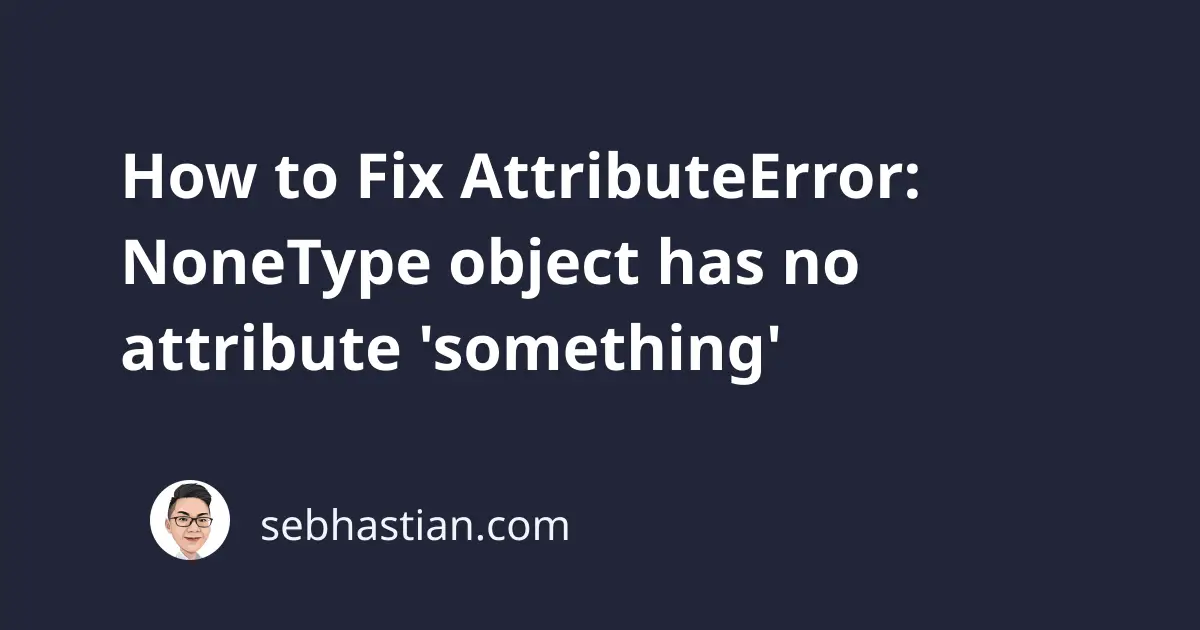
When running Python code, sometimes you get an error that says:
AttributeError: NoneType object has no attribute 'something'
The ‘something’ attribute here can be any function or attribute that you’re trying to access from the object.
This error usually occurs when you get a None
object instead of any other object you think you’re getting.
For example, suppose I try to call the join()
method thinking I have a str
object:
my_str = None
my_str.join(", ")
The output will be:
Traceback (most recent call last):
File "main.py", line 3, in <module>
my_str.join(", ")
AttributeError: 'NoneType' object has no attribute 'join'
Here, the error says ‘NoneType’ object has no attribute ‘join’ because the None
object in Python doesn’t have the join()
attribute.
The same scenario applies no matter what attribute you’re trying to access or call.
To resolve this error, you need to avoid accessing the attribute that’s not defined in the object.
One way to do so is to use a try-except
block to handle the error gracefully when the object is actually None
:
my_str = None
try:
my_str.join(", ")
except AttributeError:
print("The attribute doesn't exist")
# ... continue code execution
By using a try-except
block, the error won’t stop the code execution.
Another way to avoid this error is to make sure that you’re not assigning None
to the variable in the lines between the declaration of that variable and the line where you call the attribute.
In the following example, the variable my_str
got assigned a None
object in the middle:
my_str = "abcdef"
my_str = None
try:
my_str.join(", ")
except AttributeError:
print("The attribute doesn't exist")
Here, you can see the my_str
variable is assigned a None
right before the try
block.
You need to avoid doing so to resolve this error.
Conclusion
The AttributeError: NoneType object has no attribute 'something'
occurs in Python whenever you attempt to access an attribute or call a function that’s not defined in the None
object.
Most likely, you somehow got the original object replaced by None
, or you got one from an API or function call.
To resolve this error, you can use a try-except
block to catch when you accessed an attribute from a None
object.
If you have access to the original variable source, you can also make sure that you didn’t assign a None
to the variable by accident.
I hope this tutorial helps. Happy coding!