensure a clean install.
You can’t install individual dependencies as you can with npm install
:
# 👇 error
npm ci webpack
When the versions listed under package.json
and package-lock.json
files do not match, npm ci
will throw an error and stop the process.
This is different from npm install
which will update package-lock.json
to match package.json
versions.
Because npm ci
doesn’t check for the latest versions available, the command can run faster than npm install
. In a project with many dependencies, npm ci
can be twice faster than npm install
.
When to use npm install vs npm ci?
The npm install
command is used when you are developing the project. This is where you install new dependencies and update existing ones.
When you finished a project and want to run it in automated environments, the npm ci
command should be used.
This ensures that your project will have the exact dependencies versions installed in testing and production environments.
To summarize: use npm install
for development. Use npm ci
when you run the project in automated environments.
Unlike npm install
that seeks to update existing dependencies when possible, npm ci
only install existing dependencies. This ensures you have reliable builds in continuous integration.
And now you’ve learned the differences between npm install
and npm ci
commands. Great work! 👍