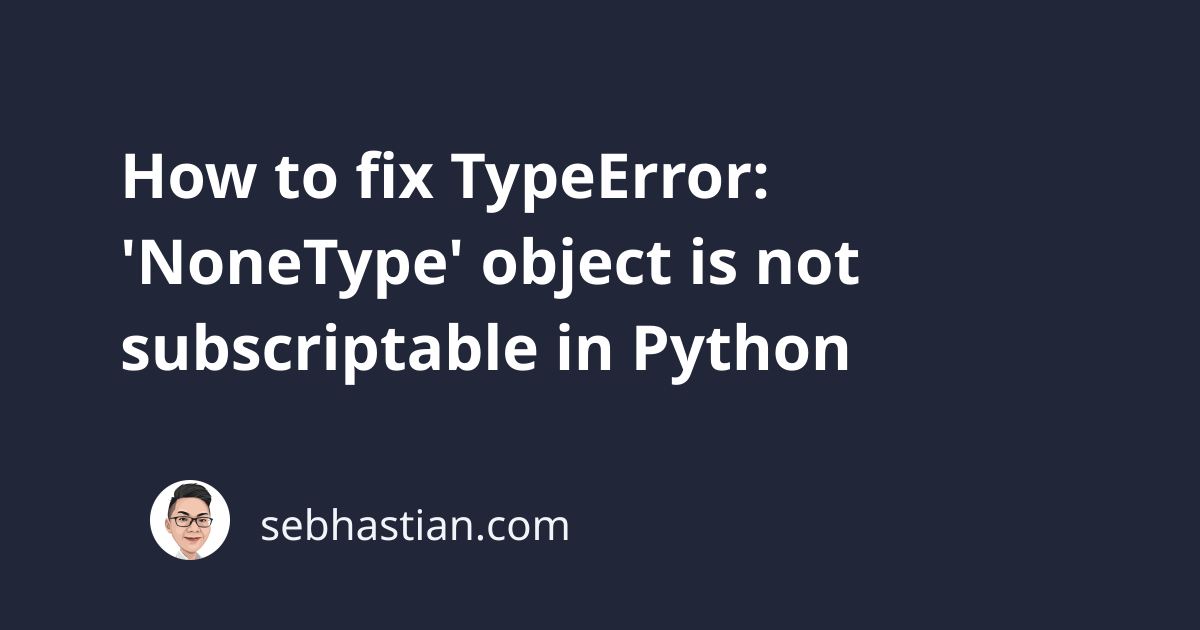
One error you might encounter when running Python code is:
TypeError: 'NoneType' object is not subscriptable
This error occurs when you attempt to access an element of a None
object as if it were a subscriptable object (like a string, list, or set) using the square brackets []
notation.
Most likely, you’ve mistaken a None
object for an iterable because you assign the result of calling iterable methods such as the list.sort()
method.
This tutorial will show you an example that causes this error and how I fix it in practice.
How to reproduce this error
Suppose you have a list of strings in your code, which you sorted using the list.sort()
method:
my_list = ["z", "b", "k"]
sorted_list = my_list.sort()
Next, you want to access an element of the sorted_list
as follows:
print(sorted_list[1])
But you get this error:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(sorted_list[1])
~~~~~~~~~~~^^^
TypeError: 'NoneType' object is not subscriptable
This error occurs because the list.sort()
method in Python sorts the list in place, meaning the original list is changed without returning a new sorted list.
You can test this by printing the my_list
and sorted_list
variables:
my_list = ["z", "b", "k"]
sorted_list = my_list.sort()
print(my_list)
print(sorted_list)
Output:
['b', 'k', 'z']
None
As you can see, the my_list
variable is already ordered, while the sorted_list
variable contains a None
object.
How to fix this error
To resolve this error, you need to access the sorted list items directly in the original list.
You don’t need to assign a new variable when calling the sort()
method because the method doesn’t return any value:
my_list = ["z", "b", "k"]
my_list.sort()
print(my_list[1]) # k
When in doubt, you need to check on the variable you accessed using the subscript (square brackets) notation.
You might expect the variable to be a different type, but you got a None
instead. Trace the code and see where the variable is assigned a None
in your source code.
Conclusion
The Python TypeError: 'NoneType' object is not subscriptable
occurs when you attempt to access a None
object using the square brackets []
notation.
The square brackets are reserved for subscriptable objects such as a string, a list, or a tuple, so using it on a None
object will raise an error.
To resolve this error, you need to make sure you’re not accessing a None
object using square brackets.
I hope this tutorial is useful. See you in other tutorials! 👋