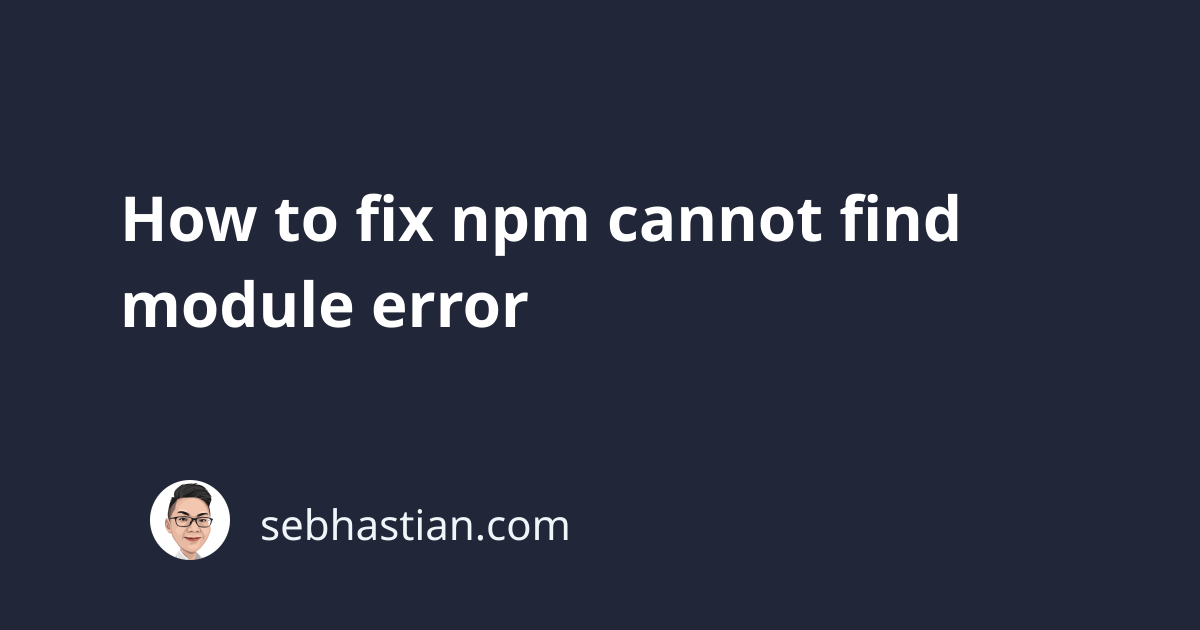
JavaScript’s Node.js server supports module export and import in both ECMAScript modules and CommonJS format.
Sometimes, npm will throw an error saying Cannot find module
because of module import as shown below:
$ node index.js
node:internal/modules/cjs/loader:936
throw err;
^
Error: Cannot find module 'axios'
Require stack:
- /n-app/index.js
at ... {
code: 'MODULE_NOT_FOUND',
requireStack: [ '/n-app/index.js' ]
}
Here’s the content of the index.js
file:
var axios = require("axios");
The cannot find module error occurs because npm cannot find the module required by the index.js
file. In this case, the axios
module.
To resolve the error, you need to make sure that axios
is installed in the node_modules/
folder.
npm install axios
Please note that the node_modules/
folder must be located in the same directory as the index.js
file:
.
├── index.js
├── node_modules
├── package-lock.json
└── package.json
If you have run the npm install
command before, then it’s possible that the installation of the module is incomplete or corrupted.
Delete the node_modules/
folder using the rm -rf node_modules
command, then run npm install
again. That may fix the issue.
Finally, the same error can happen when you require()
a local .js
file that can’t be found.
Suppose you have a file named lib.js
placed in the same folder as the index.js
file:
.
├── index.js
└── lib.js
To import the file, you need to specify the correct path in the require()
function.
The following code:
var helper = require("lib.js");
Will produce the same error:
$ node index.js
node:internal/modules/cjs/loader:936
throw err;
^
Error: Cannot find module 'lib.js'
Require stack:
- /n-app/index.js
at ... {
code: 'MODULE_NOT_FOUND',
requireStack: [ '/n-app/index.js' ]
}
This is because the require()
function will always look inside the node_modules/
folder.
To let Node.js knows that you are importing a local file, use the absolute path as follows:
./lib.js
The ./
syntax means the root directory where you run the node
command. In this case, the folder where index.js
is located.
If you have a file one level down like this:
.
├── index.js
└── helpers
└── lib.js
Then you need to adjust the require()
path as shown below:
var helper = require("./helpers/lib.js");
The same also applies when you use the ES modules format as follows:
import helper from "./helpers/lib.mjs";
To conclude, the error “Cannot find module” happens when Node.js can’t find the module that a file is trying to import.
You can see the file and the module that’s causing the issue from the error output generated by Node itself.
And that’s how you resolve the npm cannot find module issue. Great work! 😉